Question
a. I need to recreate the queue push and pop functions which i have already created, but for a stacked element. I have to add
a. I need to recreate the queue push and pop functions which i have already created, but for a stacked element. I have to add another variable using lastOperationIsPush the queue should now be able to hold up tocapacity elements. The complexity of each of your functions should beO(1).
b. A linear list is being maintained circularly in an array withfront andrear, set up as for circular queues. Obtain a formula in terms of the array capacity,front andrear,for the number of elements in the list.
c. Finally I need to create some sort of function to delete thekth element in the list.The kth element of the list is the position (front+k)% capacity. Since the kth element is deleted, all the elements residing in positions from (front+k+1)%capacity to rear must bemoved up by one position.
import java.util.*; public class LLStack<T> { class Node { public T data; public Node next; } private Node first; public LLStack() { first = null; } public void push(T x) { Node n = new Node(); n.data = x; n.next = first; first = n; } public T peek() { if (first == null) { throw new NoSuchElementException(); } else { T ret = first.data; return ret; } } public T pop() { if (first == null) { throw new NoSuchElementException(); } else { Node temp = first; first = first.next; return temp.data; } } public boolean isEmpty() { return first==null; } }
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * * @author scobo */ import java.util.*; // Class for queue public class Queue { private int arr[]; // array to store queue elements private int front; // front points to front element in the queue private int rear; // rear points to last element in the queue private int capacity; // maximum capacity of the queue private int count; // current size of the queue // Constructor to initialize queue Queue(int size) { arr = new int[size]; capacity = size; front = 0; rear = -1; count = 0; } // Utility function to remove front element from the queue public void dequeue() { // check for queue underflow if (isEmpty()) { System.out.println("UnderFlow Program Terminated"); System.exit(1); } System.out.println("Removing " + arr[front]); front = (front + 1) % capacity; count--; } // Utility function to add an item to the queue public void enqueue(int item) { // check for queue overflow if (isFull()) { System.out.println("OverFlow Program Terminated"); System.exit(1); } System.out.println("Inserting " + item); rear = (rear + 1) % capacity; arr[rear] = item; count++; } // Utility function to return front element in the queue public int peek() { if (isEmpty()) { System.out.println("UnderFlow Program Terminated"); System.exit(1); } return arr[front]; } // Utility function to return the size of the queue public int size() { return count; } // Utility function to check if the queue is empty or not public Boolean isEmpty() { return (size() == 0); } // Utility function to check if the queue is empty or not public Boolean isFull() { return (size() == capacity); } /* public static void main (String[] args) { // create a queue of capacity 5 Queue q = new Queue(5); q.enqueue(1); q.enqueue(2); q.enqueue(3); System.out.println("Front element is: " + q.peek()); q.dequeue(); System.out.println("Front element is: " + q.peek()); System.out.println("Queue size is " + q.size()); q.dequeue(); q.dequeue(); if (q.isEmpty()) System.out.println("Queue Is Empty"); else System.out.println("Queue Is Not Empty"); } */ }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
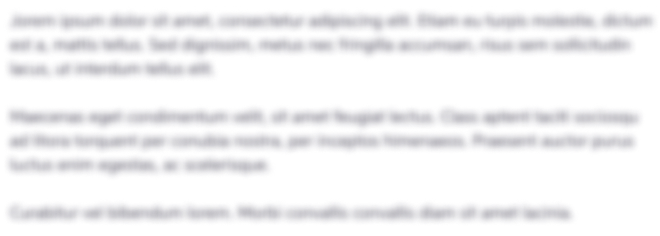
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started