Question
A set is an unordered collection of well-defined and distinct items; that is, there are no duplicate values within a set. In this assignment, your
A set is an unordered collection of well-defined and distinct items; that is, there are no duplicate values within a set. In this assignment, your task is to design and implement (using an array) an ADT Set of integers that supports the following operations:
+Set()
// constructor that creates an empty set
+isEmpty() : Boolean
// Determines whether a set is empty
+size() : integer
// Returns the cardinality of the set
+add(in item : integer)
// Adds the specified element to this set if it is not
// already present
+contains(in item : integer) : Boolean
// Determines if this set contains the specified item
+union(in other : Set) : Set
// Creates a new set containing all of the elements of
// this set and the other set (no duplicates) and
// returns the resulting set
+intersection(in other : Set) : Set
// Creates a new set of elements that appear in both
// this set and the other set and returns the
// resulting set
+removeAll()
// Removes all of the items in the set
2. Your implementation should contain a class attribute called setArray that is an array of integers of length 10 and a class attribute called size that is initialized to 0.
3. On each call to add, check to see if setArray is full. If adding the new element would cause the array to overflow, then
create a new larger array that is twice the size of the original array;
copy the contents of the original array to the new array;
add the new element to the new array;
reassign setArray to reference the new array; and
increment the size attribute.
4. Your implementation should reset the size of setArray back to 10 if all the elements are removed (i.e. removeAll() is called).
I M P L E M E N T A T I O N H I N T S :
Sets contain no duplicate values. Therefore, attempting to add an item to a set that already contains the item will result in no change to the set. A set of integers will not contain duplicate values.
In set theory, the union of two sets is a new set that contains all of the items in the original two sets. For example, given a set S1 = { 1, 3, 5, 7, 9 } and a set S2 = { 2, 3, 4, 5, 6 }, the union of the two sets S1 S2 = { 1, 2, 3, 4, 5, 6, 7, 9 }.
In set theory, the intersection of two sets is a new set that contains only those items that are in both of the original sets. For example, given a set S1 = { 1, 3, 5, 7, 9 } and a set S2 = { 2, 3, 4, 5, 6 }, the intersection of the two sets S1 S2 = { 3, 5 }. If the two sets have no common items, then the intersection of the two sets is an empty set.
If implemented properly, the size attribute will double as both indicating how many items are stored in the set and as the index of the next available position.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
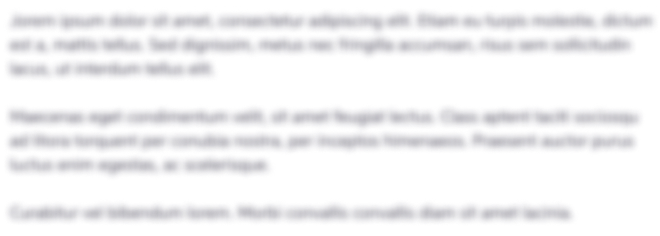
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started