Answered step by step
Verified Expert Solution
Question
1 Approved Answer
An alternative design for the LinkedStringLog class is to include an instance variable size that represents the number of Strings contained in the StringLog. What
An alternative design for the LinkedStringLog class is to include an instance variable size that represents the number of Strings contained in the StringLog. What changes need to be made to the LinkedStringLog implementation?
Create a new class LinkedStringLog2 to use this approach by inheriting all thats possible from LinkedStringLog and changing what needs to be changed. Test it.
public class LinkedStringLog implements StringLogInterface { protected LLStringNode log; // reference to first node of linked // list that holds the StringLog strings protected String name; // name of this StringLog public LinkedStringLog(String name) // Instantiates and returns a reference to an empty StringLog object // with name "name". { log = null; this.name = name; } public int insert(String element) // Precondition: This StringLog is not full. // // Places element into this StringLog. { LLStringNode newNode = new LLStringNode(element); newNode.setLink(log); log = newNode; return 0; } public boolean isFull() // Returns true if this StringLog is full, false otherwise. { return false; } public int size() // Returns the number of Strings in this StringLog. { int count = 0; LLStringNode node; node = log; while (node != null) { count++; node = node.getLink(); } return count; } public boolean contains(String element) // Returns true if element is in this StringLog, // otherwise returns false. // Ignores case difference when doing string comparison. { LLStringNode node; node = log; while (node != null) { if (element.equalsIgnoreCase(node.getInfo())) // if they match return true; else node = node.getLink(); } return false; } public void clear() // Makes this StringLog empty. { log = null; } public String getName() // Returns the name of this StringLog. { return name; } public String toString() // Returns a nicely formatted string representing this StringLog. { String logString = "Log: " + name + " "; LLStringNode node; node = log; int count = 0; while (node != null) { count++; logString = logString + count + ". " + node.getInfo() + " "; node = node.getLink(); } return logString; } // question 48 public boolean isEmpty() { LLStringNode node; node = log; if(node != null) { return false; } else return true; } //49 public int howMany(String element) { LLStringNode node; node = log; int count = 0; while (node != null) { if(element.equalsIgnoreCase(node.getInfo())) count++; log.getLink(); } return count; } //50 public boolean uniqueInsert(String element) { LLStringNode node; node = log; while (node != null) { if (element.equalsIgnoreCase(node.getInfo())) { return false; } node = node.getLink(); } insert(element); return true; }
test class:
public class UseStringLog { public static void main(String[] args) { StringLogInterface sample; sample = new LinkedStringLog("Example Use"); sample.insert("Elvis"); sample.insert("King Louis XII"); sample.insert("Yoda"); sample.insert("Captain Kirk"); System.out.println(sample); System.out.println("The size of the log is " + sample.size()); System.out.println("Elvis is in the log: " + sample.contains("Elvis")); System.out.println("Santa is in the log: " + sample.contains("Santa")); System.out.println(sample.isEmpty()); System.out.println(sample.uniqueInsert("Captain Kirk")); System.out.println(sample.first()); } }
Interface:
public interface StringLogInterface { int insert(String element); // Precondition: This StringLog is not full. // // Places element into this StringLog. boolean isFull(); // Returns true if this StringLog is full, otherwise returns false. int size(); // Returns the number of Strings in this StringLog. boolean contains(String element); // Returns true if element is in this StringLog, // otherwise returns false. // Ignores case differences when doing string comparison. void clear(); // Makes this StringLog empty. String getName(); // Returns the name of this StringLog. String toString(); // Returns a nicely formatted string representing this StringLog. boolean isEmpty(); //check is the list is empty int howMany(String element); //count how many of the element in the list boolean uniqueInsert(String element); //take in a unique insert String first(); //will get }
Here is the declaration: public class LLStringNode { private String info; private LLStringNode link; public LLStringNode(String info) { this.info = info; link = null; } public void setInfo(String info) // Sets info string of this LLStringNode. { this.info = info; } public String getInfo() // Returns info string of this LLStringNode. { return info; } public void setLink(LLStringNode link) // Sets link of this LLStringNode. { this.link = link; } public LLStringNode getLink() // Returns link of this LLStringNode. { return link; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
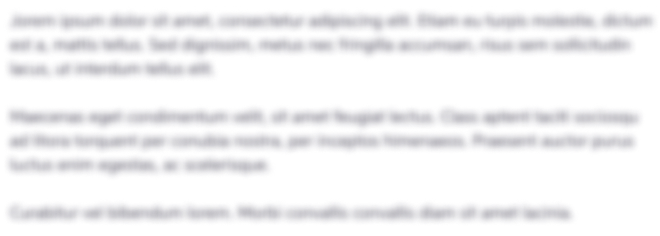
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started