Question
ANSWER ALL What is the total elapsed time for the two processes to run to completion?of a tie. Give the combined execution pattern of the
ANSWER ALL
What is the total elapsed time for the two processes to run to completion?of a tie. Give the combined execution pattern of the two processes in the format as in part (b), and determine the total elapsed time for the two processes to run to completion. [5 marks] (iii) Repeat part (b)(ii) with a pre-emptive scheduler operating with a time slice of 4 ms. [5 marks] (iv) What are the costs and the benefits of a pre-emptive over a non-preemptive scheduler for this workload, which would you choose, and why
) Modern operating systems typically support both threads and processesth concepts? [2 marks] (b) You get a summer job with a company which has an in-house operating system called sOs. sOs uses static priority scheduling, supports at most 32 concurrently-execut... Describe a common code structure in which programmers are likely to be able to define a static order between two lock types. [2 marks] (ii) Describe a common case in which programmers are likely to rely instead on dynamic discovery of an order between two lock types. [2 marks] (iii) Unlike the deadlock-detection algorithm presented in lecture, thes not remove edges when locks are released WITNESS is subject to false positives: warnings can be emitted due to legitimate cycles even though, by design, the cycle could never trigger an actual deadlock. Describe a situation in which this might arise, and explain why deadlock could never occur. [3 marks] (v) WITNESS, as written, is intended to be used with mutexes and other lock types providing mutual exclusion. A developer might na?vely extend WITNESS to support reader-writer locks (rwlock) by introducing graph edges for both read and write acquires as it does for mutex acquires. Explain why this might not always lead to the desired result. [3 marks]
Remote Procedure Call (RPC) allows procedures (functions, methods) to be forwarded over the network, and is a fundamental building block of distributed systems such as the Network File System (NFS). (a) Explain, with respect to a client, what it means for an RPC call to be: (i) Synchronous [1 mark] (ii) Asynchronous [1 mark] (iii) Idempotent [1 mark] (b) Distributed-filesystem clients utilize different tradeoffs between performance and consistency for operations on the directory namespace (e.g., file or directory creation) versus those on file data itself. The following program creates and opens a file foo, writes some data to it, and closes it, via NFSv3: fd = open("foo", O_CREAT | O_RDWR, 0755); // POINT A write(fd, data, sizeof(data)); // POINT B close(fd); // POINT C For each of points A, B, and C in the program, discuss whether or not another NFSv3 client is guaranteed to be able to see the results of each of open and (if it has been called) write. [5 marks] (c) The developers of a new distributed filesystem use a persistent log to ensure all-or-nothing retry semantics for filesystem operations. However, this comes at a substantial performance cost. Describe two circumstances under which filesystem RPCs can be excluded from the log while maintaining correctness, and give an example of each. [4 marks] (d) The distributed filesystem's persistent RPC log is maintained in a write-ahead log stored in local disk blocks. Explain why large RPCs may require special care in the server-side log implementation. [2 marks] (e) RPC protocols and write-ahead logging both rely on unique ID numbers. Explain why reusing client-generated RPC IDs for server log transaction IDs could harm each of correctness, scalability, and security. [6 marks]
(a) immutable Java class Complex that represents a complex number with integer real and imaginary components. [Note: Your class should contain only methods that are essential for its use. Do not incorporate any mathematical operations in the class.] [4 marks] (b) Without using Generics, adapt the class to support the use of arbitrary types to store the real and imaginary components. Give three disadvantages of this approach and comment on the immutability of the new class. [7 marks] (c) class from part (b) using Generics. Discuss the extent to which this new version addresses the problems identified in part (b). [7 marks] (d) Explain carefully why the following code cannot be used to print out an object of type LinkedList>. You may assume the existence of a working print method within each Complex object. void printAll(LinkedList> list) { for (Complex c : list) c.print();
Cons cumulative reward, a policy, and an optimal policy for a problem of this kind. [5 marks] (b) Give a detailed derivation of the Q-learning algorithm. [5 marks] (c) In the reinforcement learning problem shown in the diagram, states are positions on a grid and actions are down and right. The initial state is s1. The only way an agent can receive a (non-zero) reward is by moving into one of two special positions, one of which has reward ?50 and the other 150. s1 s2 s3 s4 s5 s6 s7 s8 s9 s10 s11 sossible sequence of actions (sequence 1) is shown by solid arrows, and another (sequence 2) by dashed arrows. Assume that all Q values are initialised at 0. Explain how the Q values are modified by the Q-learning algorithm if sequence 1 is used once, followed by two uses of sequence 2, and then one final use of sequence 1. [10 marks]
The following code has been written by a novice Java programmer. You are not required either to understand or to debug the details of how this code draws some particular pattern (a "Dragon"). The programmer finds that the Java compiler complains. Identify any errors and comment on any issues that (even if not strictly invalid Java) are liable to cause problems. You are not required to provide corrections.pper class Dragon extends JApplet throws Exception { import javax.swing.; public paint(Graphics g) { this.g = g; drawDragon(DRAWDEPTH,100,200,300,200); } /* @title: drawDragon Function to draw a dragon curve between too points
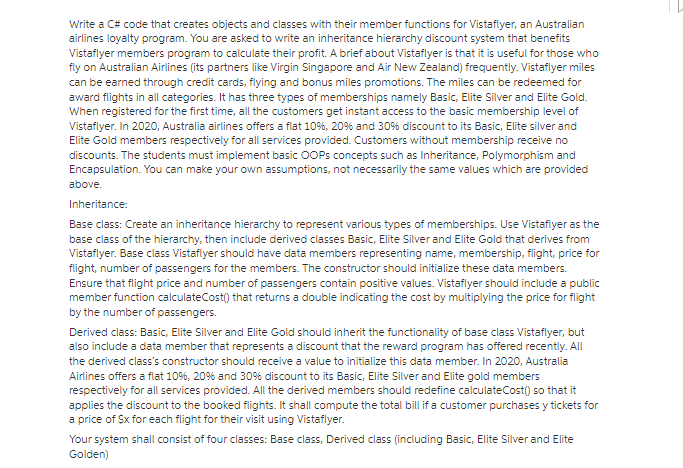
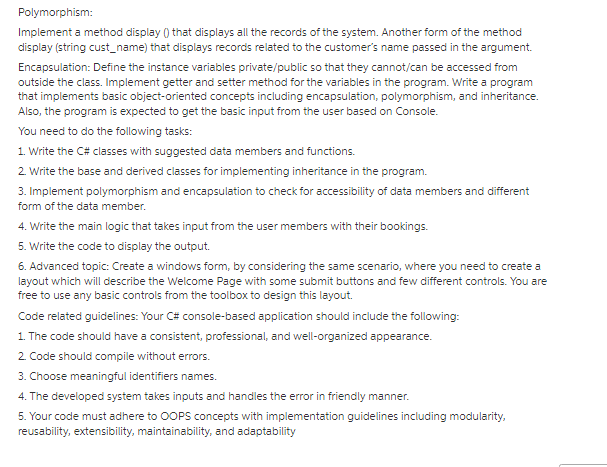
Write a C# code that creates objects and classes with their member functions for Vistaflyer, an Australian airlines loyalty program. You are asked to write an inheritance hierarchy discount system that benefits Vistaflyer members program to calculate their profit. A brief about Vistaflyer is that it is useful for those who fly on Australian Airlines (its partners like Virgin Singapore and Air New Zealand) frequently. Vistaflyer miles can be earned through credit cards, flying and bonus miles promotions. The miles can be redeemed for award flights in all categories. It has three types of memberships namely Basic, Elite Silver and Elite Gold. When registered for the first time, all the customers get instant access to the basic membership level of Vistaflyer. In 2020, Australia airlines offers a flat 10%, 20% and 30% discount to its Basic, Elite silver and Elite Gold members respectively for all services provided. Customers without membership receive no discounts. The students must implement basic OOPs concepts such as Inheritance, Polymorphism and Encapsulation. You can make your own assumptions, not necessarily the same values which are provided above. Inheritance: Base class: Create an inheritance hierarchy to represent various types of memberships. Use Vistaflyer as the base class of the hierarchy, then include derived classes Basic, Elite Silver and Elite Gold that derives from Vistaflyer. Base class Vistaflyer should have data members representing name, membership, flight, price for flight, number of passengers for the members. The constructor should initialize these data members. Ensure that flight price and number of passengers contain positive values. Vistaflyer should include a public member function calculateCost() that returns a double indicating the cost by multiplying the price for flight by the number of passengers. Derived class: Basic, Elite Silver and Elite Gold should inherit the functionality of base class Vistaflyer, but also include a data member that represents a discount that the reward program has offered recently. All the derived class's constructor should receive a value to initialize this data member. In 2020, Australia Airlines offers a flat 10%, 20% and 30% discount to its Basic, Elite Silver and Elite gold members respectively for all services provided. All the derived members should redefine calculateCost() so that it applies the discount to the booked flights. It shall compute the total bill if a customer purchases y tickets for a price of $x for each flight for their visit using Vistaflyer. Your system shall consist of four classes: Base class, Derived class (including Basic, Elite Silver and Elite Golden)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
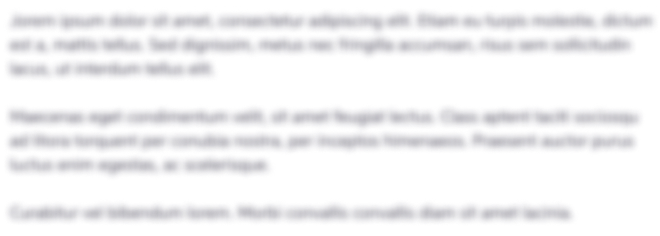
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started