Question
All your test code can reside in main. Below are the function prototype s that you must implement. Also, each function must be tested more
All your
test code can reside in main. Below are the function prototype
s
that you must implement.
Also, each
function must be tested more than once (ex. in your testIsThere
,
test for more than one numbe
r to insure
it works
(one you know is there and one you dont
at a minimum)
).
SortedType createTestList(int numbers[], int count);
//Used to create lists for your tests
...or if you prefer
void createTestList(SortedType &list, int numbers[], int count);
//
This would just populate your list
These functions should return true if the tests passed (which should be the case)
bool testIsThere();
bool testMergeLists();
bool testSplitLists();
Use the following data sets for Merge Lists:
List1
-
>0,3,1,5,9
List2
-
>7,4,6,10,8
...and for isThere and Split:
List
-
>0,3,1,5,9,7,4,6,10,8
All code must be commented including the functions themselves
. In NetB
eans, after you write the
function prototype, if you type /**
in the line above it
followed by enter, it will automa
tically create the
following for you (using creatTestList):
/**
*
* @param numbers
* @param count
* @return
*/
Finally, some well
-
formatted output would be nice
(actually
required
)
with the results from each test
using the functions return value
(true for pass)
, these printouts must not be in the functions themselves
.
bool SortedType::IsThere(ItemType item)
{
int midPoint;
int first = 0;
int last = length - 1;
bool moreToSearch = first <= last;
bool found = false;
while (moreToSearch && !found)
{
midPoint = (first + last) / 2;
switch (item.ComparedTo(info[midPoint]))
{
case LESS : last = midPoint - 1;
moreToSearch = first <= last;
break;
case GREATER : first = midPoint + 1;
moreToSearch = first <= last;
break;
case EQUAL : found = true;
break;
}
}
return found;
}
void MergeLists(SortedType list1, SortedType list2,
SortedType& result)
{
int length1;
int length2;
int counter1 = 1;
int counter2 = 1;
ItemType item1;
ItemType item2;
length1 = list1.GetLength();
length2 = list2.GetLength();
list1.ResetList();
list2.ResetList();
item1 = list1.GetNextItem();
item2 = list2.GetNextItem();
result.MakeEmpty();
while (counter1 <= length1 && counter2 <= length2)
switch (item1.ComparedTo(item2))
{
case LESS : result.PutItem(item1);
item1 = list1.GetNextItem();
counter1++;
break;
case EQUAL : result.PutItem(item1);
counter1++;
counter2++;
item1 = list1.GetNextItem();
item2 = list2.GetNextItem();
break;
case GREATER: result.PutItem(item2);
counter2++;
item2 = list2.GetNextItem();
break;
}
if (counter1 <= length1)
result.PutItem(item1);
for (counter1; counter1 < length1; counter1++)
{
item1 = list1.GetNextItem();
result.PutItem(item1);
}
if (counter2 <= length2)
result.PutItem(item2);
for (counter2; counter2 < length2; counter2++)
{
result.PutItem(item2);
item2 = list2.GetNextItem();
}
}
void SortedType::SplitLists(ItemType item, SortedType& list1, SortedType&
list2)
{
int counter = 0;
list1.MakeEmpty();
list2.MakeEmpty();
while (info[counter].ComparedTo(item) == LESS
||info[counter].ComparedTo(item) == EQUAL)
{
list1.PutItem(info[counter]);
counter++;
}
for (counter; counter < length; counter++){
list2.PutItem(info[counter]);
}
}
void SplitLists(SortedType& list, ItemType item,
SortedType& list1, SortedType& list2)
{
int length;
ItemType tempItem;
int counter = 1;
bool inList1;
list1.MakeEmpty();
list2.MakeEmpty();
length = list.GetLength();
//list.ResetList();
tempItem = list.GetNextItem();
inList1 = (tempItem.ComparedTo(item) == LESS ||
tempItem.ComparedTo(item) == GREATER);
while (inList1 && counter <= length)
{
list1.PutItem(tempItem);
if (counter < length)
{
tempItem = list.GetNextItem();
inList1 = (tempItem.ComparedTo(item) == LESS ||
tempItem.ComparedTo(item) == GREATER);
counter++;
}
}
for (int count = counter; count <= length; count++)
{
list2.PutItem(tempItem);
if (count < length)
tempItem = list.GetNextItem();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
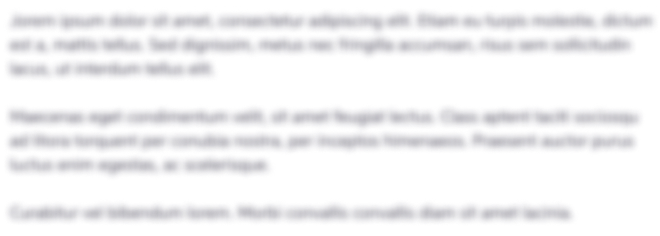
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started