Question
Assignment 2, Part 1 - Boxcar For this assignment, you will be writing a class called Boxcar that represents a single car on a freight
Assignment 2, Part 1 - Boxcar
For this assignment, you will be writing a class called Boxcar that represents a single car on a freight train. Download the template file, Boxcar.java (Links to an external site.), as a starting point. Your job is to add code to Boxcar.java so that your implementation meets the requirements specified below. To complete the assignment, replace all the /* missing code */ comments in the file with your own code. You do not need to change any other code in the file.
In previous assignments, we had a requirement that your class be named Main. In this assignment, the class is required to be named Boxcar.
When you are done writing your Boxcar.java code, you can test it by downloading the Student_Runner_Boxcar.java (Links to an external site.) file into the same directory and running the file. Check to see that your implementation output matches the sample run listed below. You may find that you wish to change some of the test cases in the runner file to more closely test the functionality of your code. We will test your code using our own runner file that is different from the one provided.
When you are done coding and testing, copy and paste your entire Boxcar class into the Code Runner and press "Submit" in order for your assignment to count as turned in.
Variables
- String cargo - Represents the type of cargo carried by the boxcar, with possible values of "gizmos", "gadgets", "widgets" and "wadgets". No other cargo types are allowed.
- int numUnits - Represents the number of units carried by the boxcar. Must be between 0 and 10 inclusive.
- boolean repair - Represents whether the boxcar is in repair or in service. Set to true if the boxcar is in repair. If the boxcar is in repair, numUnits must be 0.
Methods
- Boxcar() - Default constructor that sets the boxcar to gizmos, 5 units, and in service (not in repair).
- Boxcar(String c, int u, boolean r) - This constructor sets the cargo variable to the parameter c, but only if c is "gizmos", "gadgets", "widgets", or "wadgets". The constructor ignores the case of the value in c. If c holds any value other than "gizmos", "gadgets", "widgets", or "wadgets", the constructor sets cargo to "gizmos". The numUnits variable is set to the parameter u. If u is less than 0 or higher than the maximum capacity of 10 units, numUnits is set to 0. The repair variable is set to the parameter r. If repair is true, numUnits is set to 0 no matter what value is stored in the u parameter.
- String toString () - returns a String with the Boxcar in the format:
5 gizmos in service 10 widgets in service 0 gadgets in repair
Notice there is one space in between the number of units and the cargo and a tab between the value for cargo and "in repair"/"in service
- void loadCargo() - This method adds 1 to the number of units in the BoxCar. The maximum capacity of a boxcar is 10 units. If increasing the number of units would go beyond the maximum, keep numUnits at the max capacity. If the repair variable is true, then numUnits may only be set to 0.
- String getCargo() - This method returns the cargo type of the boxcar.
- void setCargo(String c) - The cargo variable is set to c only if c is "gizmos", "gadgets", "widgets", or "wadgets", ignoring case. The value stored for cargo should be lowercase. If c holds any value other than "gizmos", "gadgets", "widgets", or "wadgets" (ignoring case), the method sets the cargo to "gizmos".
- boolean isFull() - The isFull method returns true if numUnits is equal to 10, false otherwise.
- void callForRepair() - Sets repair to true. Recall that if the repair variable is true, then the numUnits variable may only be set to 0.
Sample Run
Testing Boxcar constructors: Printing Boxcar(): 5 gizmos in service Printing Boxcar("widgets", 7, false): 7 widgets in service Testing lowercase cargo and setting cargo to 0 if in repair. Printing Boxcar("WaDGeTs", 7, true): 0 wadgets in repair Testing cargo other than accepted values. Printing Boxcar("OtherStuff", 7, true): 7 gizmos in service Testing callForRepair: Printing Boxcar called for repair: 0 widgets in repair Printing Boxcar with 8 gadgets: 8 gadgets in service Printing Boxcar with 10 gadgets, tried to overload: 10 gadgets in service Printing Boxcar in repair, can't load (0 cargo): 0 widgets in repair Testing isFull: Printing isFull on full car: true Printing isFull on non-full car: false Testing getCargo: Printing getCargo on a "widgets" car: widgets Testing setCargo: Setting cargo to gadgets: 7 gadgets in service Testing lowercase conversion (WADGetS -> wadgets): 7 wadgets in service Testing invalid cargo type sets to gizmos (onions -> gizmos): 7 gizmos in service
********************************************************************
My Code:
import java.util.ArrayList; public class Boxcar { private String cargo; private int numUnits; private boolean repair; Boxcar() { cargo = "gizmos"; numUnits = 5; repair = false; } Boxcar(String c, int u, boolean r) { setCargo(c); if(r || u < 0 || u > 10) { numUnits = 0; } } public void loadCargo() { if (!repair && numUnits <= 10) { numUnits++; } if (numUnits > 10) { numUnits = 10; } if (repair) { numUnits = 0; } } public String getCargo(int u) { return cargo; } public void setCargo(String c) { if (c.equalsIgnoreCase("gizmos") || c.equals("gadgets") || c.equals("widgets") || c.equals("wadgets")) { cargo = c.toLowerCase(); } else { cargo = "gizmos"; } } public void callForRepair() { repair = true; loadCargo(); } public boolean isFull() { if (numUnits == 10) { return true; } else { return false; } } public String toString() { String output = ""; output += (numUnits + " " + cargo); if (repair) { output += ("\t" + "in repair"); } else { output += ("\t" + "in service"); } return output; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
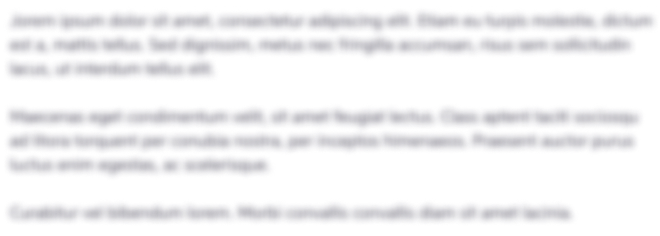
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started