Question
Assignment #1 Create a program for managing inventory data. The class name should be InvTracker, an underscore, and your NetID run together; for example, if
Assignment #1
Create a program for managing inventory data. The class name should be InvTracker, an underscore, and your NetID run together; for example, if your NetID is abcde6, your class name is InvTracker_abcde6. For this assignment, we will create the initial class definition with data members, method shells (just headers), and a second class, InvItem. The design requirements are:
1) InvTracker data members:
a) an array of type InvItem, with no specific size (statement: InvItem[] items;)
b) an int for the InvItem array size
2) InvTracker methods:
a) inStock: takes a long as a parameter, returns a boolean
b) stockAmount: takes a long as a parameter, returns an int
c) add: takes a long and an int as parameters, returns void
d) remove: takes a long and an int as parameters, returns void
e) delete: takes a long as a parameter, returns void
f) search: takes a String as a parameter, returns an array of type InvItem
(method header: InvItem[] search(String s))
3) The InvItem class should be placed after and outside the InvTracker class definition, as:
public class InvTracker { // code goes here} class InvItem {//more code goes here}
Note that only the InvTracker class should be public!
4) InvItem data members:
a) a long named id
b) a String named name
c) an int named quantity
5) InvItem methods:
a) get & set methods for id (resp. return or accept a long)
b) get & set methods for name (resp. return or accept a String)
c) get & set methods for quantity (resp. return or accept an int)
Additional Constraints
The program should be implemented as a single file and Java class. All data members should be declared as private; all methods should be declared without any visibility modifiers. Methods that return a value should return a placeholder value (like -1 or an empty String) to avoid appearing as code errors in Eclipse. Note that, for this assignment, the program wont actually run since it doesnt have a main method.
Assignment #2
1) InvTracker3 data members:
a) an array named items of type InvItem, with no specific size
b) an int named count for the InvItem array size
2) InvTracker3 methods:
a) inStock: takes a long as a parameter, returns a boolean. Returns true if an element in items has id equal to the parameter long and returns false otherwise.
b) stockAmount: takes a long as a parameter, returns an int. Returns the quantity attribute of the element in items with id equal to the parameter long and returns -1 otherwise.
c) add: takes a long and an int as parameters, returns void. If an element in items has id matching the parameter long, the parameter int will be added to its quantity attribute. Otherwise, a new InvItem will be created with the parameter long as its id and the parameter int as its quantity and count will be incremented.
d) remove: takes a long and an int as parameters, returns void. If an element in items has id matching the parameter long and has quantity equal to or greater than the parameter int, the parameter int will be subtracted from its quantity attribute.
e) delete: takes a long as a parameter, returns void. If an element in items has id matching the parameter long, that InvItem object will be deleted, any InvItems at subsequent indexes will be moved to one index lower, and count will be decremented.
f) search: takes a String as a parameter, returns an array of type InvItem. This method will create an InvItem array containing all InvItems from items whose name attributes contain the text of the parameter String. This does not require an exact match use Strings indexOf method.
g) a constructor method that takes no parameters, creates items as an array with size 1000, and sets count equal to zero.
h) a main method that creates a InvTracker3 object. If desired, you may also include a loop in the main method to accept and process user commands for testing your code. However, this is not required for the assignment.
3) The InvItem class should be placed after and outside the InvTracker3 class definition, as:
public class InvTracker3 { // code goes here} class InvItem {//more code goes here}
Note that only the InvTracker3 class should be public!
4) InvItem data members:
a) a long named id
b) a String named name
c) an int named quantity
5) InvItem methods:
a) get & set methods for id (resp. return or accept a long). Add code to read or write the id attribute.
b) get & set methods for name (resp. return or accept a String). Add code to read or write the name attribute.
c) get & set methods for quantity (resp. return or accept an int). Add code to read or write the quantity attribute.
d) a constructor method that takes a long as a parameter, creates an InvItem with id equal to the parameter long, name equal to an empty String, and quantity equal to 0.
Additional Constraints
The program should be implemented as a single file and Java class. All data members should be declared as private; all methods should be declared without any visibility modifiers.
Assignment #3
Create a program for managing inventory data. The class name should be InvTracker3, an underscore, and your NetID run together; for example, if your NetID is abcde6, your class name is InvTracker3_abcde6. For this assignment, we will extend our earlier code and provide a GUI. All prior code requirements remain in effect, with the updated class name.
The new design requirements are:
1) InvTracker3 should extend JFrame and implement the ActionListener interface. Each InvTracker3 object should have, in addition to the prior data members:
a) Five JPanels
b) Three JTextFields for displaying an InvItems id, name, and quantity
c) Five JButtons with labels Next, Previous, Update Qty, Load, and Save.
d) Arrangement: One JPanel should be on the content pane and the other four JPanels should be added to it using a GridLayout with 4 rows & 1 column. The first (upper) three JPanels should each have a JTextField and the last (bottom) JPanel should have the JButtons.
2) JTextField functionalities:
a) The id and name text fields will display the current InvItems id and name, respectively. These JTextFields should be read-only. Note that when the application is launched, there will be no data in the items array and so these will both be blank.
b) The quantity text field will display the quantity for the current InvItem. When the application is launched, the items array will be empty and this field will also be blank.
3) JButton functionalities:
a) Clicking the Load JButton will load a file InvData.dat which contains an array of InvItem objects and overwrites any existing InvTracker3.items array and updates the count value. The current InvItem will be the one at index zero and the JTextFields should display its data values.
b) Clicking the Save JButton will save the current items array as the file InvData.dat.
c) Clicking the Next JButton will display the id, name, and quantity for the next-higher index in the items array. If the current InvItem is the last one in the items array or if no items array currently exists, the click will do nothing.
d) Clicking the Previous JButton will display the id, name, and quantity for the next-lower index in the items array. If the current InvItem is the first one in the items array (index 0) or if no items array currently exists, the click will do nothing.
e) Clicking the Update Qty JButton will set the current items quantity to whatever value the user has entered in the quantity text field.
f) All JButtons should use the InvTracker3 object as the listener.
No error-checking is required for this assignment. You can assume users only enter valid inputs.
**PLEASE NOTE THAT I ONLY NEED HELP WITH ASSIGNMENT #3 - ASSIGNMENTS 1 & 2 ARE JUST FOR REFERENCE
Step by Step Solution
There are 3 Steps involved in it
Step: 1
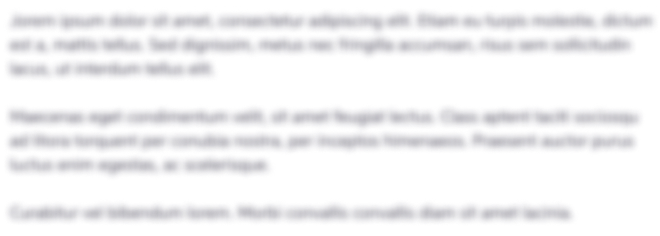
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started