Question
Assignment 9 - Changing Sort Keys Please Code in Python # beginning of class Student definition ------------------------- class Student: # class (static) attributes and intended
Assignment 9 - Changing Sort Keys
Please Code in Python
# beginning of class Student definition ------------------------- class Student: # class ("static") attributes and intended constants DEFAULT_NAME = "zz-error" DEFAULT_POINTS = 0 MAX_POINTS = 30
# initializer ("constructor") method ------------------- def __init__(self, last = DEFAULT_NAME, first = DEFAULT_NAME, points = DEFAULT_POINTS): # instance attributes if (not self.set_last_name(last)): self.last_name = Student.DEFAULT_NAME if (not self.set_first_name(first)): self.first_name = Student.DEFAULT_NAME if (not self.set_points(points)): self.total_points = Student.DEFAULT_POINTS
# mutator ("set") methods ------------------------------- def set_last_name(self, last): if not self.valid_string(last): return False # else self.last_name = last return True
def set_first_name(self, first): if not self.valid_string(first): return False # else self.first_name = first return True def set_points(self, points): if not self.valid_points(points): return False # else self.total_points = points return True # accessor ("get") methods ------------------------------- def get_last_name(self): return self.last_name
def get_first_name(self): return self.first_name def get_total_points(self): return self.total_points
# output method ---------------------------------------- def display(self, client_intro_str = "--- STUDENT DATA ---"): print( client_intro_str + str(self) )
# standard python stringizer ------------------------ def __str__(self): return self.to_string()
# instance helpers ------------------------------- def to_string(self, optional_title = " ---------- "): ret_str = ( (optional_title + " name: {}, {}" + " total points: {}."). format(self.last_name , self.first_name, self.total_points) ) return ret_str
# static/class methods ----------------------------------- @staticmethod def valid_string(test_str): if (len(test_str) > 0) and test_str[0].isalpha(): return True; return False
@classmethod def valid_points(cls, test_points): if 0
@classmethod def compare_two_students(cls, first_stud, second_stud): """ comparison done on parameters' last names """ return cls.compare_strings_ignore_case( first_stud.last_name, second_stud.last_name) @staticmethod def compare_strings_ignore_case(first_string, second_string): """ returns -1 if first second, and 0 if same this particular version based on last name only (case insensitive) """ fst_upper = first_string.upper() scnd_upper = second_string.upper() if fst_upper scnd_upper: return 1 # else return 0
# beginning of class StudentArrayUtilities definition --------------- class StudentArrayUtilities: @classmethod def print_array(cls, stud_array, optional_title = "--- The Students -----------: "): print( cls.to_string(stud_array, optional_title) )
@classmethod def array_sort(cls, data, array_size): for k in range(array_size): if not cls.float_largest_to_top(data, array_size - k): return
# class stringizers ---------------------------------- @staticmethod def to_string(stud_array, optional_title = "--- The Students -----------: "): ret_val = optional_title + " " for student in stud_array: ret_val = ret_val + str(student) + " " return ret_val @staticmethod def float_largest_to_top(data, array_size): changed = False # notice we stop at array_size - 2 because of expr. k + 1 in loop for k in range(array_size - 1): if Student.compare_two_students(data[k], data[k + 1]) > 0: data[k], data[k+1] = data[k + 1], data[k] changed = True return changed
# client --------------------------------------------
# instantiate some students, one with and illegal name ... my_students = \ [ Student("smith","fred", 95), Student("bauer","jack",123), Student("jacobs","carrie", 195), Student("renquist","abe",148), Student("3ackson","trevor", 108), Student("perry","fred",225), Student("lewis","frank", 44), Student("stollings","pamela",452) ] array_size = len(my_students)
StudentArrayUtilities.print_array(my_students, "Before: ") StudentArrayUtilities.array_sort(my_students, array_size) StudentArrayUtilities.print_array(my_students, "After: ")
""" ---------------------- RUN ------------------------ Before: ---------- name: smith, fred total points: 95. ---------- name: bauer, jack total points: 123. ---------- name: jacobs, carrie total points: 195. ---------- name: renquist, abe total points: 148. ---------- name: zz-error, trevor total points: 108. ---------- name: perry, fred total points: 225. ---------- name: lewis, frank total points: 44. ---------- name: stollings, pamela total points: 452. After: ---------- name: bauer, jack total points: 123. ---------- name: jacobs, carrie total points: 195. ---------- name: lewis, frank total points: 44. ---------- name: perry, fred total points: 225. ---------- name: renquist, abe total points: 148. ---------- name: smith, fred total points: 95. ---------- name: stollings, pamela total points: 452. ---------- name: zz-error, trevor total points: 108. ------------------------------------------------------------- """
LAB Assignment 9 - Changing Sort Keys Submit Assignment Due Wednesday by 2pm Points 20 Submitting a file upload File Types txt Available Feb 27 at 2pm - Mar 22 at 11:59pm 23 days Assignment 9 - Changing Sort Keys Make sure you have read and understood . both modules A and B this week, and module 2R-Lab Homework Requirements before submitting this assignment. Hand in only one program, please While it may appear long at first, this lab is easier than it looks, so stay calm. It is easier because 1. it uses material and code that you learned, compiled and ran over a week ago when you studied Module 8, and 2. below, I give you very explicit directions on what to do for each of the very short methods you need to write. You will start with my existing Student and StudentArrayUtilities classes that you have already compiled and run when you read the modules. Then you will modify each of these classes as described below and provide a new test client. Sort Flexibility (Student class) In week 8, we saw an example of a Student class that provided a static compare_strings_ignore_case) method. We needed to define such a method because our sort algorithm (which was in the StudentArrayUtilities (SAU) class) had to have a basis for comparing two Student objects, the foundation of SAUs sorting algorithm. Since a Student is a compound data type, not a float or a string, there was no pre-defined less-than.= 2 elements: find the two middle elements and return their average of their total points. o Odd-numbered arrays3 elements: return the total points of the exact middle element. Special Note: This method has to do the following. It must sort the array according to total points in order to get the medians, and that's easy since we already have the sort method. Then it has to find the middle-student's score (e.g., if the array is size 21, the middle element is the score in array 10], after the sort). But, before doing the sort, it also has to change the sort key of the Student class to SORT BY-POINTS. One detail, that you may not have thought of, is that, at the very start of the method, it needs to save the client's sort key. Then, before returning, restore the client's sort key. This method doesn't know what that sort key might be, but there is an accessor get sort key0 that will answer that question. o This method has the word "Destructive" in its name to remind the client that it may (and usually will) modify the order of the array, since it is going to sort the array by total points in the process of computing the median. However, it will not destroy or modify the client's sort key when the method returns to client (see previous bullet) o If the user passes in a bad arraySize 1) return a 0. The Main Program Our client will declare three Student arrays: using direct initialization, as in the modules: no user input. The array sizes should be 15, 16 and 1. The second array can be the same as the first with one extra Student tagged onto the end. Each array should be initialized in no particular order: unsorted in all felds. Using the largest, even numbered, array 1. display the array immediately before cling a sort method, 2. sort the array using the default (initial) sort key and display, 3. change the sort key to first name, sort and display 4. change the sort key to total score, sort and display, 5. set sort key) to first name, call the get median destructive0 method and display the median score. and finally 6. call get sort_key) to make sure that the get median_destructive0 method preserved the client's sort key value of first name that was just set prior to the get_median_destructive0 call. Using each of the two other arrays: .get the median of each array and display. No other testing needed in this part Here's a sample output, but you must not use my arrays. Make your own as per the spec above. Before default sort (even) name: smith, fred total points: 95 name: bauer, jack total points: 123 name: jacobs, carrie total points: 19!5 etc After default sort (even) name: bauer, jack total points: 123 name: cassar, john total points: 321 name: charters, rodney total points: 29!5 name: jacobs, carrie total points: 19!5 etc After sort BY FIRST After sort BY FIRST: name: renquist, abe total points: 148 name: jacobs, carrie total points: 19!5 name: loceff, fred total points: 44 name: perry, fred total points: 225 etc After sort BY POINTS: name: loceff, fred total points: 44 name: smith, fred total points: 95 name: zz-error, trevor total points: 108 name: bauer, jack total points: 123 etc Median of even class.. Successfully preserved sort key Median of odd class- Median of small classStep by Step Solution
There are 3 Steps involved in it
Step: 1
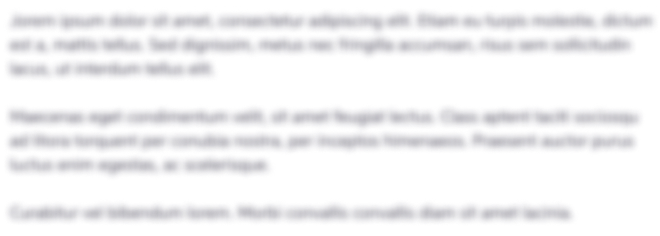
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started