Question
Answer only in JAVA language MyStack Class: importjava.util.ArrayList; public classMyStack { public final ArrayList list = new ArrayList (); public
Answer only in JAVA language
MyStack Class:
import java.util.ArrayList;
public class MyStack
public final ArrayList
public int getSize(){
return list.size();
}
public E peek() {
return list.get(getSize()-1);
}
public void push(E o) {
list.add(o);
}
public E pop() {
E o = list.get(getSize()-1);
list.remove(getSize()-1);
return o;
}
public boolean isEmpty() {
return list.isEmpty();
}
public String toString() {
return "stack:" + list;
}
}
Implement a class, call it Expr, which will be used for transforming the expressions (infix to postfix) and evaluate postfix.
Write a method
public static String infixToPostfix(String infix){
// Write appropriate codes here
// Return postfix as a returned String of this method
}
Use your MyStack to write the procedure of transformation from infix to postfix. Use the algorithm of infix to postfix translation which is introduced in the lecture, translate infix expression to postfix expression.
Assumption:
Input infix string doesn't have whitespaces.
All input number is one-digit number (positive digit 0 to 9)
Input infix can include parenthesis ( )
Operator + - * / ^ can be used
The output postfix string doesn't have whitespaces.
In the Expr class, implement another method, call it postfixEval, which prompts the user to enter a string value of postfix expression. Using class MyStack, the program should evaluate the postfix expression and return the correct calculated result.
Write a method
public static double postfixEval(String postfix){
// Write appropriate codes here
// Return calculated result value
}
Assumption:
Input postfix string doesn't have whitespaces.
All input number is one-digit number (positive digit 0 to 9)
Input postfix can include parenthesis ( )
Operator + - * / ^ can be used.
In the Expr class, write a main method which tests above two methods
Write a method
public static void main(String[] args){
// What is the process of main method.
// - User will insert infix
// - Invoke infixToPostfix to transform it to postfix expression
// - Invoke postfixEval to evaluate postfix expression
// - Show the calculated result
// Write appropriate codes here
// Allow the user re-run the program
}
// Examples
Enter an infix: (7+3)*(3-1)/2 //Red characters are user input
Postfix Expression: 73+31-*2/
Result value: 10.0
Do you want to continue? (Y/N) Y
Enter an infix: (5-6)/4*2-(5+2)
Postfix Expression: 56-4/2*52+-
Result value: -7.5
Do you want to continue? (Y/N) Y
Enter an infix: 3+2^(2+3)
Postfix Expression: 3223+^+
Result value: 35.0
Do you want to continue? (Y/N) N
Step by Step Solution
3.38 Rating (148 Votes )
There are 3 Steps involved in it
Step: 1
import javautilScanner public class Expr public static void mainString args Scanner scanner new Scan...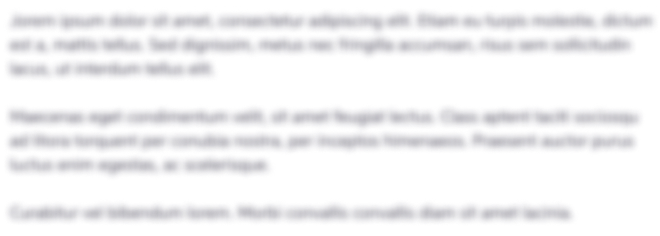
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started