Answered step by step
Verified Expert Solution
Question
1 Approved Answer
/** A message left by the caller */ public class Message { /** Construct a Message object @param messageText the message text */ public Message(String
/** A message left by the caller */ public class Message { /** Construct a Message object @param messageText the message text */ public Message(String messageText) { text = messageText; } /** Get the message text. @return message text */ public String getText() { return text; } private String text; } import java.util.ArrayList; /** A first-in, first-out collection of messages. This implementation is not very efficient. We will consider a more efficient implementation in chapter 3. */ public class MessageQueue { /** Constructs an empty message queue. */ public MessageQueue() { queue = new ArrayList<>(); } /** Remove message at head. @return message that has been removed from the queue */ public Message remove() { return queue.remove(0); } /** Append message at tail. @param newMessage the message to be appended */ public void add(Message newMessage) { queue.add(newMessage); } /** Get the total number of messages in the queue. @return the total number of messages in the queue */ public int size() { return queue.size(); } /** Get message at head. @return message that is at the head of the queue, or null 48 if the queue is empty */ public Message peek() { if (queue.size() == 0) return null; else return queue.get(0); } private ArrayListqueue; } public class Mailbox { /** Creates Mailbox object. @param aPasscode passcode number @param aGreeting greeting string */ public Mailbox(String aPasscode, String aGreeting) { passcode = aPasscode; greeting = aGreeting; newMessages = new MessageQueue(); keptMessages = new MessageQueue(); } /** Check if the passcode is correct. @param aPasscode a passcode to check @return true if the supplied passcode matches the mailbox passcode */ public boolean checkPasscode(String aPasscode) { return aPasscode.equals(passcode); } /** Add a message to the mailbox. @param aMessage the message to be added */ public void addMessage(Message aMessage) { newMessages.add(aMessage); } /** Get the current message. @return the current message */ public Message getCurrentMessage() { if (newMessages.size() > 0) return newMessages.peek(); else if (keptMessages.size() > 0) return keptMessages.peek(); else return null; } /** Remove the current message from the mailbox. @return the message that has just been removed 55 */ public Message removeCurrentMessage() { if (newMessages.size() > 0) return newMessages.remove(); else if (keptMessages.size() > 0) return keptMessages.remove(); else return null; } /** Save the current message */ public void saveCurrentMessage() { Message m = removeCurrentMessage(); if (m != null) keptMessages.add(m); } /** Change mailbox's greeting. @param newGreeting the new greeting string */ public void setGreeting(String newGreeting) { greeting = newGreeting; } /** Change mailbox's passcode. @param newPasscode the new passcode */ public void setPasscode(String newPasscode) { passcode = newPasscode; } /** Get the mailbox's greeting. @return the greeting */ public String getGreeting() { return greeting; } private MessageQueue newMessages; private MessageQueue keptMessages; private String greeting; private String passcode; } public class Connection { /** Construct a Connection object. @param s a MailSystem object @param p a Telephone object */ public Connection(MailSystem s, Telephone p) { system = s; phone = p; resetConnection(); } /** Respond to the user's pressing a key on the phone touchpad @param key the phone key pressed by the user */ public void dial(String key) { if (state == CONNECTED) connect(key); else if (state == RECORDING) login(key); else if (state == CHANGE_PASSCODE) changePasscode(key); else if (state == CHANGE_GREETING) changeGreeting(key); else if (state == MAILBOX_MENU) mailboxMenu(key); else if (state == MESSAGE_MENU) messageMenu(key); } /** Record voice. @param voice voice spoken by the user */ public void record(String voice) { if (state == RECORDING || state == CHANGE_GREETING) currentRecording += voice; } /** The user hangs up the phone. */ public void hangup() { if (state == RECORDING) currentMailbox.addMessage(new Message(currentRecording)); resetConnection(); } /** Reset the connection to the initial state and prompt */ private void resetConnection() { currentRecording = ""; accumulatedKeys = ""; state= CONNECTED; phone.speak(INITIAL_PROMPT); } /** Try to connect the user with the specified mailbox. @param key the phone key pressed by the user */ private void connect(String key) { if (key.equals("#")) { currentMailbox = system.findMailbox(accumulatedKeys); if (currentMailbox != null) { state = RECORDING; phone.speak(currentMailbox.getGreeting()); } else phone.speak("Incorrect mailbox number. Try again!"); accumulatedKeys = "";; } else accumulatedKeys += key; } /** Try to log in the user. @param key the phone key pressed by the user */ private void login(String key) { if (key.equals("#")) { if (currentMailbox.checkPasscode(accumulatedKeys)) { state = MAILBOX_MENU; phone.speak(MAILBOX_MENU_TEXT); } else phone.speak("Incorrect passcode. Try again!"); accumulatedKeys = "";; } else accumulatedKeys += key; } /** Change passcode. @param key the phone key pressed by the user */ private void changePasscode(String key) { if (key.equals("#")) { currentMailbox.setPasscode(accumulatedKeys); state = MAILBOX_MENU; phone.speak(MAILBOX_MENU_TEXT); accumulatedKeys = "";; } else accumulatedKeys += key; } /** Change greeting. @param key the phone key pressed by the user */ private void changeGreeting(String key) { if (key.equals("#")) { currentMailbox.setPasscode(accumulatedKeys); currentRecording = ""; state = MAILBOX_MENU; phone.speak(MAILBOX_MENU_TEXT); } } /** * Respond to the user's selection from mailbox menu. * @param key the phone key pressed by the user */ private void mailboxMenu(String key) { if (key.equals("1")); { state = MESSAGE_MENU; phone.speak(MESSAGE_MENU_TEXT); } else if (key.equals("2")); { state = CHANGE.PASSCODE; phone.speak("Enter new passcode folllowed by the # key"); } } /** * Respond to the user's selection from message menu. * @param key the phone key pressed by the user */ private void messageMenu(String key) { if (key.equals("1")) { String output = ""; Message m = currentMailbox.getCurrentMessage(); if (m == null) output += "No messages." + " "; else output += m.getText() + " "; output += MESSAGE_MENU_TEXT; phone.speak(output); } else if (key.equals("2")) { currentMailbox.saveCurrentMessage(); phone.speak(MESSAGE_MENU_TEXT); } else if (key.equals("3")) { currentMailbox.removeCurrentMessage(); phone.speak(MESSAGE_MENU_TEXT); } else if (key.equals("4")) { state = MAILBOX_MENU; phone.speak(MAILBOX_MENU_TEXT); } } private MailSystem system; private Mailbox currentMailbox; private String currentRecording; private String accumulatedKeys; private Telephone phone; private int state; private static final int DISCONNECTED = 0; private static final int CONNECTED = 1; private static final int RECORDING = 2; private static final int MAILBOX_MENU = 3; private static final int MESSAGE_MENU = 4; private static final int CHANGE_PASSCODE = 5; private static final int CHANGE_GREETING = 6; private static final String INITIAL_PROMPT = "Enter mailbox number followed by #"; private static final String MAILBOX_MENU_TEXT = "Enter 1 to listen to your messages " + "Enter 2 to change your passcode " + "Enter 3 to change your greeting"; private static final String MESSAGE_MENU_TEXT = "Enter 1 to listen to the current message " + "Enter 2 to save the current message " + "Enter 3 to delete the current message " + "Enter 4 to return to the main menu"; } import java.util.ArrayList; /** A system of voice mail boxes */ public class MailSystem { /** * Constructs a mail system with a given number of mailboxes * @param mailboxCount the number of mailboxes */ public MailSystem(int mailboxCount) { mailboxes = new ArrayList<>(); //Initialize mail boxes. for (int i = 0; i < mailboxCount; i++) { String passcode = "" + (i + 1); String greeting = "You have reached mailbox " + (i + 1) + ". Please leave a message now."; mailboxes.add(new Mailbox(passcode, greeting)); } } /** * Locate a mailbox * @param ext the extension number * @return the mailbox or null if not found */ public Mailbox findMailbox(String ext) { int i = Integer.parseInt(ext); if (1 <= i && i <= mailboxes.size()) return mailboxes.get(i-1); else return null; } private ArrayList mailboxes; } import java.util.Scanner; /** A telephone that takes simulated keystrokes and voice input from the user and simulates spoken text */ public class Telephone { /** * Construct phone object. * @param aScanner that reads text form a character-input stream */ public Telephone(Scanner aScanner) { scanner = aScanner; } /** * Speak a message to System.out * @param output the text that will be "spoken" */ public void speak(String output) { System.out.println(output); } /** * Loops reading user input and passes the input to the * Connection object's methods dial, record or hangup. * @param c the connection that connects this phone to the * voice mail system */ public void run(Connection c) { boolean more = true; while (more) { String input = scanner.nextLine(); if (input == null) return; if (input.equalsIgnoreCase("H")) c.hangup(); else if (input.equalsIgnoreCase("Q")) more = false; else if (input.length() == 1 && "1234567890#".contains(input)) c.dial(input); else c.record(input); } } private Scanner scanner; } import java.util.Scanner; /** * This program tests the mail system. A single phone * communicates with the program through System.in/System.out. */ public class MailSystemTester { public static void main(String[] args) { MailSystem system = new MailSystem(MAILBOX_COUNT); Scanner console = new Scanner(System.in); Telephone p = new Telephone(console); Connection c = new Connection(system, p); p.run(c); } private static final int MAILBOX_COUNT = 20;
How would I make this follow the laws of demeter in Java?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
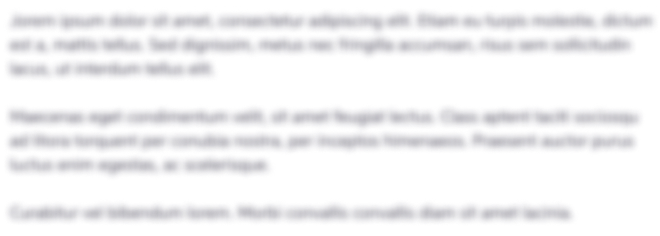
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started