Question
Application #3 Using the Queue interface and its implementation ArrayDeque class from java.util write a program that simulates a train route. A train route consists
Application #3
Using the Queue interface and its implementation ArrayDeque class from java.util write a program that simulates a train route.
A train route consists of a number of stations (assume 10, represented by the variable STATIONS), starting with station 0, and ending with a FINAL_STATION (value of STATIONS 1). The time that the train needs to travel between a pair of consecutive stations on the route is randomly generated (number between 5 and 9, represented by the variables MIN_TIME_TO_NEXT_STATION and MAX_TIME_TO_NEXT_STATION).
Associated with each station is a queue of passengers. Random number of passengers (between 0 and 10, see variable MAX_NUM_OF_PASSENGERS) is generated at each tick of the simulation clock. Each passenger is given a random entry station, and a random destination station (the entry station is always smaller than the destination station).
Trains leave a station at regular intervals (represented by the variable TRAIN_INTERVAL) and visit the stations on the route. When a train stops at a station, all passengers for that station exit first. Then any passenger waiting in the queue at the station boards the train until either the queue is empty, or the train is full.
Once the simulation is completed the final report is printed giving the statistics from the simulation.
TrainSimulation.java is the class that you will need to finish:
Follow the steps described in the file:Step#1 implement generateStations() method.
Run your application.
OUTCOME: 10 stations should be created with valid times to next station
Step #2 implement generatePassengers() method.
Run your application.
OUTCOME: At every time marker, between 0 and 9 passengers should be created with valid start and destination stations. Number of waiting passengers should be changed accordingly. Number of passengers on trains should be 0.
Step#3 implement startNewTrain() method.
Run your application.
OUTCOME: At every TRAIN_INTERVAL marker a new train should be created
Step #4 implement moveTrains() method.
Run your application
OUTCOME: At every time marker you should see trains unloading and loading the appropriate number of passengers. If a train reaches the final station it should no longer be in the queue. Your produced output should be similar to the sample run below.
public class TrainSimulation { // an array that will hold all stations private Station[] allStations; // a queue that will hold all passengers, so we can print statistics // when the simulation is over private QueueallPassengers; // a queue that will hold all trains private Queue allTrains; // keeps track of the number of trains en route private int trainCount; // total number of passengers created private int passengersCreated; // total number of passengers on the trains private int passengersOnTrains; // total number of passengers off the trains private int passengersDelivered; // number of stations for the simulation private final int STATIONS = 10; private final int FINAL_STATION = STATIONS - 1; // frequency of trains departing from the station 0 private final int TRAIN_INTERVAL = 5; // max number of passengers per train private final int TRAIN_CAPACITY = 20; // simulation time private final int DURATION = 50; // time range between two stations private final int MIN_TIME_TO_NEXT_STATION = 5; private final int MAX_TIME_TO_NEXT_STATION = 9; // max number of passengers to be randomly generated in one simulation tick private final int MAX_NUM_OF_PASSENGERS = 10; public Random generator; public TrainSimulation() { // create an array that will hold all stations this.allStations = new Station[STATIONS]; // create a queue that will hold all trains this.allTrains = new ArrayDeque<>(); // create a queue that will hold all passengers this.allPassengers = new ArrayDeque<>(); // initialize counters this.trainCount = 0; this.passengersCreated = 0; this.passengersOnTrains = 0; this.passengersDelivered = 0; // create Random object to be used for generating random values this.generator = new Random(); // generate all stations generateStations(); } public void generateStations() { // TODO Project 3 - Step #1 // fill the allStation array with Station objects where the value // of "time to next station" is randomly generated. // for each created station print the station's "time to next" } public void startNewTrain(int clock) { if ((clock % TRAIN_INTERVAL) == 0) { // TODO Project 3 - Step #3 // create new train object and add it to the allTrains queue // print the new train object // increment the train count by 1 } } public void generatePassengers(int clock) { // randomly generate number of new passengers int newPassengers = this.generator.nextInt(MAX_NUM_OF_PASSENGERS + 1); // TODO Project 3 - Step #2 // create the calculated number of passenger objects. For each new passenger // randomly generate the destination station and the start station. Remember // that the start station must be smaller than the destination station // add each passenger to the allPassengers queue and increment the number // of passengers created - you will need this information for the statistics // add each passenger to its appropriate start station - use allStations array } public void moveTrains(int clock) { System.out.println(" >> Moving each train <<"); int trainsToCheck = this.trainCount; for (int i = 0; i < trainsToCheck; i++) { // TODO Project 3 - Step #4 // 1. remove train from the allTrains queue // 2. move the train (see the Train class) // 3. if the train's time to the next station is 0 the train is at the station // a. unload the passengers from the train // b. load the waiting passengers on the train // c. update the passenger counters // d. if the train is at the final station // print the appropriate message and decrement the number of trains // otherwise update train's next station data: train.updateStation(allStations[stationNo].getTimeToNextStation()) // put the train back on the queue, and print the the train object // 4. if the train's time to the next station is not 0 the train is still in transit // so put it back to the queue and print the train object } } public void reportAtTimeMarker(int simulationTimer) { int passengersWaiting = this.passengersCreated - this.passengersOnTrains - this.passengersDelivered; System.out.println("-----At time marker " + simulationTimer + " -> passengers waiting: " + passengersWaiting + "\t on trains: " + this.passengersOnTrains + "\t active trains: " + this.trainCount + "-----"); System.out.println(); } public void finalSimulationReport(int clock) { DecimalFormat df = new DecimalFormat("#0.00"); System.out.println("***************** Final Report ***************"); System.out.println("The total number of passengers is " + this.passengersCreated); System.out.println("The number of passengers currently on a train " + this.passengersOnTrains); System.out.println("The number of passengers delivered is " + this.passengersDelivered); int passengersWaiting = this.passengersCreated - this.passengersOnTrains - this.passengersDelivered; System.out.println("The number of passengers waiting is " + passengersWaiting); int waitBoardedSum = 0; Passenger passenger; for (int i = 0; i < this.passengersCreated; i++) { passenger = this.allPassengers.poll(); if (passenger.boarded()) { waitBoardedSum += passenger.waitTime(clock); } } System.out.print("The average wait time for passengers that have boarded is "); System.out.println(df.format((double) waitBoardedSum / (this.passengersOnTrains + this.passengersDelivered))); } public static void main(String args[]) { System.out.println("************** TRAIN SIMULATION ************** "); TrainSimulation simulator = new TrainSimulation(); System.out.println("--> Starting the clock; duration set to " + simulator.DURATION + " "); for (int clock = 0; clock < simulator.DURATION; clock++) { simulator.generatePassengers(clock); simulator.startNewTrain(clock); simulator.moveTrains(clock); simulator.reportAtTimeMarker(clock); } simulator.finalSimulationReport(simulator.DURATION); } }
SAMPLE RUN with seed set to 101:
************** TRAIN SIMULATION **************
--> Creating 10 stations:
Station 0 has no passengers waiting; the time to next station is 5
Station 1 has no passengers waiting; the time to next station is 5
Station 2 has no passengers waiting; the time to next station is 8
Station 3 has no passengers waiting; the time to next station is 9
Station 4 has no passengers waiting; the time to next station is 5
Station 5 has no passengers waiting; the time to next station is 5
Station 6 has no passengers waiting; the time to next station is 9
Station 7 has no passengers waiting; the time to next station is 8
Station 8 has no passengers waiting; the time to next station is 7
Station 9 has no passengers waiting; the time to next station is 6
--> Starting the clock; duration set to 50
Passenger arrived at time marker 0 at station 3 heading to 4
Passenger arrived at time marker 0 at station 0 heading to 9
Passenger arrived at time marker 0 at station 3 heading to 5
Passenger arrived at time marker 0 at station 0 heading to 1
New train: Train 1 capacity of 20 arriving at station 0 in 1 minutes; currently 0 passengers on board
>> Moving each train <<
Train 1 is at station 0; unloaded 0; loaded 2 passengers; Space left 18
Train 1 capacity of 20 arriving at station 1 in 5 minutes; currently 2 passengers on board
-----At time marker 0 -> passengers waiting: 2 on trains: 2 active trains: 1-----
Passenger arrived at time marker 1 at station 0 heading to 8
Passenger arrived at time marker 1 at station 1 heading to 3
Passenger arrived at time marker 1 at station 3 heading to 4
Passenger arrived at time marker 1 at station 5 heading to 6
Passenger arrived at time marker 1 at station 0 heading to 3
Passenger arrived at time marker 1 at station 0 heading to 3
Passenger arrived at time marker 1 at station 7 heading to 8
>> Moving each train <<
Train 1 capacity of 20 arriving at station 1 in 4 minutes; currently 2 passengers on board
-----At time marker 1 -> passengers waiting: 9 on trains: 2 active trains: 1-----
Passenger arrived at time marker 2 at station 0 heading to 2
Passenger arrived at time marker 2 at station 0 heading to 1
Passenger arrived at time marker 2 at station 0 heading to 1
Passenger arrived at time marker 2 at station 8 heading to 9
>> Moving each train <<
Train 1 capacity of 20 arriving at station 1 in 3 minutes; currently 2 passengers on board
-----At time marker 2 -> passengers waiting: 13 on trains: 2 active trains: 1-----
Passenger arrived at time marker 3 at station 5 heading to 7
Passenger arrived at time marker 3 at station 1 heading to 6
Passenger arrived at time marker 3 at station 0 heading to 3
>> Moving each train <<
Train 1 capacity of 20 arriving at station 1 in 2 minutes; currently 2 passengers on board
-----At time marker 3 -> passengers waiting: 16 on trains: 2 active trains: 1-----
Passenger arrived at time marker 4 at station 1 heading to 7
Passenger arrived at time marker 4 at station 4 heading to 5
Passenger arrived at time marker 4 at station 4 heading to 7
.
.
.
.
.
.
.
.............
***************** Final Report ***************
The total number of passengers is 257
The number of passengers currently on a train 110
The number of passengers delivered is 121
The number of passengers waiting is 26
The average wait time for passengers that have boarded is 4.48
Process finished with exit code 0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
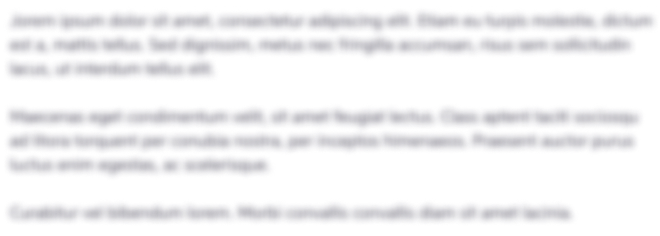
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started