Question
Activity mastermind package core package userInterface package RoundCornerButton.java Download class and add to the userInterface package RoundButton.java Download class and add to the userInterface package
Activity | ||
mastermind package |
|
|
core package |
|
|
userInterface package |
|
|
RoundCornerButton.java | Download class and add to the userInterface package |
|
RoundButton.java | Download class and add to the userInterface package |
|
CodebreakerUi.java | Add member variables of type: RoundButton[] buttons; RoundButton[][] attempts; |
|
| Update method initComponents(), it should do the followingFor the codebreakerColors JPanel Set the layout manager to use the default FlowLayout Instantiate the 1-D array buttons of RoundButton objects to the size of eight Loop through the array to do the following:Instantiate and instance of class RoundButton Instantiate an instance of class Color set equal to one of the colors stored in the Constants. codeColors ArrayList Set the background color of the instance of class RoundButton in step 1 above using the instance of class Color in step 2 above as an argument Call method .putClientProperty() on the instance of class RoundButton in step 1 above passing argument color and the instance of class Color in step 2 above Evaluate the instance of class Color in step 2 above to set the tool tip text of the RoundButton instance in step 1 above, call method setToolTipText on the RoundButton instance passing the Color instance as an argument Add the RoundButton instance to the codebreakerColors JPanel |
|
| Update method initComponents(), it should do the followingFor the codebreakerAttempt JPanel Set the layout manager to use GridLayout so that it is 10 rows by 4 columns Instantiate the 2-D array attempts of RoundButton objects to the size of 10 rows by 4 columns Loop through the array to do the following:Instantiate and instance of class RoundButton Disable the RoundButton instance if it isnt in the bottom row on the UI Add the RoundButton instance to the codebreakerAttempt JPanel |
|
CodemakerUi.java | Add member variables of type: JLabel[] secretLabels; JLabel[][] responseLabels; ImageIcon question; |
|
| Update method initComponents(), it should do the followingFor the secretCode JPanel Set the layout manager to use the default FlowLayout Instantiate the 1-D array secretLabels of JLabel objects to the size of four (2 points) Instantiate the instance of class ImageIcon passing as an argument to the constructor similar to the following: getClass().getResource("question.jpg"); the JPG passed is the image I used, you will need to search for an image that you desire to use, save this image to the userInterface package (10 points) Loop through the array to do the following:Instantiate an instance of class JLabel (2 points) Instantiate an instance of class ImageIcon set equal to the method call imageResize() passing as an argument the instance of ImageIcon in step iii above (NOTE: source code for method imageResize() is provided before figure 1 below) (10 points) Call method setIcon() on the JLabel instance in step 1 above passing as an argument the instance of class ImageIcon in step iii above (4 points) Add the JLabel instance to the secretCode JPanel (2 points) Instantiate an instance of class JButton passing as an argument text Check or something similar (3 points) Add the JButton instance in step v above to the secretCode JPanel (2 points) |
|
| Update method initComponents(), it should do the followingFor the codemakerResponse JPanel Instantiate the 2-D array responseLabels of JLabel objects to the size of 10 rows by 4 columns Loop through the array to do the following:Instantiate and instance of class JLabel Call method .setBorder() passing as an argument your desired border type from the BorderFactory class Add the JLabel instance to the codemakerResponse JPanel |
|
Mastermind application |
|
|
Test Case 1 | Test Case 1 passes |
|
Test Case 2 | Test Case 2 passes |
|
Test Case 3 | Test Case 3 passes |
|
Test Case 4 | Test Case 4 passes |
|
Test Case 5 | Test Case 5 passes |
|
| Source compiles with no errors |
|
| Source runs with no errors |
|
| Source includes comments |
|
Total |
|
|
Perform the following test cases
Test Cases | ||
| Action | Expected outcome |
Test Case 1 | Rregression Testing: Initial JOptionPane displays | JOptionPane is similar to figure 1 |
Test Case 2 | Mastermind Initial UI displays | Mastermind UI looks similar figure 2 |
Test Case 3 | Enabled buttons are selectable | Button should highlight, similar to figure 3 |
Test Case 4 | Disabled buttons are not selectable | Button should not highlight, similar to figure 4 |
Test Case 5 | Project view | Project view matches figure 5 except the image |
Source code to resize images
Note: The first two arguments passed to method getScaledInstance() represent the size in pixels of width and height.
private ImageIcon imageResize(ImageIcon icon)
{
Image image = icon.getImage();
Image newImage = image.getScaledInstance(30, 30, java.awt.Image.SCALE_SMOOTH);
icon = new ImageIcon(newImage);
return icon;
}
Current Code:
package constants;
import java.awt.Color; import java.util.ArrayList; import java.util.Arrays;
public class Constants { // peg color options public static final ArrayList
package core;
import java.awt.Color; import java.util.ArrayList; import constants.Constants; import java.util.Scanner;
public class Codebreaker implements ICodebreaker {
// member variables private ArrayList switch(guess.toUpperCase()) { case "BLUE": System.out.println("You enteted blue"); codebreakerAttempt.add(Color.BLUE); break; case "BLACK": System.out.println("You enteted black"); codebreakerAttempt.add(Color.BLACK); break; case "GREEN": System.out.println("You enteted green"); codebreakerAttempt.add(Color.GREEN); break; case "ORANGE": System.out.println("You enteted orange"); codebreakerAttempt.add(Color.ORANGE); break; case "PINK": System.out.println("You enteted pink"); codebreakerAttempt.add(Color.PINK); break; case "RED": System.out.println("You enteted red"); codebreakerAttempt.add(Color.RED); break; case "YELLOW": System.out.println("You enteted yellow"); codebreakerAttempt.add(Color.YELLOW); break; case "WHITE": System.out.println("You enteted white"); codebreakerAttempt.add(Color.WHITE); break; default: System.out.println("Invalid color choice, try again"); break; } if(codebreakerAttempt.size() getCodebreakerAttempt() { // temporary code to test backend consoleAttempt(); return codebreakerAttempt; } /** * @param codebreakerAttempt the codebreakerAttempt to set */ public void setCodebreakerAttempt(ArrayList package core; import constants.Constants; import java.awt.Color; import java.util.ArrayList; import java.util.HashSet; import java.util.List; import java.util.Random; import java.util.Set; public class Codemaker implements ICodemaker { // member variables private Set if((secretList.get(peg) != attempt.get(peg)) && (secretList.contains(attempt.get(peg))) && !countedPegs.contains(attempt.get(peg))) { System.out.println("Found correct color in wrong position"); // add a white peg whitePegs++; countedPegs.add(attempt.get(peg)); } } } } } evaluatePegs(redPegs, whitePegs); } private void evaluatePegs(int red, int white) { // clear the codemakerResponse codemakerResponse.removeAll(codemakerResponse); System.out.println("Red pegs " + red + " white pegs " + white); if(red == Constants.MAX_PEGS) { codeGuessed = true; } for(int r = 0; r getSecretCode() { return secretCode; } /** * @param secretCode the secretCode to set */ public void setSecretCode(Set /** * @return the codemakerResponse */ public ArrayList /** * @param codemakerResponse the codemakerResponse to set */ public void setCodemakerResponse(ArrayList /** * @return the codeGuessed */ public boolean isCodeGuessed() { return codeGuessed; } /** * @param codeGuessed the codeGuessed to set */ public void setCodeGuessed(boolean codeGuessed) { this.codeGuessed = codeGuessed; } } package core; import java.awt.Color; import java.lang.System; import java.util.ArrayList; import java.util.Scanner; import constants.Constants; public class Game implements IGame { private int attempt; private Codebreaker codebreaker; private Codemaker codemaker; public Game() { // instantiate the instances of the member variables codemaker = new Codemaker(); codebreaker = new Codebreaker(); attempt = 0; // play(); } public void play() { do{ attempt++; System.out.println("**** Attempt " + attempt + " ****"); ArrayList /** * @return the attempt */ public int getAttempt() { return attempt; } /** * @param attempt the attempt to set */ public void setAttempt(int attempt) { this.attempt = attempt; } /** * @return the codebreaker */ public Codebreaker getCodebreaker() { return codebreaker; } /** * @param codebreaker the codebreaker to set */ public void setCodebreaker(Codebreaker codebreaker) { this.codebreaker = codebreaker; } /** * @return the codemaker */ public Codemaker getCodemaker() { return codemaker; } /** * @param codemaker the codemaker to set */ public void setCodemaker(Codemaker codemaker) { this.codemaker = codemaker; } } package core; import java.awt.Color; import java.util.ArrayList; public interface ICodebreaker { public void checkCode(ArrayList package core; import java.awt.Color; import java.util.ArrayList; public interface ICodemaker { public void generateSecretCode(); public void checkAttemptedCode(ArrayList package core; public interface IGame { public void play(); } package mastermind; import core.Game; import javax.swing.JOptionPane; import userinterface.MasterMindUi; public class MasterMind { /** * @param args the command line arguments */ public static void main(String[] args) { System.out.println("Welcome to MasterMind!"); JOptionPane.showMessageDialog(null, "Let's Play MasterMind!"); Game game = new Game(); MasterMindUi ui = new MasterMindUi(game); } } package userinterface; import core.Codebreaker; import java.awt.Dimension; import java.awt.FlowLayout; import javax.swing.BorderFactory; import javax.swing.JPanel; import javax.swing.JButton; import userinterface.RoundButton; public class CodebreakerUi { RoundButton[] buttons; RoundButton[] attempts; private JPanel codebreakerAttempt; private JPanel codebreakerColors; private Codebreaker codebreaker; public CodebreakerUi(Codebreaker codebreaker) { this.codebreaker = codebreaker; initComponents(); } private void initComponents() { FlowLayout flowLay = new FlowLayout(); codebreakerColors.setLayout(flowLay); RoundButton[] buttons = new RoundButton[8]; initCodemakerColors(); initCodemakerAttempt(); } private void initCodemakerColors() { codebreakerColors = new JPanel(); codebreakerColors.setBorder(BorderFactory.createTitledBorder("Codebreaker Colors")); codebreakerColors.setMinimumSize(new Dimension(200, 50)); codebreakerColors.setPreferredSize(new Dimension(200,50)); } private void initCodemakerAttempt() { codebreakerAttempt = new JPanel(); codebreakerAttempt.setBorder(BorderFactory.createTitledBorder("Codebreaker Attempt")); codebreakerAttempt.setMinimumSize(new Dimension(200, 50)); codebreakerAttempt.setPreferredSize(new Dimension(200,50)); } /** * @return the codebreakerAttempt */ public JPanel getCodebreakerAttempt() { return codebreakerAttempt; } /** * @return the codebreakerColors */ public JPanel getCodebreakerColors() { return codebreakerColors; } } package userinterface; import core.Codemaker; import java.awt.Dimension; import javax.swing.BorderFactory; import javax.swing.JPanel; public class CodemakerUi { private JPanel codemakerResponse; private JPanel secretCode; private Codemaker codemaker; public CodemakerUi(Codemaker codemaker) { this.codemaker = codemaker; initComponents(); } private void initComponents() { initCodemakerResponse(); initSecretCode(); } private void initCodemakerResponse() { codemakerResponse = new JPanel(); codemakerResponse.setBorder(BorderFactory.createTitledBorder("Codemaker Response")); codemakerResponse.setMinimumSize(new Dimension(200, 50)); codemakerResponse.setPreferredSize(new Dimension(200,50)); } private void initSecretCode() { secretCode = new JPanel(); secretCode.setBorder(BorderFactory.createTitledBorder("Secret Code")); secretCode.setMinimumSize(new Dimension(200, 50)); secretCode.setPreferredSize(new Dimension(200,50)); } /** * @return the codemakerResponse */ public JPanel getCodemakerResponse() { return codemakerResponse; } /** * @return the secretCode */ public JPanel getSecretCode() { return secretCode; } } package userinterface; import core.Game; import constants.Constants; import java.awt.BorderLayout; import java.awt.Desktop; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.File; import javax.swing.JFrame; import javax.swing.JMenu; import javax.swing.JMenuBar; import javax.swing.JMenuItem; import javax.swing.JOptionPane; public class MasterMindUi { private Game game; private CodebreakerUi codebreakerUi; private CodemakerUi codemakerUi; private JFrame frame; private JMenuBar menuBar; private JMenu gameMenu; private JMenu helpMenu; private JMenuItem newGameMenuItem; private JMenuItem exitMenuItem; private JMenuItem aboutMenuItem; private JMenuItem rulesMenuItem; public MasterMindUi(Game game) { this.game = game; initComponents(); } private void initComponents() { // instantiate the codebreaker and codemaker UIs codebreakerUi = new CodebreakerUi(game.getCodebreaker()); codemakerUi = new CodemakerUi(game.getCodemaker()); // create the jframe frame = new JFrame("Mastermind"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(660, 500); // create the jmenubar initMenuBar(); // add codebreaker UI components frame.add(codebreakerUi.getCodebreakerColors(), BorderLayout.SOUTH); frame.add(codebreakerUi.getCodebreakerAttempt(), BorderLayout.CENTER); // add codemaker UI components frame.add(codemakerUi.getSecretCode(), BorderLayout.NORTH); frame.add(codemakerUi.getCodemakerResponse(), BorderLayout.EAST); frame.setJMenuBar(menuBar); frame.setVisible(true); } private void initMenuBar() { menuBar = new JMenuBar(); // game menu gameMenu = new JMenu("Game"); newGameMenuItem = new JMenuItem("New Game"); exitMenuItem = new JMenuItem("Exit"); exitMenuItem.addActionListener(new ExitListener()); // help menu helpMenu = new JMenu("Help"); aboutMenuItem = new JMenuItem("About"); aboutMenuItem.addActionListener(new AboutListener()); rulesMenuItem = new JMenuItem("Game Rules"); rulesMenuItem.addActionListener(new RulesListener()); // put it all together gameMenu.add(newGameMenuItem); gameMenu.add(exitMenuItem); helpMenu.add(aboutMenuItem); helpMenu.add(rulesMenuItem); menuBar.add(gameMenu); menuBar.add(helpMenu); } // inner classes private class ExitListener implements ActionListener { @Override public void actionPerformed(ActionEvent ae) { int response = JOptionPane.showConfirmDialog(frame, "Confirm to exit Mastermind?", "Exit?", JOptionPane.YES_NO_OPTION); if (response == JOptionPane.YES_OPTION) System.exit(0); } } private class AboutListener implements ActionListener { @Override public void actionPerformed(ActionEvent ae) { String message = "Mastermind version 1.0 Karin Whiting Fall 2018"; JOptionPane.showMessageDialog(frame, message); } } private class RulesListener implements ActionListener { @Override public void actionPerformed(ActionEvent ae) { String stepOne = "Step 1: The codemaker selects a four color secret code, in any order, no duplicate colors. "; String stepTwo = "Step 2: The codebreaker places a guess in the bottom row, no duplicate colors. "; String stepThree = "Step 3: The codemaker gives feedback next to each guess row with four pegs " + "~ Each red peg means that one of the guessed colors is correct, and is in the right location. " + "~ Each white peg means that one of the guessed colors is correct, but is in the wrong location. "; String stepFour = "Step 4: Repeat with the next row, unless the secret code was guessed on the first turn "; String stepFive = "Step 5: Continue until the secret code is guessed or there are no more guesses left, there are 10 attempts "; JOptionPane.showMessageDialog(frame, stepOne + stepTwo + stepThree + stepFour + stepFive); } } } package userinterface; import java.awt.Dimension; import java.awt.geom.Ellipse2D; public class RoundButton extends RoundedCornerButton { protected RoundButton() { super(); } @Override public Dimension getPreferredSize() { Dimension d = super.getPreferredSize(); int s = Math.max(d.width, d.height); d.setSize(s, s); return d; } @Override protected void initShape() { if (!getBounds().equals(base)) { base = getBounds(); shape = new Ellipse2D.Double(0, 0, getWidth() - 1, getHeight() - 1); border = new Ellipse2D.Double(FOCUS_STROKE, FOCUS_STROKE, getWidth() - 1 - FOCUS_STROKE * 2, getHeight() - 1 - FOCUS_STROKE * 2); } } } package userinterface; import java.awt.Color; import java.awt.GradientPaint; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.RenderingHints; import java.awt.Shape; import java.awt.geom.RoundRectangle2D; import javax.swing.JButton; public class RoundedCornerButton extends JButton { private static final double ARC_WIDTH = 16d; private static final double ARC_HEIGHT = 16d; protected static final int FOCUS_STROKE = 2; protected final Color fc = new Color(100, 150, 255, 200); protected final Color ac = new Color(230, 230, 230); protected final Color rc = Color.ORANGE; protected Shape shape; protected Shape border; protected Shape base; protected RoundedCornerButton() { super(); } @Override public void updateUI() { super.updateUI(); setContentAreaFilled(false); setFocusPainted(false); setBackground(new Color(250, 250, 250)); initShape(); } protected void initShape() { if (!getBounds().equals(base)) { base = getBounds(); shape = new RoundRectangle2D.Double(0, 0, getWidth() - 1, getHeight() - 1, ARC_WIDTH, ARC_HEIGHT); border = new RoundRectangle2D.Double(FOCUS_STROKE, FOCUS_STROKE, getWidth() - 1 - FOCUS_STROKE * 2, getHeight() - 1 - FOCUS_STROKE * 2, ARC_WIDTH, ARC_HEIGHT); } } private void paintFocusAndRollover(Graphics2D g2, Color color) { g2.setPaint(new GradientPaint(0, 0, color, getWidth() - 1, getHeight() - 1, color.brighter(), true)); g2.fill(shape); g2.setPaint(getBackground()); g2.fill(border); } @Override protected void paintComponent(Graphics g) { initShape(); Graphics2D g2 = (Graphics2D) g.create(); g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); if (getModel().isArmed()) { g2.setPaint(ac); g2.fill(shape); } else if (isRolloverEnabled() && getModel().isRollover()) { paintFocusAndRollover(g2, rc); } else if (hasFocus()) { paintFocusAndRollover(g2, fc); } else { g2.setPaint(getBackground()); g2.fill(shape); } g2.dispose(); // super.paintComponent(g); } @Override protected void paintBorder(Graphics g) { initShape(); Graphics2D g2 = (Graphics2D) g.create(); g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); g2.setPaint(getForeground()); g2.draw(shape); g2.dispose(); } @Override public boolean contains(int x, int y) { initShape(); return shape != null && shape.contains(x, y); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
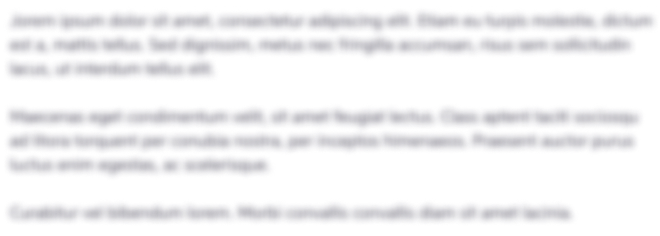
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started