Answered step by step
Verified Expert Solution
Question
1 Approved Answer
According to requirements to rewrite the following code. #**************************************************************************************** # FILE: h3-2.s # # DESCRIPTION # CSE/EEE 230 Spring 2017 Homework Assignment 3, Exercise 6
According to requirements to rewrite the following code. #**************************************************************************************** # FILE: h3-2.s # # DESCRIPTION # CSE/EEE 230 Spring 2017 Homework Assignment 3, Exercise 6 # # AUTHOR #6. (14 pts) Download ha 3-2.8 from the course website. Read Appendiz A which discusses how local array variables are allocated in the stack frame of a function and how an array is passed as an argument in a function call. Let array be a 1-dimensional array of ints. The size of array i.e., the number of elements in array, is specified by variable m. Shown below is the pseudocode for a function coun which has array and n as parameters. This function walks through array counting the number of negative values and the number of non-negative values in the array. It returns both counts. function count (array int0, n int) int, int, int i, neg, nonneg for i 0 to n -1 do if array i 0 then neg neg 1 else nonneg nonneg 1 end for return neg, nonneg end function search Complete the following exercises (name your source code file h3-2.s) a. Rewrite the pseudocode for count0 converting the for loop into a functionally-equivalent while loop. b. Rewrite the coun function from Exercise 6a converting the code using a while loop into equivalent code that only uses if statements and goto's. Also, rewrite the ifelse statement inside the loops to only use an if statement and a goto. c. The rewritten coun function from 6b should now map directly onto the assembly language version of the func tion. Write the function in assembly language. d. Test your program using the provided main() function in h3-2.s which allocates an array of 128 integers, with each element being a randomly-generated integer in the range 1500, 500. main() calls count0 passing the address of a in the $a0 register. count must return the values of local variables neg and nonreg in the $v0 and $v1 registers. With the pseudorandom number generator seed 1234, the array a should contain 69 negative integers and 59 non-negative integers( ) # ( ) #**************************************************************************************** #======================================================================================== # Equivalents for MARS System Calls #======================================================================================== .eqv SYS_EXIT 10 .eqv SYS_PRINT_CHAR 11 .eqv SYS_PRINT_INT 1 .eqv SYS_PRINT_STR 4 .eqv SYS_RAND_INT_RNG 42 .eqv SYS_READ_INT 5 .eqv SYS_SET_SEED 40 #======================================================================================== # Data Section # # Contains global data for the program. #======================================================================================== .data s_neg: .asciiz "Number of negative ints: " s_nonneg: .asciiz "Number of non-negative ints: " #======================================================================================== # Text Section # # Contains instructions for the program. #======================================================================================== .text #---------------------------------------------------------------------------------------- # main() - Allocates an array of 128 ints, fills it up with random integers in [-500, # 500], and calls count() which returns the number of negative and non-negative ints. # Displays the two counts and then terminates. # # PSEUDOCODE # function main () # int a[128], i, neg, nonneg # SysSetSeed (1, 1234) # for i = 0 to 127 do # a[i] = SysRandInt (1, 1000) - 500 -- generates random integers in [-500, 500] # end for # neg, nonneg = count (a, 128) # SysPrintStr ("Number of negative ints: ") # SysReadInt (neg) # SysPrintChar (' ') # SysPrintStr ("Number of non-negative ints: ") # SysReadInt (nonneg) # SysPrintChar (' ') # SysExit () # end function main # # The following pseudocode is main() rewritten to convert the for loop into a while # loop, # # function main () # int a[128], i, neg, nonneg # SysSetSeed (1, 1234) # i = 0 # while i 127 then goto main_loop_end # a[i] = SysRandInt (1, 1000) - 500 -- generates random integers in [-500, 500] # i = i + 1 # goto main_loop_begin # main_loop_end: # neg, nonneg = count (a, 128) # SysPrintStr ("Number of negative ints: ") # SysReadInt (neg) # SysPrintChar (' ') # SysPrintStr ("Number of non-negative ints: ") # SysReadInt (nonneg) # SysPrintChar (' ') # SysExit () # end function main # # This final revision now maps directly onto the assembly language implementation. # # STACK FRAME # main() must allocate 131 words for local variables a, i, neg, and nonneg. Since # main() does not return, we do not need to save $ra. The stack frame will look like # this, # # +----------+ # | | 127 drop out of loop # a[i] = SysRandInt(1, 1000) - 500 addi $v0, $zero, SYS_RAND_INT_RNG # $v0 = SysRandInt service code addi $a0, $zero, 1 # $a0 = pseudorandom number generator id addi $a1, $zero, 1000 # $a1 = upper limit of range [0, 1000] syscall # SysRandInt(1, 1000) sub $t1, $a0, $t8 # $a0 = SysRandInt(1, 1000) - 500 sll $t0, $t0, 2 # $t0 = 4i add $t0, $sp, $t0 # $t0 = a + 4i = &a[i] sw $t1, 0($t0) # a[i] = SysRandInt(1, 1000) - 500 # i = i + 1 lw $t0, 512($sp) # $t0 = i addi $t0, $t0, 1 # $t0 = i + 1 sw $t0, 512($sp) # i = i + 1 j main_loop_begin # continue looping main_loop_end: move $a0, $sp # $a0 = &a addi $a1, $zero, 128 # $a1 = 128 jal count # Call count(a, 128) sw $v0, 516($sp) # neg = first return value sw $v1, 520($sp) # nonneg = second return value # SysPrintStr ("Number of negative ints: ") addi $v0, $zero, SYS_PRINT_STR # $v0 = SysPrintStr service code la $a0, s_neg # $a0 = address of string to be printed syscall # Call SysPrintStr(...) # SysPrintInt (neg) addi $v0, $zero, SYS_PRINT_INT # $v0 = SysPrintInt service code lw $a0, 516($sp) # $a0 = neg syscall # SysPrintInt(neg) # SysPrintChar(' ') addi $v0, $zero, SYS_PRINT_CHAR # $v0 = SysPrintChar service code addi $a0, $zero, ' ' # $a0 = ' ' syscall # SysPrintChar(' ') # SysPrintStr ("Number of non-negative ints: ") addi $v0, $zero, SYS_PRINT_STR # $v0 = SysPrintStr service code la $a0, s_nonneg # $a0 = address of string to be printed syscall # Call SysPrintStr(...) # SysPrintInt (nonneg) addi $v0, $zero, SYS_PRINT_INT # $v0 = SysPrintInt service code lw $a0, 520($sp) # $a0 = nonneg syscall # SysPrintInt(nonneg) # SysPrintChar(' ') addi $v0, $zero, SYS_PRINT_CHAR # $v0 = SysPrintChar service code addi $a0, $zero, ' ' # $a0 = ' ' syscall # SysPrintChar(' ') # Call SysExit() addi $sp, $sp, 524 # Deallocate stack frame addi $v0, $zero, SYS_EXIT # $v0 = SysExit service code syscall # Call SysExit() #---------------------------------------------------------------------------------------- # count() # # ARGUMENTS # $a0 - array (the address of the 'array' variable allocated in main()'s stack frame # $a1 - n (the size of the array) #---------------------------------------------------------------------------------------- count:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
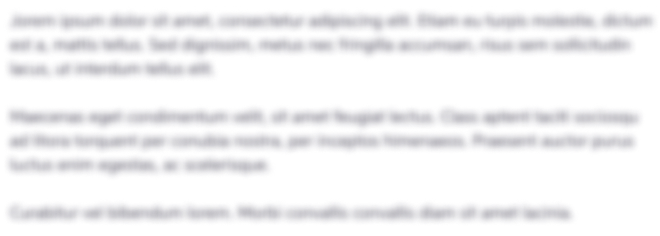
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started