Question
Assignment Background: Most calculators use infix notation, in which the operator is typed between the operands. Everyone is familiar with solving infix math expressions, such
Assignment Background:
Most calculators use infix notation, in which the operator is typed between the operands. Everyone is familiar with solving infix math expressions, such as 2 + 3, or 9 - 5.
There are two other types of notation: prefix and postfix. In prefix, the operator comes before ("pre") the operands, such as in these expressions: + 2 3 or - 9 5.
Postfix expressions are used on some specialized calculators called RPN calculators, so complex math expressions can be entered without the need for parentheses. Postfix math is also called reverse polish notation (RPN). RPN is defined as a mathematical expression in which the numbers precede the operation (i.e., 2 + 2 is 2 2 + in RPN, or 10 - 3 * 4 is 10 3 4 * - in RPN). Postfix statements look like 2 3 + or 9 5 -.
Here is a comparison of infix versus postfix math expressions:
infix postfix 5 + ((7 + 9) * 2) 5 7 9 + 2 * + A postfix expression is evaluated as follows:
- if a value is entered, it is placed on the stack - if an operation is entered (+, -, *, /), the stack is popped twice and the operation is applied to those two numbers. The resulting answer is placed back on the stack.
The expression 5 7 9 + 2 * + is solved in this order:
5 16 2 * + 5 32 + 37
Notice that postfix expressions are simply solved by moving from left to right and parentheses are not needed.
The following special cases must be addressed:
- if the problem to be solved is 7 - 5 (infix), the problem is entered on an RPN calculator in the following order.
7 (enter) 5 (enter) -
This causes the stack to be popped twice and the correct expression to be evaluated is:
7 5 -
The answer is 2.
A similar ordering issue surrounds the (/) operator:
If the problem to be solved is 9 / 2 (infix), the problem is entered on an RPN calculator as:
9 2 /
The answer is 4.
Assignment:
Write a program to implement a simple RPN calculator that processes only the following type of input:
single-digit integers the four integer math operations: +, -, *, /
The user will type in single character input until Q or q is entered. As described above, if a digit is entered, the number is placed on the stack. If an operation is entered, pop two values off the stack, do the math, then return the answer back onto the stack. As keyboard input is entered, the program should keep track of all keystrokes entered to be replayed after Q or q is entered.
When Q or q is entered, the program will print out the entire problem (i.e. the sequence of characters that had been input) and the answer. It is suggested that a queue and a stack should be used in this lab.
You must create a modular solution and have at least 2 helper methods beyond the calculate() method that you see in the starter code.
Instructions:
Here are 5 examples of the input and output:
9 5 - q 9 5 - = 4 // Note: This is the output that should be printed out
7 3 * 6 / Q // Note: either a 'q' or 'Q'... 7 3 * 6 / = 3
8 4 7 + - q 8 4 7 + - = -3
7 3 9 4 5 * + - - q 7 3 9 4 5 * + - - = 33
8 4 3 * * 6 4 2 - + + Q 8 4 3 * * 6 4 2 - + + = 104
Complete the following Java program -
import java.util.*; public class RPN { Scanner scan = new Scanner( System.in ); private Stack
/** * Constructs an RPN Calculator */ public RPN() { // TODO complete constructor } // **** Used for testing - Do Not Remove *** public RPN(String console) { this(); scan = new Scanner( console ); }
// TODO Write your method description here. public void calculate() { // TODO complete method }
// TODO: additional helper methods
// Instantiates an RPN Calculator and invokes it calculate method public static void main( String[] args ) { RPN rpn = new RPN(); rpn.calculate(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
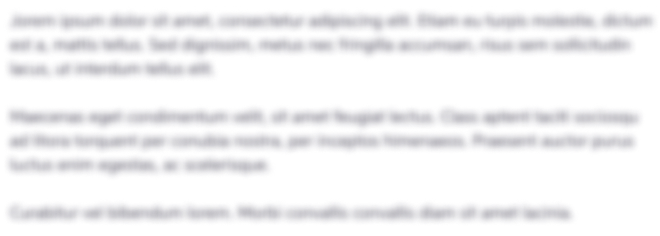
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started