Question
Assignment: Create these functions: I need to know if my input operator is correct and i need help making the string constructor 1. ComplexNumber(const char
Assignment: Create these functions: I need to know if my input operator is correct and i need help making the string constructor
1. ComplexNumber(const char *str) This constructor initializes a ComplexNumber object using a C-string with one of the following forms:
i. [+|-]realNumber ii. [+|-][realNumber]i iii. [+|-]realNumber(+|-)[realNumber]i
| stands for OR, [] stands for OPTIONALLY, () stands for EITHER, realNumber stands for any valid real number literal, including numbers in signed or unsigned scientific notation.
2. istream& operator>>(istream &in, ComplexNumber &c); This friend function allows input into ComplexNumber using cin and the extraction operator (i.e., >>).
Given Code: ComplexNumber.h
// Author: Ryan Carpenter // Date: 2/4/18 // Purpose: HW2
#include #include #include #include
using namespace std;
class ComplexNumber { public: // constructors (copy constructor not necessary, included for illustration) ComplexNumber(float real = 0.0, float imag = 0.0); ComplexNumber(const ComplexNumber &cn); ComplexNumber(const char *str);
// assignment operator (not necessary, included for illustration) ComplexNumber& operator=(const ComplexNumber &b);
// equality operators (not needed in HW2, included for illustration bool operator==(const ComplexNumber &b) const; bool operator!=(const ComplexNumber &b) const;
// getters (not needed in HW2) float real() const; float imag() const;
// operator functions friend ComplexNumber operator+(const ComplexNumber &, const ComplexNumber &); friend ComplexNumber operator-(const ComplexNumber &, const ComplexNumber &); friend ComplexNumber operator*(const ComplexNumber &, const ComplexNumber &); friend ComplexNumber operator/(const ComplexNumber &, const ComplexNumber &); friend ostream& operator<<(ostream &, const ComplexNumber &); friend istream& operator>>(istream &, ComplexNumber &c);
// data members private: float r; float i; };
ComplexNumber.cpp
// Author: Ryan Carpenter // Date: 2/4/18 // Purpose: HW2
#include #include #include "ComplexNumber.h"
// paste your solution for your new constructor here ComplexNumber::ComplexNumber(const char *str) { }
// paste your solution for your new input operator here istream& operator>>(istream&, ComplexNumber &c) { double r, i; //get the real part cin >> r; //now get the imaginary part cin >> i; c = ComplexNumber(r, i); return cin;
}
// two parameters constructor w/default parameters in .h -- default constructor ComplexNumber::ComplexNumber(float real, float imag) : r(real), i(imag) { }
// copy constructor -- unncessary seeing it does what the compiler-provided // copy constructor would do -- provided for illustration ComplexNumber::ComplexNumber(const ComplexNumber &cn) : r(cn.r), i(cn.i) { }
// assignment operator -- unncessary seeing it does what the compiler-provided // assignment operator would do -- provided for illustration ComplexNumber& ComplexNumber::operator=(const ComplexNumber &b) { r = b.r; i = b.i; return *this; }
// equality operator w/o which ComplexNumbers could not be compared bool ComplexNumber::operator==(const ComplexNumber &b) const { return (r == b.r && i == b.i); }
// non-equality operator provides an example of using other existing operators bool ComplexNumber::operator!=(const ComplexNumber &b) const { return !(*this == b); }
// accessors or getters float ComplexNumber::real() const { return r; } float ComplexNumber::imag() const { return i; }
// arithmetic operators follow as examples of friend functions ComplexNumber operator+ (const ComplexNumber &a, const ComplexNumber &b) { ComplexNumber result;
result.r = a.r + b.r; result.i = a.i + b.i;
return result; }
ComplexNumber operator- (const ComplexNumber &a, const ComplexNumber &b) { ComplexNumber result;
result.r = a.r - b.r; result.i = a.i - b.i;
return result; }
ComplexNumber operator* (const ComplexNumber &a, const ComplexNumber &b) { ComplexNumber result;
result.r = (a.r * b.r - a.i * b.i); result.i = (a.r * b.i + a.i * b.r);
return result; }
ComplexNumber operator/ (const ComplexNumber &a, const ComplexNumber &b) { ComplexNumber result;
result.r = (a.r * b.r + a.i * b.i) / (b.r * b.r + b.i * b.i); result.i = (a.i * b.r - a.r * b.i) / (b.r * b.r + b.i * b.i);
return result; }
// This operator is a "pretty-print" replacement for the output operator in ComplexNumber.cpp
ostream& operator<< (ostream &out, const ComplexNumber &b) {
bool rPrinted = false;
if (b.r != 0 || (b.r == 0 && b.i == 0)) { out << b.r; rPrinted = true; }
if (b.i > 0) { if (rPrinted) { out << "+"; } if (b.i != 1) { out << b.i; } out << "i"; } else if (b.i < 0) { if (b.i == -1) { out << "-"; } else { out << b.i; } out << "i"; }
return out; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
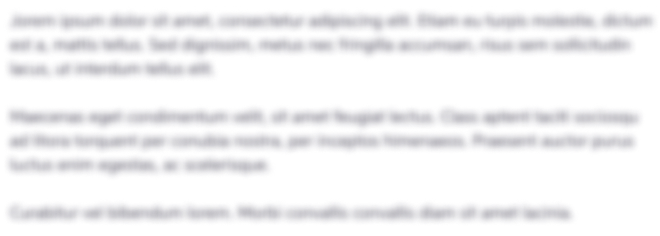
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started