Question
Add (and test!) the following methods (functions) to the EncapsulatedArray class (C++): - void swap (int index1, int index2) { swaps two elements of the
Add (and test!) the following methods (functions) to the EncapsulatedArray class (C++):
- void swap (int index1, int index2) { swaps two elements of the same array provided index1 and index2}
- void bubbleSort ( ) { uses the bubble sort algorithm implementation to sort the encapsulated array}
- void selectSort ( ) { uses the select sort algorithm implementation to sort the encapsulated array}
- void insertSort ( ) { uses the insert sort algorithm implementation to sort the encapsulated array}
- void deleteIndex (int index_to_delete) { deletes an element at index_to_delete, shifts-left elements on top of deleted element }
- void noDups ( ) { removes all duplicates from a sorted array, shifts-left elements on top of deleted elements }
- void insert_in_order ( int number_to_insert ) { insert a number in the correct order, in an already sorted array }
ENCAPSULATED ARRAY class: #includeusing namespace std; class Item { private: string name; double cost; public: Item() { name = ""; cost = 0.0; } Item(string newName, double newCost) { name = newName; cost = newCost; } void show() { cout << "- " << name << " $" << cost << endl; } string get_name() { return name; } double get_cost() { return cost; } void set_item(string newName, double newCost) { name = newName; cost = newCost; } void setItem(Item item) { name = item.get_name(); cost = item.get_cost(); } }; class Bag { private: Item *items; int number_items; int max; public: Bag(int Max) { items = new Item[Max]; // an array of items number_items = 0; // this is the index of current item max = Max; // size of the bag/array } void insert_item(string name, double cost) { items[number_items].set_item(name, cost); number_items++; } void insertItem(Item item) { // items[number_items].set_item(item.get_name(), item.get_cost()); items[number_items].setItem(item); number_items++; } int size() { return max; } int get_index_of(Item item) { // returns the index of an item (based on the name) int index = -1; for(int i = 0; i < number_items; i++) { if(items[i].get_name() == item.get_name()) { index = 1; break; } } return index; } void most_expensive() { // shows the most expensive item if(number_items > 0) { double maxExp = items[0].get_cost(); int index = 0; for (int i = 0; i < number_items; i++) { if (items[i].get_cost() > maxExp) { maxExp = items[i].get_cost(); index = i; } } cout << " The most expensive item: "; items[index].show(); } } void show_reverse() { // shows everything in the bag in reverse order of showItems() for(int i = number_items - 1; i >= 0; i--) items[i].show(); } void get_frequency(Item item) { // shows how many times an Item (the name) appears in the bag int count = 0; for(int i = 0; i < number_items; i++) { if(items[i].get_name() == item.get_name()) count++; } cout << " The item " << item.get_name() << " appears in the bag " << count << " time/times." << endl; } void showItems() { for(int i = 0; i < number_items; i++) items[i].show(); } void delete_first() { // shift all the elements one to the left for(int i = 0; i < max - 1; i++) items[i] = items[i+1]; number_items --; // resize bag } bool deleteItem(Item item) { // delete item(based on the name), shift array elements to the left, return true if successful int index = -1; for(int i = 0; i < number_items; i++) { if(items[i].get_name() == item.get_name()) { index = i; break; } } if(index != -1) { number_items--; for(int i = index; i < number_items; i++) { items[i] = items[i+1]; } return true; } else return false; } bool contains(string name_to_find) { for(int i = 0; i < number_items; i++) if(name_to_find == items[i].get_name()) return true; return false; } void sort_by_cost_acending() // bubble sort { for(int i = 0; i < number_items - 1; i++) for(int j = 0; j < number_items - 1; j++) if(items[j].get_cost() > items[j+1].get_cost()) swap(j, j+1); } void swap(int index1, int index2) { Item temp = items[index1]; items[index1] = items[index2]; items[index2] = temp; } double sum_of_all() { // returns the sum of all Items double sum = 0; for(int i = 0; i < number_items; i++) { sum += items[i].get_cost(); } return sum; } }; int main() { /// Test to see if Item works cout << "Testing Item: "; Item anItem("Fuji Apple", 4.99); Item anItem2("Green Apple", 5.99); Item anItem3("Apple", 8.99); Item anItem4("Mango", 6.99); Item anItem5("Pear", 3.99); anItem.show(); Bag b(10); b.insertItem(anItem); b.insertItem(anItem2); b.insertItem(anItem3); b.insertItem(anItem4); b.insertItem(anItem5); b.most_expensive(); cout << " Items in reverse: " << endl; b.show_reverse(); b.get_frequency(anItem); b.get_index_of(anItem3); b.deleteItem(anItem4); cout << " After deleting " << anItem.get_name() << ", items are: " << endl; b.showItems(); cout << " Sum of all items: " << b.sum_of_all(); return 0; } /* OUTPUT: /Users/dado/CLionProjects/SCIS46_SP2018/cmake-build-debug/SCIS46_SP2018 Testing Item: - Fuji Apple $4.99 The most expensive item: - Apple $8.99 Items in reverse: - Pear $3.99 - Mango $6.99 - Apple $8.99 - Green Apple $5.99 - Fuji Apple $4.99 The item Fuji Apple appears in the bag 1 time/times. After deleting Fuji Apple, items are: - Fuji Apple $4.99 - Green Apple $5.99 - Apple $8.99 - Pear $3.99 Sum of all items: 23.96 Process finished with exit code 0 */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
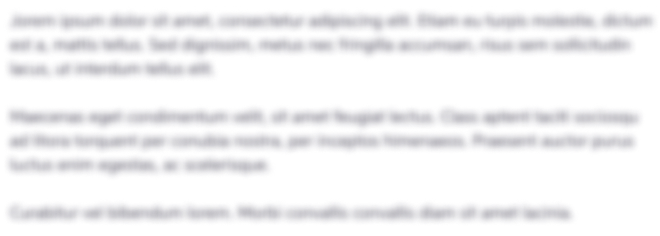
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started