Answered step by step
Verified Expert Solution
Question
1 Approved Answer
any help would be appreciated, thank you! Write a MIPS program to remove a given element from an array. Below is an example of the
any help would be appreciated, thank you! Write a MIPS program to remove a given element from an array. Below is an example of the C code segment to perform the above task. You may use a different code, but you have to write the code that you use as a comment before the MIPS code. Declaration of variables int A; Base address of array A int n; Length of the array A int val; Given value to be removed from array Remove elements equal to val int i ; while i n if Ai val If no match, proceed with next element i; else for int k i; k n ; k Shift elements if a match is found Ak Ak; n n ; if element deleted, length of array decreases Registers Variables $s A $s n $s val Addresses Contents $s A $s A $s A $sn An You may use any temporary registers from $t to $t or saved registers from $s to $s Clearly specify your choice of registers and explain your code using comments. Example Test: If the values of $s through $s and array elements are initialized in the simulator as: Use the button under the Registers display to initialize register values for $s $s $s and the button under the Memory display to initialize the initial array elements. Registers Data $s $s $s Addresses Contents The resultant registers are: Registers Data $s $s $s The resultant array is: Addresses Contents Note: Only the registers $s $s $s and the first $s elements in memory will be checked by the automated tests. This is what i have so far, but it wont compile: # Change the C code here if you have used something different from the one given. #int i ; #while i n # if Ai val If no match, proceed with next element # i; # else # for int k i; k n ; k Shift elements if a match is found # Ak Ak; # n n ; if element deleted, length of array decreases # # # Enter your MIPS code below. # Write short comments explaining # each line of your code # Explain your use of labels # MIPS code to remove a given element from an array # Register usage: # $s base address of array A # $s length of array A n # $s value to be removed from array val # $t index i # $t inner loop index k # $t temporary value for comparison # $t $t $t temporary registers for calculations # Initialize index i to addi $t $zero, # i loop: # Check if i n bge $t $s endloop # if i n exit loop # Load Ai into $t sll $t $t # t i calculate offset add $t $s $t # t baseaddress offset address of Ai lw $t$t # t Ai # Compare Ai with val bne $t $s next # if Ai val, skip to next iteration # If Ai val, shift elements to the left add $t $t # k i shiftloop: # Check if k n bge $t $s updatelength # if k n exit shift loop # Load Ak into $t sll $t $t # t k calculate offset add $t $s $t # t baseaddress offset address of Ak lw $t$t # t Ak # Store Ak at Ak sub $t $t # t k sll $t $t # tkcalculate offset add $t $s $t # t baseaddress offset address of Ak sw $t$t # Ak Ak # Increment k addi $t $t # k k j shiftloop # repeat shift loop updatelength: # Decrease the length of the array addi $s $s # n n # Do not increment i since elements are shifted j loop # repeat main loop next: # Increment i addi $t $t # i i j loop # repeat main loop endloop: # End of program, infinite loop to terminate j endloop # stay in end loop
any help would be appreciated, thank you!
Write a MIPS program to remove a given element from an array.
Below is an example of the C code segment to perform the above task. You may use a different code, but you have to write the code that you use as a comment before the MIPS code.
Declaration of variables
int A; Base address of array A
int n; Length of the array A
int val; Given value to be removed from array
Remove elements equal to val
int i ;
while i n
if Ai val If no match, proceed with next element
i;
else
for int k i; k n ; k Shift elements if a match is found
Ak Ak;
n n ; if element deleted, length of array decreases
Registers Variables
$s A
$s n
$s val
Addresses Contents
$s A
$s A
$s A
$sn An
You may use any temporary registers from $t to $t or saved registers from $s to $s Clearly specify your choice of registers and explain your code using comments.
Example Test: If the values of $s through $s and array elements are initialized in the simulator as:
Use the button under the Registers display to initialize register values for $s $s $s and the button under the Memory display to initialize the initial array elements.
Registers Data
$s
$s
$s
Addresses Contents
The resultant registers are:
Registers Data
$s
$s
$s
The resultant array is:
Addresses Contents
Note: Only the registers $s $s $s and the first $s elements in memory will be checked by the automated tests.
This is what i have so far, but it wont compile:
# Change the C code here if you have used something different from the one given.
#int i ;
#while i n
# if Ai val If no match, proceed with next element
# i;
# else
# for int k i; k n ; k Shift elements if a match is found
# Ak Ak;
# n n ; if element deleted, length of array decreases
#
#
# Enter your MIPS code below.
# Write short comments explaining
# each line of your code
# Explain your use of labels
# MIPS code to remove a given element from an array
# Register usage:
# $s base address of array A
# $s length of array A n
# $s value to be removed from array val
# $t index i
# $t inner loop index k
# $t temporary value for comparison
# $t $t $t temporary registers for calculations
# Initialize index i to
addi $t $zero, # i
loop:
# Check if i n
bge $t $s endloop # if i n exit loop
# Load Ai into $t
sll $t $t # t i calculate offset
add $t $s $t # t baseaddress offset address of Ai
lw $t$t # t Ai
# Compare Ai with val
bne $t $s next # if Ai val, skip to next iteration
# If Ai val, shift elements to the left
add $t $t # k i
shiftloop:
# Check if k n
bge $t $s updatelength # if k n exit shift loop
# Load Ak into $t
sll $t $t # t k calculate offset
add $t $s $t # t baseaddress offset address of Ak
lw $t$t # t Ak
# Store Ak at Ak
sub $t $t # t k
sll $t $t # tkcalculate offset
add $t $s $t # t baseaddress offset address of Ak
sw $t$t # Ak Ak
# Increment k
addi $t $t # k k
j shiftloop # repeat shift loop
updatelength:
# Decrease the length of the array
addi $s $s # n n
# Do not increment i since elements are shifted
j loop # repeat main loop
next:
# Increment i
addi $t $t # i i
j loop # repeat main loop
endloop:
# End of program, infinite loop to terminate
j endloop # stay in end loop
Step by Step Solution
There are 3 Steps involved in it
Step: 1
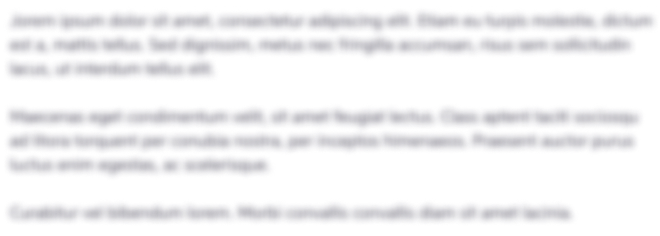
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started