Question
Assembly Language Programming with Linux Ubuntu For this lab you will be using a library cs12Lib.o And using basic math statements to write assembly code
Assembly Language Programming with Linux Ubuntu
For this lab you will be using a library
cs12Lib.o
And using basic math statements to write assembly code using integer arithmetic
The README.md file tells you how to use the library
The link to this online is https://github.com/stuart-srjc/CS12-
Labs/blob/master/cs12Lib/README.md
An example is given for each of the functions, this library may grow/change over time
Using the next two slides create a math project
You will want to create a project named
math
Create a fresh project
use your own name for projectName
Go to the CS12-Libs Directory
git pull cd template ./createProject projectName cd ../projectName make ./projectName
You should see CS12 print out
Blank project file You can use this for future projects as well
The createProject will do the following
Create a directory Create a basic .asm file Create a Makefile for this project
make will build the project make clean will clean up the files
Use pico to edit the .asm file
Add
-
Store the value 0x0a in rax
-
Print the register
-
Add 0x0a to rax
-
Print the register
-
Add 0x0a to rax again
-
Print the register
-
Your output should be what is shown on the right.
-
I will review the code for the add statements
CS12 ADD
0x000000000000000A
0x0000000000000014
0x000000000000001E
Add with carry
To add with carry store the value
0xFF in AL
-
Add 0x1 to al
-
Add with carry 0x1 to al
-
Print RAX
-
You should see the line below added to your output
0x0000000000000002
CS12
0xFF in AL
-
Add 0x1 to al
-
Add with carry 0x1 to al
-
Print RAX
-
You should see the line below added to your output
0x0000000000000002
CS12
ADD
0x000000000000000A
0x0000000000000014
0x000000000000001E
0x0000000000000002
Increment
Increment the rax register and
print it out
CS12
ADD
0x000000000000000A
0x0000000000000014
0x000000000000001E
0x0000000000000002
0x0000000000000003
Subtract
-
Add the SUB to the output
-
Subtract 0xa from the rax
-
register and print it
-
All previous data should print out as well as shown, in subsequent slides I will not be providing the previous data, but the last slide will hold the entire expected output.
CS12
ADD
0x000000000000000A
0x0000000000000014
0x000000000000001E
0x0000000000000002
0x0000000000000003
SUB
0xFFFFFFFFFFFFFFF9
Decrement
Decrement rax
Print rax
0xFFFFFFFFFFFFFFF8
Unsigned Multiplication
-
Output MUL
-
Set rax to 0x80
-
Multiply rax by 0xa (using rbx)
-
Print the register value for RDX
-
Print the register value for RAX
-
Now set rax to 0x8000000001
-
Multply rax by 0x8000000000
-
Print RDX And RAX Note values in both registers
MUL
0x0000000000000000
0x0000000000000500
0x0000000000004000
0x0000008000000000
Signed Multiplication
-
Set al to 0x8f
-
Use mul to multiply
al by 0x2 (using bl)
-
Print the register value for RAX
-
Repeat with imul
-
Note the difference between the singed and unsigned operations
MUL
0x0000000000000000
0x0000000000000500
0x0000000000004000
0x0000008000000000
0x000000000000011E
0x000000000000FF1E
Unsigned Division
Output DIV Set rax to 0xFFFA Set rdx to 0x0 Divide rdx:rax by 0xF (using rbx) Print the register value for RAX Print the register value for RDX Note values in both registers RAX is the quotient RDX is the remainder
DIV
0x0000000000001110
0x000000000000000A
Signed Division
Output DIV Set ax to -22 Divide ax by 0x5 (using bh) Print the register value for RAX Note: FE FC are ah:al FE = -2 remainder FC = -4 quotient
DIV
0x0000000000001110
0x000000000000000A
0x000000000000FEFC
What to turn in
-
Turn in a screen shot of your program run
-
Turn in the math.asm file that generates your output
-
Your output should match the output listed below
Step by Step Solution
There are 3 Steps involved in it
Step: 1
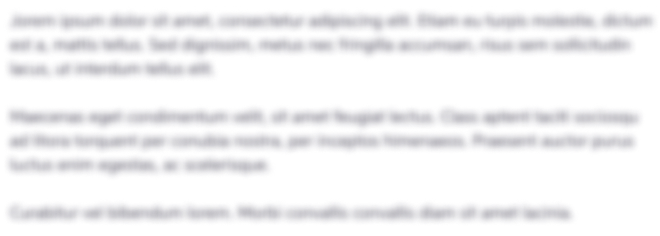
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started