Question
a multi-threaded producer / consumer program without any locking or signaling. It therefore has synchronization problems. Add locks and signals so that it works correctly.
a multi-threaded producer / consumer program without any locking or signaling. It therefore has synchronization problems. Add locks and signals so that it works correctly. /* Homework 5.X */ /* Robin Ehrlich */
/* compile: gcc Homework5.c -lpthread */
#include
struct class { struct class *next; int id; int grade; };
#define SLEEP_TIME 1 #define MAX_PRODUCE 10
static struct class *classHead = NULL; static struct class *classTail = NULL; static pthread_mutex_t classMutex; static pthread_cond_t classSignal; static int producerComplete = 0; static int randomSeed;
static void *consumerThread(void *arg) {
struct class *classCurrent; int sleepTime;
printf("Consumer thread starting ");
while (1) {
/* get next item */ classCurrent = classHead; if ((classCurrent == NULL) && (producerComplete)) break; classHead = classCurrent->next;
/* do some work */ printf("consume: id = %d, grade = %d ", classCurrent->id, classCurrent->grade); sleepTime = (rand_r(&randomSeed) % SLEEP_TIME) + 1; sleep(sleepTime); free(classCurrent); }
printf("Consumer thread completed ");
return (0);
}
static void *producerThread(void *arg) { struct class *classCurrent; int i; int sleepTime;
printf("Producer thread starting ");
for (i = 0; i < MAX_PRODUCE; i++) {
/* do some work */ printf("produce: id = %d, grade = %d ", classCurrent->id, classCurrent->grade); sleepTime = (rand_r(&randomSeed) % SLEEP_TIME) + 1; sleep(sleepTime);
/* give to consumer */
classCurrent = malloc(sizeof(struct class)); classCurrent->id = 462 + i; classCurrent->grade = 90 - i; classCurrent->next = NULL; if (classHead == NULL) classHead = classCurrent; else classTail->next = classCurrent; classTail = classCurrent; }
producerComplete = 1;
printf("Producer thread completed ");
return (0);
}
int main(int argc, char **argv) {
pthread_attr_t attr; struct class *classCurrent; pthread_t consumerTid; pthread_t producerTid;
printf("Starting ");
randomSeed = (int) time(NULL); srand(randomSeed);
pthread_mutex_init(&classMutex, NULL); pthread_cond_init(&classSignal, NULL);
pthread_attr_init(&attr); pthread_create(&producerTid, &attr, producerThread, NULL); pthread_create(&consumerTid, &attr, consumerThread, NULL);
/* wait for threads to complete */
pthread_join(producerTid, NULL); pthread_join(consumerTid, NULL);
printf("Completed ");
return (0);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
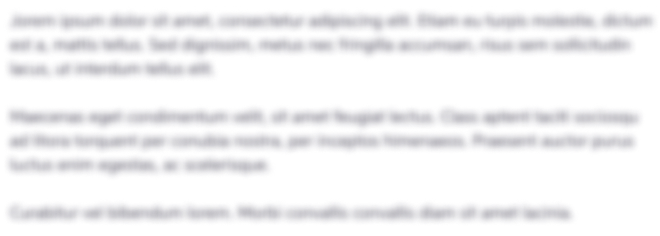
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started