Question
A count-controlled loop iterates a specific number of times. Although you can write this with a While or a Do-While loop as performed in Lab
A count-controlled loop iterates a specific number of times. Although you can write this with a While or a Do-While loop as performed in Lab 5, most programming languages provide a loop known as the For loop. This loop is specifically designed as a count-controlled loop.
The process of the For loop is:
The loop keeps a count of the number of times that it iterates, and when the count reaches a specified amount, the loop stops.
A count-controlled loop uses a variable known as a counter variable to store the number of iterations that it has performed.
Using the counter, the following three actions take place (Initialization, Test, and Increment).
The pseudocode for a for statement looks as follows:
For counterVariable = startingValue to maxValue
Statement
Statement
Statement
Etc.
End For
This lab requires you to implement a count-controlled loop using a For statement.
Step 1: Examine the following code.
Constant Integer MAX_HOURS = 24
Declare Integer hours
For hours = 1 to MAX_HOURS
Display "The hour is ", hours
End For
Step 2: Explain what you think will be displayed to the screen in Step 1. (Reference: For Statement, page 239):
______________________________________________________________
______________________________________________________________
______________________________________________________________
Step 3: Write a For loop that will print 60 minutes to the screen. Complete the missing lines of code.
Constant Integer MAX_MINUTES = ______________________
Declare Integer minutes
For _____________ = 1 to ___________________
Display ________________________________________
End For
Step 4: Write a For loop that will print 60 seconds to the screen. Complete the missing lines of code.
Constant Integer MAX_SECONDS = ______________________
Declare Integer seconds
For _____________ = 1 to ___________________
Display ________________________________________
End For
Step 5: For loops can also be used to increment by more than one. Examine the following code.
Constant Integer MAX_VALUE = 10
Declare Integer counter
For counter = 0 to MAX_VALUE Step 2
Display "The number is ", counter
End For
Step 6: Explain what you think will be displayed to the screen in Step 5. (Reference: Incrementing by Values Other than 1, page 244):
______________________________________________________________
______________________________________________________________
______________________________________________________________
Step 7: Write a For loop that will display the numbers starting at 20, then 40, then 60, and continuing the sequence all the way to 200.
Constant Integer MAX_VALUE = _________________
Declare Integer counter
For counter = ____________ to MAX_VALUE Step ____________
Display "The number is ", _____________________
End For
Step 8: For loops can also be used when the user controls the number of iterations. Examine the following code:
Declare Integer numStudents
Declare Integer counter
Display "Enter the number of students in class"
Input numStudents
For counter = 1 to numStudents
Display "Student #", counter
End For
Step 9: Explain what you think will be displayed to the screen in Step 8. (Reference: Letting the User Control the Number of Iterations, page 248):
______________________________________________________________
______________________________________________________________
______________________________________________________________
Step 10: For loops are also commonly used to calculate a running total. Examine the following code.
Declare Integer counter
Declare Integer total = 0
Declare Integer number
For counter = 1 to 5
Display "Enter a number: "
Input number
Set total = total + number
End For
Display "The total is: ", total
Step 11: Explain what you think will be displayed to the screen in Step 10. (Reference: Calculating a Running Total, page 255):
______________________________________________________________
______________________________________________________________
______________________________________________________________
Step 12: Write the missing lines for a program that will allow the user to enter how many ages they want to enter and then find the average.
Declare Integer counter
Declare Integer totalAge = 0
Declare Real averageAge = 0
Declare Integer age
Declare Integer number
Display "How many ages do you want to enter: "
Input ___________________________
For counter = 1 to number
Display "Enter age: "
Input ___________________
Set totalAge = _________________ + ________________
End For
averageAge = ___________________ / ___________________
Display "The average age is ", _______________________
Step by Step Solution
There are 3 Steps involved in it
Step: 1
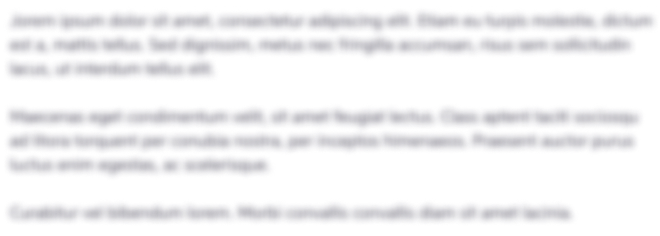
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started