Question
a. The Soda module: A FSM processis used to update the present state to the next state when a clocked coin is entered, this process
a. The Soda module:
A FSM processis used to update the present state to the next state when a clocked coin is entered, this process is used to show the description of the transitions of state machines from state to state using the Case statement.
Study the 35-cent soda vending machine VHDL description for this module below then complete part a VHDL programmed to dispense a product in the following manner:
The machine requires the equivalent of 7 nickels made of a combination of Quarters, Dimes and provides possible change.There are 7-coin states labeled sevenN(7 nickels needed), sixN (six more nickels needed), fiveN(five more nickels needed) etc... plus two dispense states, one with change, dispense_C and one without change, dispense_NC. All these states are declared as enumerated TYPE.
The coins can be accepted one at a time by pushing the KEY button.Initially, a green light, ready is on.The first state is labeled seven requiring the equivalent of seven nickels (7 x 5=35) made of a combination of quarters, dimes and nickels.
If a Quarter is entered, two more nickels are needed, so the next state will be twoN(two more nickels needed).
If a Dime is entered, five more nickels are needed, so the next state will be fiveN.
If a Nickel is entered, six more nickels are needed, so the next state will be sixN.
Also, the add_coin output is activated when more change is required into the machine.
If no coin is entered, the next state will be the present state sevenN.The process repeats for all the other states.
Procedure:a. Soda module
This module uses FSM design where one process will be used to update the present state to the next state when a clocked coin is entered, it is used to show the description of the transitions of state machines from state to state using the case statement. See more description above under discussion.Copy and paste the code below then complete it using the discussion above. (note: there are 2 copies for dispense, dispens0 and dispense1)
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.STD_LOGIC_ARITH.ALL;
use IEEE.STD_LOGIC_UNSIGNED.ALL;
entity soda is port (___,___,____,___,____: ___ ____; --reset,clock,Quarter Q, Dime D, Nickel N
___,___,____,___,____: ___ ____); -- ready, add_coin, change, dispense0,
dispense1
end soda;
architecture behavioral of soda is
Type states is (oneN, twoN, threeN, fourN, fiveN, sixN, sevenN, dispense_NC, dispense_C);
Signal next_state: states; beginprocess (____,_____,____,____,____) --sensitivity list begin
ready <= '0'; --describe default state
dispense0<= '0';
dispense1<= '0';
more_coin<= '0';
change<= '0';
if reset ='0' then next_state <=_______; --on reset always go to sevenN
elsif ____________(clk) then --on rising edge of clock
case next_state is
when sevenN => -- seven Nickels needed
--describe this state below
ready <= '__'; --activate ready
dispense0<= '__'; --no dispense yet
dispense1<= __';
add_coin<= '__'; --no coin detected yet
change<= '__'; --no change yet
--Check for incoming coins
if Q='1' then--check if quarter
next_state<= ____;
elsif D='1' then--check if Dime
next_state<= _____;
elsif N='1' then --check if Nickel
next_state<= ____;
else next_state<= ______; --stay
end if;
when sixN => -- six Nickels needed
--describe this state below
ready <= '__'; --deactivate ready
dispense0<= '__'; --no dispense yet
dispense1<= __';
add_coin<= '__'; --ask for more coins
change<= '__'; --no change yet
--Check for incoming coins
if Q='1' then
--check if quarter
next_state<= ____;
elsif D='1' then--check if Dime
next_state<= _____;
elsif N='1' then --check if Nickel
next_state<= ____;
else next_state<= _____; --stay
end if;
when fiveN => -- five Nickels needed
--describe this state below
ready <= '__'; --deactivate ready
dispense0<= '__'; --no dispense yet
dispense1<= __';add_coin<= '__'; --ask for more coins
change<= '__'; --no change yet
--Check for incoming coins
if Q='1' then--check if quarter
next_state<= ____;
elsif D='1' then--check if Dime
next_state<= _____;
elsif N='1' then --check if Nickel
next_state<= ____;
else next_state<= _____; --stay
end if;
when fourN => --four Nickels needed
--describe this state below
ready <= '__'; --deactivate ready
dispense0<= '__'; --no dispense yet
dispense1<= __';
add_coin<= '__'; --ask for more coins
change<= '__'; --no change yet--Check for incoming coins
if Q='1' then--check if quarter
next_state<= ____;
elsif D='1' then--check if Dime
next_state<= _____;
elsif N='1' then --check if Nickel
next_state<= ____;
else next_state<= ___;--stay
end if;
when threeN => -- three Nickels needed
--describe this state belowready <= '__'; --deactivate
ready dispense0<= '__'; --no dispense yet
dispense1<= __';
add_coin<= '__'; --ask for more coins
change<= '__'; --no change yet--Check for incoming coins
if Q='1' then--check if quarter
next_state<= ____;
elsif D='1' then--check if Dime
next_state<= _____;
elsif N='1' then --check if Nickel
next_state<= ____;
else next_state<= ______; --stay
end if;
when twoN => -- two Nickels needed
--describe this state below
ready <= '__'; --deactivate ready
dispense0<= '__'; --no dispense yet
dispense1<= __';
add_coin<= '__'; --ask for more coins
change<= '__'; --no change yet
--Check for incoming coins
if Q='1' then --check if quarter
next_state<= ____;
elsif D='1' then--check if Dime
next_state<= _____;
elsif N='1' then --check if Nickel
next_state<= ____;
else next_state<= _______;--stay
end if;
when oneN => -- one Nickel needed
--describe this state below
ready <= '__'; --deactivate ready
dispense0<= '__'; --no dispense yet
dispense1<= __';add_coin<= '__'; --ask for more coins
change<= '__'; --no change yet
--Check for incoming coins
if Q='1' then --check if quarter
next_state<= ____;
elsif D='1' then--check if Dime
next_state<= _____;
elsif N='1' then --check if Nickel
next_state<= ____;
else next_state<= ________; --stay
end if;
when dispense_C => --dispense with change
ready<='__';
add_coin<= '__';
change<= '___'; --activate change
dispense0<= '__'; --activate dispense
dispense1<= '__';
next_state<= next_state;
when dispense_NC => --dispense without change
ready<='__';
add_coin<= '__';
change<= '___'; --no change
dispense0<= '__'; --activate dispense
dispense1<= '__';
next_state<= next_state;
end case;
end if;
end process;
end behavioral;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
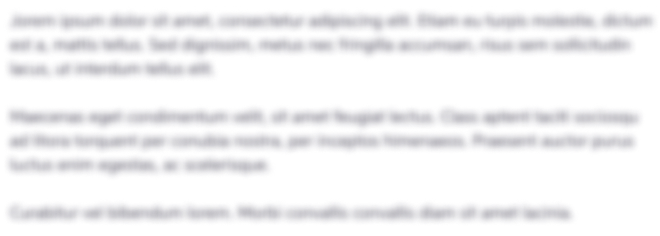
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started