Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Anyone could helo me how to developed this app (ATM) Using C# .Net framework? Automated Teller Machine (ATM) In this assignment you'll be implementing a
Anyone could helo me how to developed this app (ATM) Using C# .Net framework?
Automated Teller Machine (ATM) In this assignment you'll be implementing a simple ATM. You'll be writing six classes: 1. Account - Objects of this class represent a customer's bank account 2. ChequingAccount - It is a type of Account used for everyday banking 3. SavingAccount - it is a type of Account used for Saving purposes 4. Transaction - It is used to store the details of a Transaction. 5. AccountList - Object of this class is used to manage bank accounts 6. ATM This class creates an object of the AccountList class and provides and provides a menu to perform transactions, similar to an automated teller machine. This class contains the Main method. Account Class The Account class keeps track of customer information like their name and bank balance, and can perform transactions like depositing or withdrawing money from the account. Additionally, the Account class should include the following fields and properties: balance: A private field to represent the account's balance (double) AccountNumber: A read-only property to represent the customer's account number (int) AccountHolderName: A read-only property to represent the name of the customer (string) Balance: A read-only property to get the account balance (double) AnnualInterestRate: A property to get or set the Percent interest that the account earns per year (double) Unique account numbers must be generated: In the Account class declare a private static field named s_nextAccountNumber Add a static constructor and initialize it to 100 In the regular constructor initialize the ID field to s_nextAccount Number Increment the value of s_nextAccountNumber The Account class should have the following methods: A one-argument constructor that creates a bank account with the customer name A one-argument method named Deposit that deposits the specified amount to the account (adds to the balance) A one-argument method named Withdraw that withdraws the specified amount from the account (subtracts from the balance) ChequingAccount Class This class must extend the Account class and has an overdraft limit of $1000: A customer can withdraw an amount of money from the account, but only if their account total after the withdrawal won't exceed the overdraft amount. For example, if the balance is $100 and the overdraft is $1000, the customer can withdraw no more than $1100. The customer can also deposit an amount of money to the account. You must declare the overdraft amount as a constant field variable in this class. You also need to override the Withdraw method to restrict the withdrawal. The maximum annual interest rate allowed for this account is 1%. Hint: Use exceptions in the AnnualInterestRate Property to throw a new ArgumentOutOfRangeException and show a proper message. Hint: All Exceptions must be handled in the Main method of the ATM class. SavingAccount Class This class must extend the Account class but cannot be overdrawn. Any amount of money can be deposited. Override the Withdraw method to implement this limitation. SavingAccount Class This class must extend the Account class but cannot be overdrawn. Any amount of money can be deposited. Override the Withdraw method to implement this limitation. The minimum annual interest rate allowed for this account is 3%. Hint: Use exceptions in the AnnualInterestRate Property to throw a new ArgumentOutOfRangeException and show a proper message. Hint: All Exceptions must be handled in the Main method of the ATM class. Transaction Class This class should include read-only properties for the following information: Transaction Type (Widthraw/Deposit) (enum type) Amount (double) Date (Date Time) Use an enumeration type to represent the Transaction Type and restrict its values to Withdraw and Deposit. In another method of the ATM class, display the main menu. This method is called from main and should be named 'Menu'. The Menu method allows the user to perform transactions: a. Account Info (Shows the customer's name, balance, and the interest earned on different lines) b. Deposit C. Withdraw d. Transactions e. Go back to the Main Menu Page 3 of 4 You will pass an account object to the menu method (the account the user selected). You should declare the menu method like this: public void Menu (Account acct). Notice that this method accepts one parameter, the account object the user selected by entering an Account number. To display the list of transactions on an Account you must update your Account class to store the List of Transactions. You must update the Deposit and Withdraw methods to add a Transaction to the Transaction List. Automated Teller Machine (ATM) In this assignment you'll be implementing a simple ATM. You'll be writing six classes: 1. Account - Objects of this class represent a customer's bank account 2. ChequingAccount - It is a type of Account used for everyday banking 3. SavingAccount - it is a type of Account used for Saving purposes 4. Transaction - It is used to store the details of a Transaction. 5. AccountList - Object of this class is used to manage bank accounts 6. ATM This class creates an object of the AccountList class and provides and provides a menu to perform transactions, similar to an automated teller machine. This class contains the Main method. Account Class The Account class keeps track of customer information like their name and bank balance, and can perform transactions like depositing or withdrawing money from the account. Additionally, the Account class should include the following fields and properties: balance: A private field to represent the account's balance (double) AccountNumber: A read-only property to represent the customer's account number (int) AccountHolderName: A read-only property to represent the name of the customer (string) Balance: A read-only property to get the account balance (double) AnnualInterestRate: A property to get or set the Percent interest that the account earns per year (double) Unique account numbers must be generated: In the Account class declare a private static field named s_nextAccountNumber Add a static constructor and initialize it to 100 In the regular constructor initialize the ID field to s_nextAccount Number Increment the value of s_nextAccountNumber The Account class should have the following methods: A one-argument constructor that creates a bank account with the customer name A one-argument method named Deposit that deposits the specified amount to the account (adds to the balance) A one-argument method named Withdraw that withdraws the specified amount from the account (subtracts from the balance) ChequingAccount Class This class must extend the Account class and has an overdraft limit of $1000: A customer can withdraw an amount of money from the account, but only if their account total after the withdrawal won't exceed the overdraft amount. For example, if the balance is $100 and the overdraft is $1000, the customer can withdraw no more than $1100. The customer can also deposit an amount of money to the account. You must declare the overdraft amount as a constant field variable in this class. You also need to override the Withdraw method to restrict the withdrawal. The maximum annual interest rate allowed for this account is 1%. Hint: Use exceptions in the AnnualInterestRate Property to throw a new ArgumentOutOfRangeException and show a proper message. Hint: All Exceptions must be handled in the Main method of the ATM class. SavingAccount Class This class must extend the Account class but cannot be overdrawn. Any amount of money can be deposited. Override the Withdraw method to implement this limitation. SavingAccount Class This class must extend the Account class but cannot be overdrawn. Any amount of money can be deposited. Override the Withdraw method to implement this limitation. The minimum annual interest rate allowed for this account is 3%. Hint: Use exceptions in the AnnualInterestRate Property to throw a new ArgumentOutOfRangeException and show a proper message. Hint: All Exceptions must be handled in the Main method of the ATM class. Transaction Class This class should include read-only properties for the following information: Transaction Type (Widthraw/Deposit) (enum type) Amount (double) Date (Date Time) Use an enumeration type to represent the Transaction Type and restrict its values to Withdraw and Deposit. In another method of the ATM class, display the main menu. This method is called from main and should be named 'Menu'. The Menu method allows the user to perform transactions: a. Account Info (Shows the customer's name, balance, and the interest earned on different lines) b. Deposit C. Withdraw d. Transactions e. Go back to the Main Menu Page 3 of 4 You will pass an account object to the menu method (the account the user selected). You should declare the menu method like this: public void Menu (Account acct). Notice that this method accepts one parameter, the account object the user selected by entering an Account number. To display the list of transactions on an Account you must update your Account class to store the List of Transactions. You must update the Deposit and Withdraw methods to add a Transaction to the Transaction ListStep by Step Solution
There are 3 Steps involved in it
Step: 1
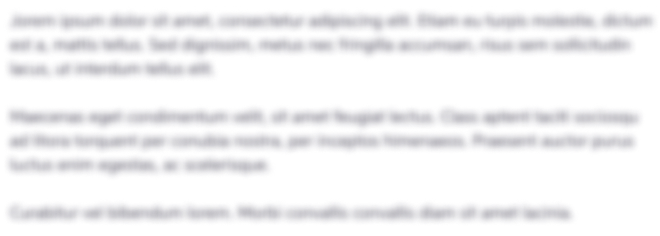
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started