Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Assignment code for Mathematic, CS I attached file codes for the assignment in the bottom please and please also make sure finish the extra credit
Assignment code for Mathematic, CS
I attached file codes for the assignment in the bottom please and please also make sure finish the extra credit assignment.
MathematicsRec.java
/** * FILL UP YOUR JAVA DOCUMENT HERE * @author FIRST_NAME LAST_NAME*/ class MathematicsRec { /** * The method returns a value which: * - Increases each of even decimal digits of n by one * - Decreases each of odd decimal digits of n by one * @param theDecimalNumber the input decimal number (n) * @return the new decimal number after digit adjustments */ public static long eduodd(long n) { return 0; } /** * The method accepts non-negative integer and returns a value as described below * @param theDecimalNumber is a non-negative decimal number (n) * @return the value in following way: * - return 1 when n = 0 * - return sum of fibby(floor(n/4)) and fibby(floor(3n/4)) when n > 0 */ public static int fibby(int n) { return 0; } /** * The method prints all consecutive values of n and its fibby value * @param theLowerBound the lower bound (start) * @param theUpperBound the upper bound (end) */ public static void stg(int n, int m) { } /** * The method returns the median that split the array into 3 parts * @param theList the list of integers (a) * @return the median */ public static double median3(int[] n) { return 0; } }
MathematicsTest.java
import java.io.ByteArrayOutputStream; import java.io.File; import java.io.PrintStream; import java.nio.file.Files; import java.nio.file.Paths; import java.security.MessageDigest; import java.security.NoSuchAlgorithmException; import java.util.Arrays; import java.util.Random; /** * The driver class to have sanity check on MathematicsRec class * @author Martin Hock*/ public class MathematicsTest { public static void rowColumn(String source, int offset) { int row = 1; int col = 1; for (int i = 0; i ExtraCredit.java
import java.io.BufferedWriter; import java.io.File; import java.io.FileOutputStream; import java.io.FileWriter; import java.io.IOException; import java.nio.ByteBuffer; import java.nio.channels.FileChannel; /** * The class is designed for extra credit * The student implements the fibby method using iterative approach * and then benchmark with their recursive approach to draw conclusion * @author Varik Hoang1. Even Digit Up - Odd Digit Down: Implement the method eduodd that accept an integer (long data type). eduodd( n ) returns a value which increases each of the even decimal digits of n by one and decreases each of the odd digits of n by one. Leading zero digits will disappear. The sign of eduodd( n ) should be the same as n, unless n is negative and eduodd( n) is zero as seen in the last example below. Here is the examples: 2. Fibby: Implement the method fibby that accepts an integer. Fibby is mathematically defined for nonnegative values in the following way: fibby(0)=1 fibby(n)=fibby(4n)+fibby(43n) where n>0 and n is the floor of n Here is the examnles- 3. Sparse Table Generation: Implement the method stg that accept two integers which are lower bound and upper bound. Print all consecutive values of n and fibby (n) (just the two numeric values separated by a space) where n start and nend. However, skip a line of output if the previous row printed has the same fibby value. For example, if you call stg(5,10), it should internally iterate as below: Hence, the expected output to the console should be: 6 8 Median: Implement the method median3 that accepts an array of integers and strictly follows the below strategy. Do not create any new arrays; you will need one or more helper methods that look at portions of the existing array. Consider an array of integers. You wish to find an approximate median. You have an idea: split the array into three pieces, find the approximate median of each piece, and then calculate the median (of the three approximate medians (if you put the three approximate medians in sorted order, the one in the middle). There are a few details to consider: - If the array is one element, that element itself is its own median. - If the array is two elements, take the mean (average) which may be a fractional value. - Otherwise, there are at least three elements in the array. There are three possibilities for the length: the length is divisible by 3 , it has a remainder of 1 when divided by 3 , or a remainder of 2 when divided by 3 . - If the length divided by 3 has a remainder of 0 : - Each piece should be n/3 elements. - If a remainder of 1 : - The first piece should consist of the first n/3 elements - The last piece should consist of the last n/3 elements - The middle piece is the remaining n/3] elements. n/3 rounds up. (For example, if there were 4 elements, the first piece is the first element, the last piece is the last element, and the middle two elements constitute the middle piece.) - If a remainder of 2 : - The first piece should consist of the first n/3 elements - The last piece should consist of the last [n/3] elements - The middle piece is the remaining n/3 elements. (For example, if there were 8 elements, the first piece is the first 3 elements, the last piece is the last 3 , and the middle two elements constitute the middle piece.) must name the file MathematicsRec.java that includes the methods as following: Extra credit is only considered if the assignment submit (and complete) on-time according to original due date on syllabus. The due date is extended will not count. Rewrite the fibby method using iterative approach and make sure it runs and produces the output correctly (you can check with your recursiv version). Compare the runtime of both approaches and draw conclusion based on your observation. The extra credits are only considered according to the correctness and the usefulness of your report. Please write your report in readme.txt file that provided in the project skeletc - 0.8% (TGS) or 0.04 (LGS) for representing two plots (one for iteration and one for recursion) on a single graph. The file must be incluc in the zip file. Each plot: - Must have at least 30 data points on the graph - Should be consistent with data generated from ExtraCredit.java - 0.2% (TGS) or 0.01 (LGS) for conclusion of which approach is better in specific scenario and why. (must have at least 100 words) Implementation Guidelines: The program does not compile will receive grade of zero. Place your code in a class named MathematicsRec. - Use the file Mathematics Test.java and run to have a local sanity check (please ignore this score): - Please do NOT leave any comment in the file MathematicsRec.java because the class Mathematies Test may detect some illegat requirements. Download: - MathematicsRec.java - Mathematics Test.java (use this file to test your functions for local sanity check and please ignore the score if shown) - ExtraCredit.java (use this file for extra credit only) - readme.txt (example) Submission: Submit to Canvas a zip file named assignment03_LASTNAME_FIRSTNAME.zip (all lower cases) that contains the following files: (assignment03_hoang_varik.zip for example) - MathematicsRec.java and ExtraCredit.java (optional) documented and styled according to class standards. - A readme.txt file that contains the following information 1. Your name 2. An estimate of the amount of time you spent completing the assignment 3. Any problems that exist in the project (if there are not any please state so) 4. Any questions you have for Varik about the assignment*/ public class ExtraCredit { /** * The method accepts non-negative integer and returns a value as described below * @param theDecimalNumber is a non-negative decimal number (n) * @return the value in following way: * - return 1 when n = 0 * - return sum of fibby(floor(n/4)) and fibby(floor(3n/4)) when n > 0 */ public static int fibby(int theDecimalNumber) { return 0; } public static void main(String args[]) { int[] dataset = new int[] { 10, 25, 50, 75, 100, 125, 150, 175, 200, 225, 250, // small dataset 500, 1000, 1500, 2000, 2500, 5000, 7500, 10000, 12500, 15000, 17500, // medium dataset 25000, 50000, 75000, 100000, 200000, 500000, 1000000, 2000000 // large dataset }; // sanity check the iterative fibby function if (fibby(1000000) != MathematicsRec.fibby(1000000)) { System.err.println("Please make sure your iterative fibby correct before doing the benchmark"); return; } long sum; int count; int attempts = 5; setContent("iterative_fibby.txt", ""); setContent("recursive_fibby.txt", ""); // benchmarking for (int value: dataset) { /** * The iterative fibby */ fibby(value); // start benchmarking for each value sum = 0; count = attempts; while (count --> 0) { long startTime = System.nanoTime(); fibby(value); long endTime = System.nanoTime(); // get the difference sum += endTime - startTime; } // write out the execution time in nanoseconds (/1000000 in milliseconds) appendContent("iterative_fibby.txt", (double) sum / attempts + " "); /** * The recursive fibby */ MathematicsRec.fibby(value); // start benchmarking for each value sum = 0; count = attempts; while (count --> 0) { long startTime = System.nanoTime(); MathematicsRec.fibby(value); long endTime = System.nanoTime(); // get the difference sum += endTime - startTime; } // write out the execution time in nanoseconds (/1000000 in milliseconds) appendContent("recursive_fibby.txt", (double) sum / attempts + " "); } } /** * The method writes content to file (overwrite if file is existed) * @param path the file along with the path * @param content the content is written to file */ protected static void setContent(String path, String content) { setContent(new File(path), content); } protected static void setContent(File file, String content) { BufferedWriter bw; try { bw = new BufferedWriter(new FileWriter(file)); bw.write(content); bw.flush(); bw.close(); } catch (IOException ex) { System.err.println(ex.getMessage()); } } /** * The method appends content to file (overwrite if file is existed) * @param path the file along with the path * @param content the content is appended to file */ protected static void appendContent(String path, String content) { appendContent(new File(path), content); } protected static void appendContent(File file, String content) { FileOutputStream fos; try { fos = new FileOutputStream(file, true); FileChannel channel = fos.getChannel(); ByteBuffer buffer = ByteBuffer.wrap(content.getBytes("UTF-8")); channel.write(buffer); channel.close(); } catch (IOException ex) { System.err.println(ex.getMessage()); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
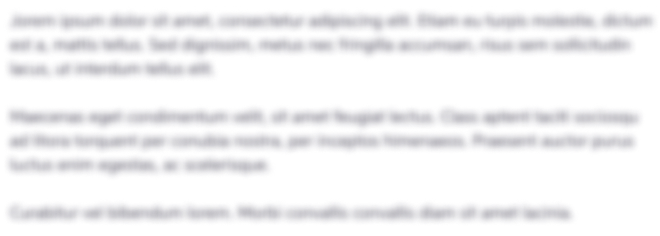
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started