Question
ALL Java Code finished, having a couple errors while running that I cant figure out. It is a multi thread chat app using diffie hellam
ALL Java Code finished, having a couple errors while running that I cant figure out. It is a multi thread chat app using diffie hellam security between clients and the server. 3 Classes provided below was wondering if you could figure out the errors? Thank You
DIFFIE SERVER CODE:
package server;
import java.io.IOException; import java.net.ServerSocket; import java.net.Socket; import java.util.ArrayList;
import net.Connection;
public class DiffieServer { private ServerSocket ssock; private ArrayList
public static void main(String args[]) { DiffieServer server = new DiffieServer(3333); server.start(); }
public DiffieServer(int port) { try { ssock = new ServerSocket(port); } catch (IOException e) { e.printStackTrace(); System.exit(1); } }
public void start() { System.out.println("Server started...");
clients = new ArrayList
try { while (true) { Socket csock = ssock.accept(); Connection newClient = new Connection(csock); newClient.sendKey(); clients.add(newClient); System.out.println("** New Client: " + csock.toString()); } } catch (IOException e) { e.printStackTrace(); System.exit(1); } }
private class BroadcastThread extends Thread { public void run() { while (true) { try { for (int i = 0; i < clients.size(); i++) { if (clients.get(i).hasMessage()) { String message = clients.get(i).getMessage(); broadcastMessage(message); System.out.println(message); } } Thread.sleep(500); } catch (Exception e) { e.printStackTrace(); System.exit(1); } } }
private void broadcastMessage(String message) { for (int i = 0; i < clients.size(); i++) { clients.get(i).sendMessage(message); } } } }
DIFFIE CLIENT CODE
package client;
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.Socket; import net.Connection;
public class DiffieClient { private Connection server;
public static void main(String args[]) { DiffieClient client = new DiffieClient("localhost", 3333); client.start(); }
public DiffieClient(String hostname, int port) { try { server = new Connection(new Socket(hostname, port)); } catch (IOException e) { e.printStackTrace(); System.exit(1); } }
public void start() { server.getKey(); BroadcastListener bcl = new BroadcastListener(); bcl.start();
BufferedReader sysin = new BufferedReader(new InputStreamReader( System.in)); while (true) { try { server.sendMessage(sysin.readLine()); } catch (IOException e) { e.printStackTrace(); System.exit(1); } } }
private class BroadcastListener extends Thread { public void run() { try { while (true) { if (server.hasMessage()) { System.out.println(server.getMessage()); } Thread.sleep(500); } } catch (Exception e) { e.printStackTrace(); System.exit(1); } } } }
CONNECTION CODE
package net; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.io.PrintStream; import java.math.BigInteger; import java.net.Socket; import java.util.Random; import java.util.Scanner;
public class Connection { private Socket socket; private BufferedReader br; private PrintStream ps; private BigInteger cipherKey;
public Connection(Socket sck) { try { socket = sck; br = new BufferedReader(new InputStreamReader( socket.getInputStream())); ps = new PrintStream(socket.getOutputStream()); } catch (IOException e) { e.printStackTrace(); System.exit(1); } }
public void sendKey() { try { // send P and Q to client BigInteger p = new BigInteger("5"); BigInteger q = new BigInteger("23"); ps.println(p.toString() + " " + q.toString() + " "); /*System.out.println("--> SEND: P=" + p.toString() + " Q=" + q.toString());*/
// receive public key from client BigInteger yourKey = new BigInteger(Integer.parseInt(br.readLine()) + ""); //System.out.println("<-- RECEIVE: yourKey=" + yourKey.toString());
// send public key to client Random rand = new Random(); BigInteger privateKey = new BigInteger((rand.nextInt(100) + 1) + ""); //System.out.println("(privateKey=" + privateKey.toString() + ")"); BigInteger publicKey = p.modPow(privateKey, q); ps.println(publicKey.toString() + " "); //System.out.println("--> SEND: publicKey=" + publicKey.toString());
// calculate cipher key cipherKey = yourKey.modPow(privateKey, q); //System.out.println("(cipherKey=" + cipherKey.toString() + ")"); } catch (IOException e) { e.printStackTrace(); System.exit(1); } }
public void getKey() { try { // receive P and Q from server Scanner ioscan = new Scanner(br.readLine()); BigInteger p = new BigInteger(ioscan.nextInt() + ""); BigInteger q = new BigInteger(ioscan.nextInt() + ""); /*System.out.println("<-- RECEIVE: P=" + p.toString() + " Q=" + q.toString());*/
// send public key to server Random rand = new Random(); BigInteger privateKey = new BigInteger((rand.nextInt(100) + 1) + ""); //System.out.println("(privateKey=" + privateKey.toString() + ")"); BigInteger publicKey = p.modPow(privateKey, q); ps.println(publicKey.toString() + " "); //System.out.println("--> SEND: publicKey=" + publicKey.toString());
// receive public key from server BigInteger yourKey = new BigInteger(Integer.parseInt(br.readLine()) + ""); //System.out.println("<-- RECEIVE: yourKey=" + yourKey.toString());
// calculate cipher key cipherKey = yourKey.modPow(privateKey, q); //System.out.println("(cipherKey=" + cipherKey.toString() + ")"); } catch (IOException e) { e.printStackTrace(); System.exit(1); } }
public boolean hasMessage() throws IOException { return br.ready(); }
public String getMessage() throws IOException { return decode(br.readLine()); }
public void sendMessage(String message) { ps.println(encode(message) + " "); }
private String encode(String message) { message = message.toUpperCase(); String encodedMessage = ""; for (int i = 0; i < message.length(); i++) { char thisChar = message.charAt(i); if (thisChar >= 65 && thisChar <= 90) { int shiftValue = 1 + Integer.parseInt(cipherKey.mod( new BigInteger("26")).toString());
//System.out.println("shiftValue = " + shiftValue); //System.out.println("thisChar (before) = " + thisChar);
thisChar += shiftValue; if (thisChar > 90) thisChar -= 26;
//System.out.println("thisChar (after) = " + thisChar); } //System.out.println("thisChar (appending) = " + thisChar); encodedMessage += thisChar; } return encodedMessage; }
private String decode(String message) { message = message.toUpperCase(); String decodedMessage = ""; for (int i = 0; i < message.length(); i++) { char thisChar = message.charAt(i); if (thisChar >= 65 && thisChar <= 90) { int shiftValue = 1 + Integer.parseInt(cipherKey.mod( new BigInteger("26")).toString()); thisChar -= shiftValue; if (thisChar < 65) thisChar += 26; } decodedMessage += thisChar; } return decodedMessage; }
public void close() { try { ps.close(); br.close(); socket.close(); } catch (IOException e) { e.printStackTrace(); System.exit(1); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
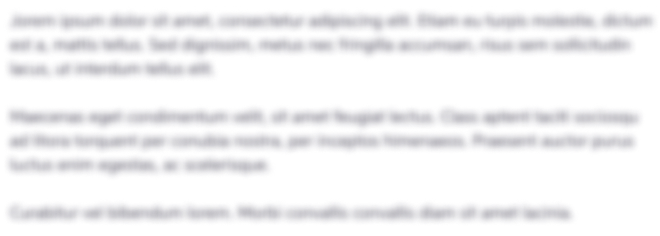
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started