Question
ANDROID STUDIO: I have created an app that mixes 3 colors. Each seekbar is assigned to Red, Green, and Blue Color values and the user
ANDROID STUDIO: I have created an app that mixes 3 colors. Each seekbar is assigned to Red, Green, and Blue Color values and the user can slide the seekabar to change the values (0 to 255) of each color. Also, I have created a new resource directory with main_menu with a resource file called main_menu.xml, which I created to apply the action bar. The action bar houses 4 buttons; setting, help, save color, and recall.
The question I have is how I implement:
- A save button:
That will allow the user to save the current color by name.
- A recall button:
That will allow the user to choose from previously saved colors. Once a color is selected, fill in the color and change the numberPickers to the appropriate values.
===========================================================
JAVA Main Activity:
=============================================================
package com.example.colorpicker; import android.graphics.Color; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.Menu; import android.widget.Button; import android.widget.SeekBar; import android.widget.TextView; public class MainActivity extends AppCompatActivity { Button buttonScreen; //displays the color TextView textViewR, textViewG, textViewB; // stores the value of seekbar SeekBar seekBarRed, seekBarGreen, seekBarBlue; //seekbars int redColor, blueColor, greenColor; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); redColor = 255; blueColor= 255; greenColor=255; buttonScreen=(Button)findViewById(R.id.buttonScreen); textViewR = (TextView) findViewById(R.id.textViewR); textViewG = (TextView) findViewById(R.id.textViewG); textViewB = (TextView) findViewById(R.id.textViewB); seekBarRed = (SeekBar)findViewById(R.id.seekBarRed); seekBarGreen = (SeekBar)findViewById(R.id.seekBarGreen); seekBarBlue = (SeekBar)findViewById(R.id.seekBarBlue); seekBarRed.setMax(redColor); seekBarRed.setProgress(redColor); seekBarGreen.setMax(greenColor); seekBarGreen.setProgress(greenColor); seekBarBlue.setMax(blueColor); seekBarBlue.setProgress(blueColor); buttonScreen.setBackgroundColor(Color.argb(255,redColor, greenColor, blueColor)); seekBarRed.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() { @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { redColor = progress; buttonScreen.setBackgroundColor(Color.argb(255,redColor, greenColor, blueColor)); textViewR.setText(String.valueOf(progress)); } @Override public void onStartTrackingTouch(SeekBar seekBar) { } @Override public void onStopTrackingTouch(SeekBar seekBar) { } }); seekBarGreen.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() { @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { greenColor = progress; buttonScreen.setBackgroundColor(Color.argb(255,redColor, greenColor, blueColor)); textViewG.setText(String.valueOf(progress)); } @Override public void onStartTrackingTouch(SeekBar seekBar) { } @Override public void onStopTrackingTouch(SeekBar seekBar) { } }); seekBarBlue.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() { @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { blueColor = progress; buttonScreen.setBackgroundColor(Color.argb(255,redColor, greenColor, blueColor)); textViewB.setText(String.valueOf(progress)); } @Override public void onStartTrackingTouch(SeekBar seekBar) { } @Override public void onStopTrackingTouch(SeekBar seekBar) { } }); } @Override public boolean onCreateOptionsMenu(Menu menu) { getMenuInflater().inflate(R.menu.main_menu,menu); return true; } }
===========================================================
XML Activity Main:
=============================================================
===============================================================
XML Main_menu: (ACTION BAR)
===============================================================
Step by Step Solution
There are 3 Steps involved in it
Step: 1
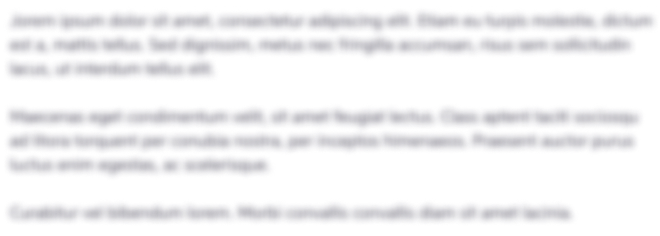
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started