Question
arith1.c code /* This is based on the Code in Figure 4.12, pages 105-107 from Kenneth C. Louden, Programming Languages Principles and Practice 2nd Edition
arith1.c code
/* This is based on the Code in Figure 4.12, pages 105-107 from Kenneth C. Louden, Programming Languages Principles and Practice 2nd Edition Copyright (C) Brooks-Cole/ITP, 2003 */ #include
char token; /* holds the current input character for the parse */
/* declarations to allow arbitrary recursion */ void command(void); int expr(void); int term(void); int factor(void); int number(void); int digit(void);
int isadigit(char c) { return (c >= '0' && c
void error(void) { printf("parse error "); exit(1); }
void getToken(void) { /* tokens are characters */ token = getchar(); if (token == '\t' || token ==' ') { getToken(); /* removes whitespaces on input line */ } }
void match(char c) { if (token == c) { getToken(); } else { error(); } }
void command(void) { int result = expr(); if (token == ' ') { printf("The result is: %d ",result); } else { error(); } }
int expr(void) { int result = term(); if (token == '+') { match('+'); result += expr(); } return result; }
int term(void) { int result = factor(); if (token == '*') { match('*'); result *= term(); } return result; }
int factor(void) { int result; if (token == '(') { match('('); result = expr(); match(')'); } else { result = number(); } return result; }
int number(void) { int result = digit(); while (isadigit(token)) { /* the value of a number with a new trailing digit is its previous value shifted by a decimal place plus the value of the new digit */ result = 10 * result + digit(); } return result; }
int digit(void) { int result = 0; if (isadigit(token)) { /* the numeric value of a digit character is the difference between its ascii value and the ascii value of the character '0' */ result = token - '0'; match(token); } else { error(); } return result; }
void parse(void) { getToken(); /* get the first token */ command(); /* call the parsing procedure for the start symbol */ }
int main() { printf("Type an arithmetic expression and hit enter: "); parse(); return 0; }
7) Examine arith1.c: It contains an implementation of a simple arithmatic calculator. The following will compile and run a few test cases. make arith1 ./arith1 1+2+3 ./arith1 1+23 The arith1 language is parsed used a recursive-descent parser: It is fairly straightforward to determine the language's grammar from the parser. a) Using the code, give the complete grammar in BNF form b) Is the grammar left or right recursive? How does that affect the associativity of plus and mult? c) Using the grammar you found in (a) add two new operators, divide, "/", and minus, "-" , operators to the arith1 language . The "-" should have the a higher precedence than "+" and lower precedence than "*". The divide operator, "/", should have the higher precedence than "*" but still be able to be overridden using parentheses as defined by the grammar. Both operators should have the same associativity as plus and times. Give the modified grammar. d) Modify arith1.c and add the new operator that you defined in (c). Pay careful attention to the pattern between the original grammar and the associated function calls in arith1.c. If you have properly adjusted the grammar, it should be a straightforward modification of the original functions with the additional of a couple new function for the new operator. Divide should round toward 0. You should pay careful attention to how expressions evaluate; a compile and a correct evaluation of some expressions does not mean you have modified everything correctly. Try the sample cases below. //arith1 1+22 The result is: 1 //arith1 235 The result is: 13 ./arith1 5/2 The result is: 2Step by Step Solution
There are 3 Steps involved in it
Step: 1
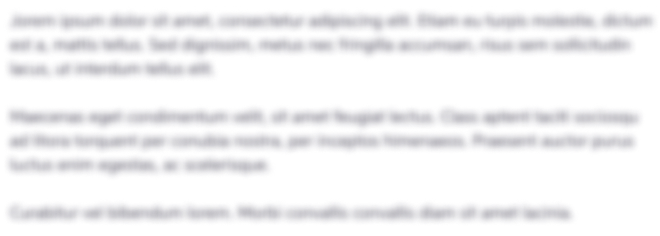
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started