Question
A soduko puzzle uses a 9X9 grid in which each column and row, as well as each of the nine 3X3 subgrids, must contain all
A soduko puzzle uses a 9X9 grid in which each column and row, as well as each of the nine 3X3 subgrids, must contain all of the digits 1 to 9.
6 | 5 | 3 | 1 | 2 | 8 | 7 | 9 | 4 |
1 | 7 | 4 | 3 | 5 | 9 | 6 | 8 | 2 |
9 | 2 | 8 | 4 | 6 | 7 | 5 | 3 | 1 |
2 | 8 | 6 | 5 | 1 | 4 | 3 | 7 | 9 |
3 | 9 | 1 | 7 | 8 | 2 | 4 | 5 | 6 |
5 | 4 | 7 | 6 | 9 | 3 | 2 | 1 | 8 |
8 | 6 | 5 | 2 | 3 | 1 | 9 | 4 | 7 |
4 | 1 | 2 | 9 | 7 | 5 | 8 | 6 | 3 |
7 | 3 | 9 | 8 | 4 | 6 | 1 | 2 | 5 |
Design a multithreaded application in C with Pthreads - it determines whether the solution to a Sudoku puzzle is valid.
There are several different ways of multithreading this application. One suggested strategy is to create threads that check the following criteria:
A thread to check that each column contains the digits 1 through 9 without duplication.
A thread to check that each row contains the digits 1 through 9 without duplication.
Nine threads to check that each of the 3X3 subgrids contains the digits 1 through 9 without duplication.
This would result in a total of eleven separate threads for validating a Sudoku puzzle. However, you are welcome to create even more (but not less) threads for this homework. For example, rather than creating one thread that checks all nine columns, you could create nine separate threads and have each of them check one column.
Representing the 9X9 Puzzle:
One may present the 9X9 grid with a two dimensional array as follows:
int grid [GRID_SIZE] [GRID_SIZE];
You can hard-code initial values to the array. For example, the following code initialize a 3X3 array:
int grid [3] [3] = { {12,3}, {4,5,6}, {7,8,9} };
where row 0 is {1,2,3}, row one is {4,5,6}, and row two is {7,8,9}. In row 0, column 0,1, and 2 has values 1,2, and 3, respectively; in row ne, column 0,1, and 2 has values 4,5,6, respectively; in row two, column 0,1,and 2 has values 7,8,and 9, respectively.
Passing Parameters to each Thread:
The parent thread will create the worker threads, passing each worker the location that it must check in the Sudoku grid. This step will require passing several parameters to each thread. The easiest approach is to create a data structure using a struc. For example, a structure to pass the row and column where a thread must begin validating would appear as follows:
/* structure for passing data to threads */
typedef struct {
int row;
int column;}
parameters;
With Pthreads, one can create worker threads using a strategy similar to that shown below:
parameters *data = (parameters *) malloc(size of (parameters));
data -> row = 1;
data -> column = 1; /* Now create the thread passing it data as a parameter */
The data pointer will be passed to the pthread_create(), which in turn will pass it as a parameter to the function that is to run as a separate thread. You should deallocate each of the memory blocks by using free() after a thread completes.
Returning Results to the Parent Thread:
Each worker thread is assigned the task of determining the validity of a particular region of the Soduku puzzle. Once a worker has performed this check, it must pass its results back to the parent. One good way to handle this is to create an array of integer values that is visible to each thread. The ith index in this array corresponds to the ith worker thread. If a worker sets its correspongding value to 1, it is indicating that its region of the Sudoku puzzle is valid. A value of 0 would indicate otherwise. When all workers threads have completed, the parent checks each entry in the result array to determine if the Sudoku puzzle is valid.
In your program, please hard-code the 9X9 grid with the solution as follows.
6,5,3,1,2,8,7,9,4,
1,7,4,3,5,9,6,8,2,
9,2,8,4,6,7,5,3,1,
2,8,6,5,1,4,3,7,9,
3,9,1,7,8,2,4,5,6,
5,4,7,6,9,3,2,1,8,
8,6,5,2,3,1,9,4,7,
4,1,2,9,7,5,8,6,3,
7,3,9,8,4,6,1,2,5
Step by Step Solution
There are 3 Steps involved in it
Step: 1
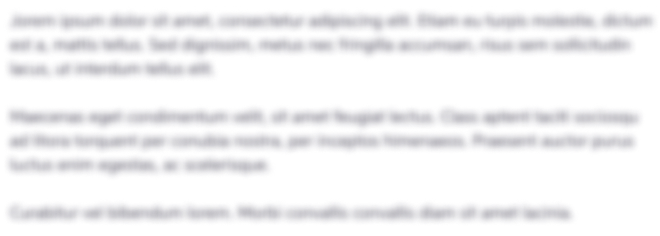
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started