Question
All that is needed is to fill in that one area that says Student provides missing code to compare computed hammingCode[] bits to the
All that is needed is to fill in that one area that says "Student provides missing code to compare computed hammingCode[] bits to the codeword[] bits to determine the incorrect bit." in the code below. THAT IS ALL THAT IS NEEDED PLUS SCREENSHOT OF COMPILED OUTPUT AFTERWARDS.
Please TAKE SCREENSHOT of the compiled output which should look similar to the sample one provided.
//------------------------------------------------------
// Problem #6
// Problem6.c
//------------------------------------------------------
#include
#include
#include
#include
#include
#include
#define MAXIMUMM 512
#define MAXIMUMR 10
typedef char BITS[MAXIMUMM+MAXIMUMR+1];
typedef enum { EVEN,ODD } PARITY;
//------------------------------------------------------
int main()
//------------------------------------------------------
{
void ComputeCodeWord(const BITS memoryWord,const PARITY parity,int *m,int *r,BITS codeWord);
void SetRandomSeed(void);
int RandomInt(const int LB,const int UB);
void ToggleBit(BITS word,const int bit);
void CorrectSingleBitError(const PARITY parity,const int m,const int r,BITS codeWord);
char fileName[80+1];
FILE *MEMORYWORDS;
BITS memoryWord,codeWord;
PARITY parity;
int m,r,n,bit;
printf("memory-words fileName? "); scanf("%s",fileName);
if ( (MEMORYWORDS = fopen(fileName,"r")) == NULL)
{
printf("Error opening memory-words file "%s"! ",fileName);
system("PAUSE");
exit( 1 );
}
SetRandomSeed();
while ( fgets(&memoryWord[1],MAXIMUMM,MEMORYWORDS) != NULL )
{
// Ignore memoryWord[0], erase ' ' at end of memoryWord[] string (if any)
memoryWord[0] = 'X';
if ( memoryWord[strlen(memoryWord)-1] == ' ' )
memoryWord[strlen(memoryWord)-1] = '';
/*
Determine and erase parity at end of memoryWord[] string (if any)--an 'E' or 'e'
suffix means (E)VEN, 'O' or 'o' suffix means (O)DD, and no suffix defaults to EVEN.
Here's BNF (Backus-Naur Form) that captures the preceding description of a memoryWord[]
*/
if ( toupper(memoryWord[strlen(memoryWord)-1]) == 'E' )
{
parity = EVEN;
memoryWord[strlen(memoryWord)-1] = '';
}
else if ( toupper(memoryWord[strlen(memoryWord)-1]) == 'O' )
{
parity = ODD;
memoryWord[strlen(memoryWord)-1] = '';
}
else
parity = EVEN;
// Compute codeWord[], m, r based on parity, memoryWord[]; display memoryWord[] and codeWord[]
ComputeCodeWord(memoryWord,parity,&m,&r,codeWord);
printf(" ");
printf("Memory word = %s%c ",&memoryWord[1],((parity == EVEN) ? 'E' : 'O'));
printf("Code word = %s original ",&codeWord[1]);
/*
Cause a random, single-bit error then show the erroroneous codeWord[];
correct the single-bit error; display corrected codeWord[]
*/
n = m+r;
bit = RandomInt(1,n);
ToggleBit(codeWord,bit);
printf("Code word = %s with error in bit #%d ",&codeWord[1],bit);
CorrectSingleBitError(parity,m,r,codeWord);
printf("Code word = %s with error corrected ", &codeWord[1]);
}
fclose(MEMORYWORDS);
system("PAUSE");
return( 0 );
}
//------------------------------------------------------
void ComputeCodeWord(const BITS memoryWord,const PARITY parity,int *m,int *r,BITS codeWord)
//------------------------------------------------------
{
int R(const int m);
bool IsPowerOf2(const int x);
int PowerOf2(const int exponent);
void ComputeHammingCode(const BITS codeWord,const PARITY parity,
const int m,const int r,BITS hammingCode);
int n,ri,mi,ci;
BITS hammingCode;
// Computer m, r, n
*m = strlen(memoryWord)-1;
*r = R(*m);
n = *m + *r;
/*
Place memoryWord[] bits into codeWord[] leaving room for the hammingCode[]
bits which are temporarily marked 'H'
*/
mi = 1;
for (ci = 1; ci
{
if ( IsPowerOf2(ci) )
codeWord[ci] = 'H';
else
{
codeWord[ci] = memoryWord[mi];
mi++;
}
}
codeWord[n+1] = '';
// Place hammingCode[] bits into codeWord[] thereby overwriting each 'H'
ComputeHammingCode(codeWord,parity,*m,*r,hammingCode);
for (ri = 1; ri
codeWord[PowerOf2(ri-1)] = hammingCode[ri];
}
//------------------------------------------------------
void ComputeHammingCode(const BITS codeWord,const PARITY parity,
const int m,const int r,BITS hammingCode)
//------------------------------------------------------
{
bool IsPowerOf2(const int x);
int PowerOf2(const int exponent);
int n = m+r;
int *sums = (int *) malloc(sizeof(int)*(r+1));
int ri,ci;
// Compute sum-of-bits for each hammingCode[] bit
for (ri = 1; ri
sums[ri] = 0;
for (ri = 1; ri
for (ci = PowerOf2(ri-1); ci
if ( !IsPowerOf2(ci) )
if ( ((ci >> (ri-1)) & 0X1) == 1 ) sums[ri] += (codeWord[ci] == '1') ? 1 : 0;
// Compute hammingCode[] bits for EVEN or ODD parity
for (ri = 1; ri
if ( sums[ri]%2 == 0 )
hammingCode[ri] = (parity == EVEN) ? '0' : '1';
else
hammingCode[ri] = (parity == EVEN) ? '1' : '0';
hammingCode[r+1] = '';
free(sums);
}
//------------------------------------------------------
void CorrectSingleBitError(const PARITY parity,const int m,const int r,BITS codeWord)
//------------------------------------------------------
{
void ComputeHammingCode(const BITS codeWord,const PARITY parity,
const int m,const int r,BITS hammingCode);
int PowerOf2(const int exponent);
void ToggleBit(BITS word,const int bit);
int ri,bit;
BITS hammingCode;
// Compute Hamming code bits
ComputeHammingCode(codeWord,parity,m,r,hammingCode);
// Compare computed Hamming code bits to codeWord bits to determine incorrect bit
Student provides missing code to compare computed hammingCode[] bits to the codeword[]
bits to determine the incorrect bit.
// Toggle incorrect bit
ToggleBit(codeWord,bit);
}
//------------------------------------------------------
int R(const int m)
//------------------------------------------------------
{
int PowerOf2(const int exponent);
int r = 1;
while ( (m+r+1) > PowerOf2(r) ) r++;
return( r );
}
//------------------------------------------------------
int PowerOf2(const int exponent)
//------------------------------------------------------
{
return( (int) pow(2,exponent) );
}
//------------------------------------------------------
bool IsPowerOf2(const int x)
//------------------------------------------------------
{
int powerOf2 = 1;
while ( powerOf2
powerOf2 *= 2;
return( (powerOf2 == x) ? true : false );
}
//------------------------------------------------------
void ToggleBit(BITS word,const int bit)
//------------------------------------------------------
{
word[bit] = (word[bit] == '1') ? '0' : '1';
}
//-----------------------------------------------------
// Functions below are "stolen" from the Dr. Hanna random-related pseudo-library
//-----------------------------------------------------
void SetRandomSeed(void)
//-----------------------------------------------------
{
srand( (unsigned int) time(NULL) );
}
//------------------------------------------------------
int RandomInt(const int LB,const int UB)
//------------------------------------------------------
{
double RandomDouble();
return( (int) ((UB-LB+1)*RandomDouble()) + LB );
}
//--------------------------------------------------
double RandomDouble()
//--------------------------------------------------
{
int R;
do
R = rand();
while ( R == RAND_MAX );
return( (double) R/RAND_MAX );
}
Problem Complete development of Problem6.c. Sample Input File, Problem6.in 1011 10101010e 01110101o 1111000010101110E 1111000010101110O 011110000110101010011101101010110 Sample Program Dialo CACOURSESICS23501Problems Problem6 Problem6.exe memory-words fileName? Problem6.in Memory word = 1011E Code word Code word Code word -0110011 original -1110011 with error in hit #1 = 0110011 with error corrected Memory word 10101010E Code word Code word Code word -111101001010 original -111101000010 with erro r in bit #9 - 111101001010 with error corrected Menory word 011101010 Code word Code word Code word -1001101 10101 with erro r in bit #6 Memory word 1111000010101110E Code word Code word Code word -001011100000101101110 original 001011000000101101 110 with error in bit #? - 001011100000101101110 with error CoFrected Memory word 11110000101011100 Code word Code word Code word or in bit #1 Memory word011110000110101010011101101010110 Code word Code word Code word Press any key to continueStep by Step Solution
There are 3 Steps involved in it
Step: 1
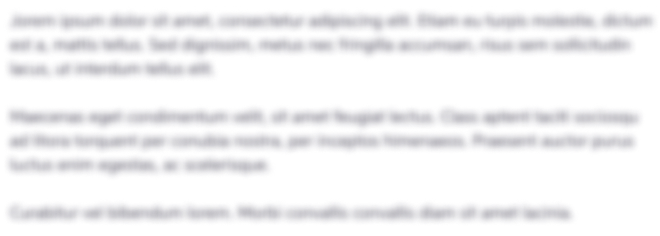
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started