Question
A UML class diagram import javax.swing.*; import java.awt.event.ActionEvent; import java.io.IOException; import java.util.EmptyStackException; public class P2GUI extends JFrame { // Variables private String expression; // Creates
A UML class diagram
import javax.swing.*; import java.awt.event.ActionEvent; import java.io.IOException; import java.util.EmptyStackException;
public class P2GUI extends JFrame {
// Variables private String expression;
// Creates Instance of ExpressionEval private ExpressionEval expressionEval = new ExpressionEval();
/** * Creates the GUI for the program, as well as handles Action Listeners */ private P2GUI() { // Set Title super("Three Address Generator");
// Create Panels JPanel main = new JPanel(); JPanel inputPanel = new JPanel(); JPanel constructPanel = new JPanel(); JPanel resultPanel = new JPanel();
// Set Layout main.setLayout(new BoxLayout(main, BoxLayout.Y_AXIS));
// Create Components JLabel inputLabel = new JLabel("Enter Postfix Expression"); JTextField inputTxt = new JTextField(null,20); JButton constructBtn = new JButton("Construct Tree"); JLabel resultLabel = new JLabel("Infix Expression"); JTextField resultTxt = new JTextField(null,20);
// Add Input Components inputPanel.add(inputLabel); inputPanel.add(inputTxt);
// Add Construct Components constructPanel.add(constructBtn);
// Add Result Components resultPanel.add(resultLabel); resultPanel.add(resultTxt); resultTxt.setEditable(false);
// Add Panels to main main.add(inputPanel); main.add(constructPanel); main.add(resultPanel);
// Add main to JFrame add(main);
// JFrame Settings setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); setSize(400,145); setLocationRelativeTo(null); setResizable(false); setVisible(true);
// "Construct Tree" Button Handler constructBtn.addActionListener((ActionEvent e) -> { expression = inputTxt.getText();
try { if (expression.isEmpty()) { throw new NullPointerException(); } else { resultTxt.setText(expressionEval.evaluate(expression)); } } catch (InvalidTokenException e1) { JOptionPane.showMessageDialog(null, "Invalid Token: " + e1.getMessage(),"Error",JOptionPane.ERROR_MESSAGE); } catch (NullPointerException e2) { JOptionPane.showMessageDialog(null, "A Postfix Expression is required.","Error",JOptionPane.ERROR_MESSAGE); } catch (EmptyStackException empty) { JOptionPane.showMessageDialog(null, "Invalid Postfix Expression. Check Operators and Operands.","Error",JOptionPane.ERROR_MESSAGE); } catch (IOException e1) { JOptionPane.showMessageDialog(null, "Error Writing to File.","Error",JOptionPane.ERROR_MESSAGE); } }); }
public static void main(String[] args) { new P2GUI(); } } ----------------------------------------------------------------------------------------------------- import java.io.IOException; import java.util.EmptyStackException; import java.util.Stack;
class ExpressionEval {
// Variables private Stack
String evaluate(String expression) throws InvalidTokenException, EmptyStackException, IOException { String[] tokens = expression.split(" ");
for (String token : tokens) { // Determines patterns used to determine tokens String operandPat = "[\\d.?]+"; String operatorPat = "[*/+\\-]";
// Ensures no illegal characters are used if (!token.matches(operandPat) && !token.matches(operatorPat)) { throw new InvalidTokenException(token); }
// Pushes Operands onto Stack. Assigns children to Operators, then pushes Operators onto Stack if (token.matches(operandPat)) { expressionStack.push(new OperandNode(token)); } else if (token.matches(operatorPat)) { operator = new OperatorNode(token, expressionStack.pop(), expressionStack.pop()); expressionStack.push(operator); } }
// Creates the Assembly Code written to File operator.post();
return expressionStack.pop().inOrderWalk(); } } ------------------------------------------------------------------------------------------------------ public class InvalidTokenException extends Exception { public InvalidTokenException(String token) { super(token); } } ------------------------------------------------------------------------------- import java.io.IOException;
public interface Node { String inOrderWalk(); String postOrderWalk() throws IOException; void post() throws IOException; } ----------------------------------------------------------------------------- public class OperandNode implements Node {
private String value;
/** * Assigns values to proper variables */ OperandNode(String value) { this.value = value; }
/** * Used for creating the Infix Expression */ public String inOrderWalk() { return String.valueOf(value); }
/** * Used for creating the Postfix Expression, as well as 3-Address.txt */ public String postOrderWalk() { return String.valueOf(value); }
/** * Helper method for postOrderWalk */ public void post() {} } --------------------------------------------------------------------------------------------- import java.io.BufferedWriter; import java.io.File; import java.io.FileWriter; import java.io.IOException;
public class OperatorNode implements Node {
// Variables private String operator; private Node right, left; private static int i; // register count for 3-address code private File file = new File("3-Address.txt"); // creates 3-Address text file on users Desktop
OperatorNode(String operator, Node right, Node left) { this.operator = operator; this.right = right; this.left = left;
operatorEval(operator); }
/** * Create the Infix Expression */ public String inOrderWalk() { return "(" + left.inOrderWalk() + " " + operator + " " + right.inOrderWalk() + ")"; }
/** * Helper method for postOrderWalk */ public void post() throws IOException { i = 0; // sets count postOrderWalk(); // starts the walk }
/** * Walks tree in PostOrder, then writes each step in expression to file */ public String postOrderWalk() throws IOException { String leftValue = left.postOrderWalk(); String rightValue = right.postOrderWalk(); String opValue = operatorEval(this.operator);
String result = "R" + i++;
// Creates string with a step in the expression then writes to file String step = opValue + " " + result + " " + leftValue + " " + rightValue; writeToFile(step);
return result; }
/** * Assigns a word to each type of operator, helper method for postOrderWalk */ private String operatorEval(String operator) { String op = "";
switch (operator) { case "+": op = "Add"; break; case "-": op = "Sub"; break; case "*": op = "Mul"; break; case "/": op = "Div"; break; }
return op; }
/** * Writes a line to the 3-Address.txt file, helper method for postOrderWalk */ private void writeToFile(String step) throws IOException { BufferedWriter bw = new BufferedWriter(new FileWriter(file,true)); bw.write(step); bw.newLine(); bw.close(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
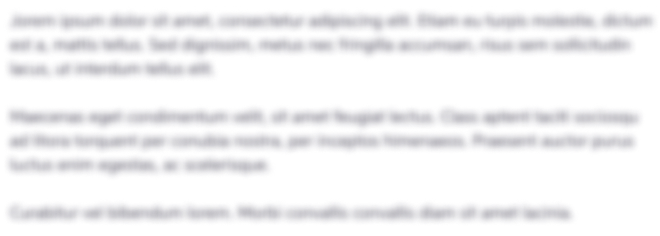
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started