Question
****Assignment Classes/Streams - Marketing software In this programming assignment, youll identify the number of potential customers for a business. The starter program outputs the number
****Assignment
Classes/Streams - Marketing software
In this programming assignment, youll identify the number of potential customers for a business. The starter program outputs the number of potential customers in a userentered age range given a file with peoples data.
1. Move the class Person to the separate files: person.h and person.cpp. Make sure you can still compile with separate files.
2. During file reading, the program isnt storing the gender or yearly income of the people. Instead, the default values are being printed. Fix the program to correctly set the data read from file.
The regions of the code that need to be fixed are marked with: FIXME Also set gender and yearly income
3. Allow the user to select the potential customers gender: male, female, or any. The program should now output only potential customers with the userspecified gender and age.
Update the GetUserInput function to prompt the user and store the users gender selection.
Also, create a function GetPeopleWithSpecificGender that returns only people with the userspecified gender.
Debugging suggestion: Use a function to print mains vector of Persons so that you can see who is in the vector after each function call. This technique may help debug the newly created function GetPeopleWithSpecificGender.
4. In addition to age and gender, allow the user to select the lower and upper range of a customers yearly income.
Update the GetUserInput function to prompt the user and store the users specified range. Also, create a function GetPeopleInIncomeRange that returns only people with the userspecified
yearly income.
The main should now look like the following code:
intmain(intargc,char*argv[]){ vector
hadError=ReadPeopleFromFile(argc,argv,people); if(hadError){
return1;//indicateserror }
GetUserInput(ageLowerRange,ageUpperRange,gender,yILowerRange, yIUpperRange);
people=GetPeopleInAgeRange(people,ageLowerRange,ageUpperRange); people=GetPeopleWithSpecificGender(people,gender); people=GetPeopleInIncomeRange(people,yILowerRange,yIUpperRange);
cout<<" Numberofpotentialcustomers="< return0; } Here is an example program execution with people.txt (user input is highlighted here for clarity): Openingfilepeople.txt. Age=20,gender=male,yearlyincome=25000 Age=25,gender=male,yearlyincome=45000 Age=23,gender=male,yearlyincome=30000 Age=16,gender=male,yearlyincome=7000 Age=30,gender=male,yearlyincome=55000 Age=22,gender=female,yearlyincome=27000 Age=26,gender=female,yearlyincome=44000 Age=21,gender=female,yearlyincome=37000 Age=18,gender=female,yearlyincome=17000 Age=29,gender=female,yearlyincome=62000 Finishedreadingfile. Enterlowerrangeofage:24 Enterupperrangeofage:30 Entergender(male,female,orany):any Enterlowerrangeofyearlyincome:43000 Enterupperrangeofyearlyincome:57000 Numberofpotentialcustomers=3 *****Starter code #include #include #include #include using namespace std; // Define a Person class, including age, gender, and yearlyIncome. class Person { public: Person(); void Print(); void SetData(int a); // FIXME Also set gender and yearly income int GetAge(); private: int age; string gender; int yearlyIncome; }; // Constructor for the Person class. Person::Person() { age = 0; gender = "default"; yearlyIncome = 0; return; } // Print the Person class. void Person::Print() { cout << "Age = " << this->age << ", gender = " << this->gender << ", yearly income = " << this->yearlyIncome << endl; return; } // Set the age, gender, and yearlyIncome of a Person. void Person::SetData(int a) { // FIXME Also set gender and yearly income this->age = a; return; } // Get the age of a Person. int Person::GetAge() { return this->age; } // Get a filename from program arguments, then make a Person for each line in the file. bool ReadPeopleFromFile(int argc, char* argv[], vector Person tmpPrsn; ifstream inFS; int tmpAge = 0; string tmpGender = ""; int tmpYI = 0; if (argc != 2) { cout << " Usage: [EXECUTABLE FILE] [TEXT DATA FILE], e.g. myprog.exe dev_people.txt" << endl; return true; // indicates error } cout << "Opening file " << argv[1] << ". "; inFS.open(argv[1]); // Try to open file if (!inFS.is_open()) { cout << "Could not open file " << argv[1] << ". "; return true; // indicates error } while (!inFS.eof()) { inFS >> tmpAge; inFS >> tmpGender; inFS >> tmpYI; tmpPrsn.SetData(tmpAge); // FIXME Also set gender and yearly income tmpPrsn.Print(); people.push_back(tmpPrsn); } inFS.close(); cout << "Finished reading file." << endl; return false; // indicates no error } // Ask user to enter age range. void GetUserInput(int &ageLowerRange, int&ageUpperRange) { cout<<" Enter lower range of age: "; cin >> ageLowerRange; cout << "Enter upper range of age: "; cin >> ageUpperRange; return; } // Return people within the given age range. vector unsigned int i = 0; vector int age = 0; for (i = 0; i < ppl.size(); ++i) { age = ppl.at(i).GetAge(); if ((age >= lowerRange) && (age <= upperRange)) { pplInAgeRange.push_back(ppl.at(i)); } } return pplInAgeRange; } int main(int argc, char* argv[]) { vector bool hadError = false; int ageLowerRange = 0; int ageUpperRange = 0; hadError = ReadPeopleFromFile(argc, argv, ptntlCstmrs); if( hadError ) { return 1; // indicates error } GetUserInput(ageLowerRange, ageUpperRange); ptntlCstmrs = GetPeopleInAgeRange(ptntlCstmrs, ageLowerRange, ageUpperRange); // FIXME Add the function GetPeopleWithSpecificGender //FIXME Addthefunction GetPeopleInIncomeRange cout << " Number of potential customers = "< return 0; } ****Use this data to complete the programming assignment for this topic.20 male 25000 25 male 45000 23 male 30000 16 male 7000 30 male 55000 22 female 27000 26 female 44000 21 female 37000 18 female 17000 29 female 62000
Step by Step Solution
There are 3 Steps involved in it
Step: 1
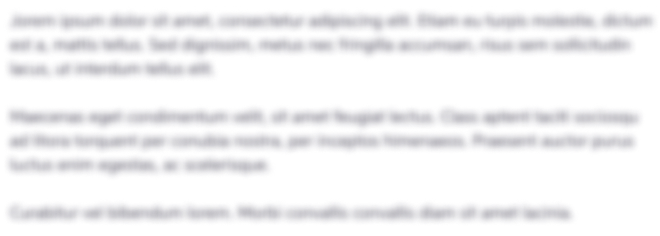
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started