Answered step by step
Verified Expert Solution
Question
1 Approved Answer
// Add header block comments here public class Asgn03 { public static Scanner cin = new Scanner(System.in); private static int cmdCount = 0; public static
// Add header block comments here public class Asgn03 { public static Scanner cin = new Scanner(System.in); private static int cmdCount = 0; public static void main(String[] args) { out.println("CPS 151 Assignment 3 (NumberedList) *Incomplete*"); NumberedListNoDupNumbered List (no duplicates) Implementation using linked list technique In this assignment you will implement a Numbered List class using Linked Lists. The clients view the items in the list to have a sequence number, but in the linked list the sequence numbers do not need to be stored with the items A Numbered List has zero or more items of a specific type (This is the type parameter for a generic class). Each item has a sequence number starting with 1 and going up to the size of the list. Items can be added to the list, or deleted from the list by specifying a position. This is very similar to ArrayList class of Java library, except that ArrayList positions start from 0. Consider the following numbered list (used to store a grocery list) 1. Bread 2. Milk 3. Cereal 4. Juice After the operation add(3, "Butter") the list becomes 1. Bread 2. Milk 3. Butter 4. Cereal 5. Juice Notice how two items now have their position numbers incremented. Now if we do the operation remove(2), the list will become 1. Bread 2. Butter 3. Cereal 4. Juice Once again some items change their position numbers No duplicates The class should not allow duplicate values to be stored Add and remove by value Like Java's ArrayList class, this class will allow adding an item without specifying a position, in which case the item gets added as the last item. A remove operation by specifying a value will remove it from whatever position it is in. Note that the item, if present, must be unique Size of the list This class supports the notion of size. The size is the number of values currently stored in the list. It would be convenient to have an instance variable hold this information . This variable is incremented for every successful add operation and is decremented for every successful remove operation. These are the only operations that modify the size. Numbered List (no duplicates) Implementation using linked list technique In this assignment you will implement a Numbered List class using Linked Lists. The clients view the items in the list to have a sequence number, but in the linked list the sequence numbers do not need to be stored with the items A Numbered List has zero or more items of a specific type (This is the type parameter for a generic class). Each item has a sequence number starting with 1 and going up to the size of the list. Items can be added to the list, or deleted from the list by specifying a position. This is very similar to ArrayList class of Java library, except that ArrayList positions start from 0. Consider the following numbered list (used to store a grocery list) 1. Bread 2. Milk 3. Cereal 4. Juice After the operation add(3, "Butter") the list becomes 1. Bread 2. Milk 3. Butter 4. Cereal 5. Juice Notice how two items now have their position numbers incremented. Now if we do the operation remove(2), the list will become 1. Bread 2. Butter 3. Cereal 4. Juice Once again some items change their position numbers No duplicates The class should not allow duplicate values to be stored Add and remove by value Like Java's ArrayList class, this class will allow adding an item without specifying a position, in which case the item gets added as the last item. A remove operation by specifying a value will remove it from whatever position it is in. Note that the item, if present, must be unique Size of the list This class supports the notion of size. The size is the number of values currently stored in the list. It would be convenient to have an instance variable hold this information . This variable is incremented for every successful add operation and is decremented for every successful remove operation. These are the only operations that modify the sizeL = new LinkedNumberedListNoDup(); char choice = showMenuGetChoice(); // process user's choice while (choice != 'Q') { applyChoice(choice, L); choice = showMenuGetChoice(); } // end loop out.println(" Goodbye"); } // end main private static void applyChoice(char choice, NumberedListNoDup L) { if (choice == 'A') handleAdd(L); else if (choice == 'C') { L.clear(); out.println("List cleared"); } else if (choice == 'F') handleFind(L); else if (choice == 'G') handleGet(L); else if (choice == 'P') out.println(" List contents: " + L); else if (choice == 'R') handleRemove(L); else if (choice == 'S') handleSet(L); else if (choice == 'Z') out.println("List size = "+ L.size()); else if (choice != 'Q') out.println("Unrecognized command"); } // end method static void displayMenu() { out.println(" Choices: Add, Clear, Find, Get, Print, Remove, Set, siZe, Quit"); cmdCount++; out.print(cmdCount + ". Your choice (A/C/F/G/P/R/S/Z/Q): "); } // end displayMenu private static char showMenuGetChoice() { displayMenu(); return cin.next().toUpperCase().charAt(0); } // end method private static int getInt(String prompt) { out.print(prompt); return cin.nextInt(); } // end method private static void handleAdd(NumberedListNoDup L) { out.println("Not implemented yet"); } private static void handleFind(NumberedListNoDup L) { out.println("Not implemented yet"); } private static void handleGet(NumberedListNoDup L) { out.println("Not implemented yet"); } private static void handleRemove(NumberedListNoDup L) { out.println("Not implemented yet"); } private static void handleSet(NumberedListNoDup L) { out.println("Not implemented yet"); } } // end class // ------------------ NumberedList interface and implementation /** * Completed by: Put your name here * @author of skeleton tsanyal1 */ interface NumberedListNoDup { boolean add(T item); boolean add(int position, T item); void clear(); // empties out the list int find(T item); // return 0 if item not in list T get(int position); // return null if invalid position boolean remove(T item); boolean remove(int position); boolean set(int position, T item); int size(); String toString(); } // end interface class Node { // instance variables public T data; public Node next; // constructor 1 public Node(T data) { this(data, null); } // constructor 2 public Node(T data, Node next) { this.data = data; this.next = next; } } // end class Node class LinkedNumberedListNoDup implements NumberedListNoDup { private int nodeCount; private Node head; // add a tail reference if you want to public LinkedNumberedListNoDup() { clear(); } public boolean add(T item) {return false;} public boolean add(int position, T item) {return false;} public boolean remove(T item) {return false;} public boolean remove(int position) {return false;} public T get(int position) {return null;} // return null if invalid position public boolean set(int position, T item) {return false;} public int size() {return 0;} public void clear() {head = null; nodeCount = 0;} // empties out the list public int find(T item) {return 0; } // return 0 if item not in list public String toString() {return "";} // add any private methods you may wanteed } // end class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
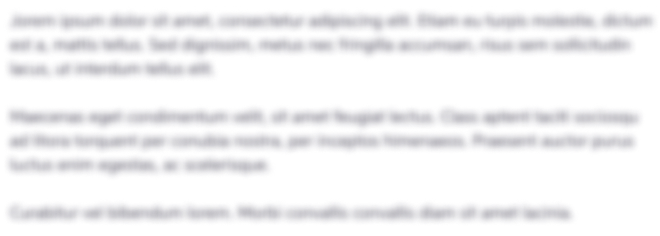
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started