Question
Assignment Scope For this assignment students will begin developing the front end, or User Interface, portion of the MasterMind game. Based on my professional experience
Assignment Scope
For this assignment students will begin developing the front end, or User Interface, portion of the MasterMind game. Based on my professional experience working in the industry I have always had to develop a UI for every application, therefore I translate that experience to students so they can have the same opportunity and be prepared professionally.
Typically, there is a one-to-one correlation of back end functionality to front end UI component. Depending upon the design of the application it doesnt always correlate perfectly, however with MasterMind, it works well.
Back-end functionality | Front-end UI component |
Codemaker.java | CodemakerUi.java |
Codebreaker.java | CodebreakerUi.java |
Game.java | MasterMindUi.java |
The goal is to develop the front-end components of the game MasterMind by creating classes:
CodebreakerUi.java
CodemakerUi.java
MasterMindUi.java
Students will integrate completed classes into their existing project.
Students will add an image icon to a JLabel.
The UI will be developed in multiple assignments, it is not expected that for Assignment 5 the fully functioning UI is complete.
The image that follows is a prototype of what the UI will look like. It does not have to be an exact match. The rubric will provide guidance and recommendations on how to accomplish this, however feel free to be creative in developing the look and feel of the UI.
To accomplish this:
Reference the tasks below for the specifics of the source code requirements.
Compress a project and submit to Webcourses
Decompress compressed project and verify it is a Netbeans project
References
Netbeans.docx
Setting up a project in Netbeans.docx
Netbeans right click menu help.docx
Deliverables
To complete this assignment you must submit your compressed Netbeans project to Webcourses.
Please keep in mind that the tasks are guidance to accomplish the goals of the assignment. At times students will be required to do additional research (e.g. Google That S**T (GTS)!) to find implementation options. In the industry, software engineers are expected to be very self-sufficient to find the best solution for the task at hand.
I have provided multiple code examples on Webcourses that shows how to implement numerous of the tasks below, please reference those code examples prior to asking me for help.
Tasks and Rubric
Activity | ||
mastermind package | ||
core package | ||
userInterface package | ||
RoundCornerButton.java | Download class and add to the userInterface package | |
RoundButton.java | Download class and add to the userInterface package | |
CodebreakerUi.java | Add member variables of type: RoundButton[] buttons; RoundButton[][] attempts; | |
Update method initComponents(), it should do the followingFor the codebreakerColors JPanel Set the layout manager to use the default FlowLayout Instantiate the 1-D array buttons of RoundButton objects to the size of eight Loop through the array to do the following:Instantiate and instance of class RoundButton Instantiate an instance of class Color set equal to one of the colors stored in the Constants. codeColors ArrayList Set the background color of the instance of class RoundButton in step 1 above using the instance of class Color in step 2 above as an argument Call method .putClientProperty() on the instance of class RoundButton in step 1 above passing argument color and the instance of class Color in step 2 above Evaluate the instance of class Color in step 2 above to set the tool tip text of the RoundButton instance in step 1 above, call method setToolTipText on the RoundButton instance passing the Color instance as an argument Add the RoundButton instance to the codebreakerColors JPanel | ||
Update method initComponents(), it should do the followingFor the codebreakerAttempt JPanel Set the layout manager to use GridLayout so that it is 10 rows by 4 columns Instantiate the 2-D array attempts of RoundButton objects to the size of 10 rows by 4 columns Loop through the array to do the following:Instantiate and instance of class RoundButton Disable the RoundButton instance if it isnt in the bottom row on the UI Add the RoundButton instance to the codebreakerAttempt JPanel | ||
CodemakerUi.java | Add member variables of type: JLabel[] secretLabels; JLabel[][] responseLabels; ImageIcon question; | |
Update method initComponents(), it should do the followingFor the secretCode JPanel Set the layout manager to use the default FlowLayout Instantiate the 1-D array secretLabels of JLabel objects to the size of four (2 points) Instantiate the instance of class ImageIcon passing as an argument to the constructor similar to the following: getClass().getResource("question.jpg"); the JPG passed is the image I used, you will need to search for an image that you desire to use, save this image to the userInterface package (10 points) Loop through the array to do the following:Instantiate an instance of class JLabel (2 points) Instantiate an instance of class ImageIcon set equal to the method call imageResize() passing as an argument the instance of ImageIcon in step iii above (NOTE: source code for method imageResize() is provided before figure 1 below) (10 points) Call method setIcon() on the JLabel instance in step 1 above passing as an argument the instance of class ImageIcon in step iii above (4 points) Add the JLabel instance to the secretCode JPanel (2 points) Instantiate an instance of class JButton passing as an argument text Check or something similar (3 points) Add the JButton instance in step v above to the secretCode JPanel (2 points) | ||
Update method initComponents(), it should do the followingFor the codemakerResponse JPanel Instantiate the 2-D array responseLabels of JLabel objects to the size of 10 rows by 4 columns Loop through the array to do the following:Instantiate and instance of class JLabel Call method .setBorder() passing as an argument your desired border type from the BorderFactory class Add the JLabel instance to the codemakerResponse JPanel | ||
Mastermind application | ||
Test Case 1 | Test Case 1 passes | |
Test Case 2 | Test Case 2 passes | |
Test Case 3 | Test Case 3 passes | |
Test Case 4 | Test Case 4 passes | |
Test Case 5 | Test Case 5 passes | |
Source compiles with no errors | ||
Source runs with no errors | ||
Source includes comments | ||
Total |
Perform the following test cases
Test Cases | ||
Action | Expected outcome | |
Test Case 1 | Rregression Testing: Initial JOptionPane displays | JOptionPane is similar to figure 1 |
Test Case 2 | Mastermind Initial UI displays | Mastermind UI looks similar figure 2 |
Test Case 3 | Enabled buttons are selectable | Button should highlight, similar to figure 3 |
Test Case 4 | Disabled buttons are not selectable | Button should not highlight, similar to figure 4 |
Test Case 5 | Project view | Project view matches figure 5 except the image |
Source code to resize images
Note: The first two arguments passed to method getScaledInstance() represent the size in pixels of width and height.
private ImageIcon imageResize(ImageIcon icon)
{
Image image = icon.getImage();
Image newImage = image.getScaledInstance(30, 30, java.awt.Image.SCALE_SMOOTH);
icon = new ImageIcon(newImage);
return icon;
}
Figure 1 Test Case 1
Figure 2 Mastermind Initial UI
Figure 3 JButton is selectable
Figure 4 JButton is not selectable
Figure 3 Project View
Code So far:
Constants.java
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package constants; import java.awt.Color; import java.util.ArrayList; import java.util.Arrays; /** * * */ public class constants { // peg color options public static final ArrayListcodeColors = new ArrayList (Arrays.asList(Color.BLUE, Color.BLACK, Color.ORANGE, Color.WHITE, Color.YELLOW, Color.RED, Color.GREEN, Color.PINK)); // response color options public static final ArrayList responseColors = new ArrayList (Arrays.asList(Color.RED, Color.WHITE)); public static final int MAX_ATTEMPTS = 10; public static final int MAX_PEGS = 4; public static final int COLORS = 8; }
Codebreaker.java
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package core; import java.awt.Color; import java.lang.reflect.Field; import java.util.ArrayList; import java.util.Scanner; import constants.constants; public abstract class Codebreaker implements ICodebreaker { // member variables private ArrayListcodebreakerAttempt; public Codebreaker() { // instanatiate the member variables codebreakerAttempt = new ArrayList(); } /** * @return the codebreakerAttempt */ public ArrayList getCodebreakerAttempt() { consoleAttempt(); return codebreakerAttempt; } /** * @param codebreakerAttempt the codebreakerAttempt to set */ public void setCodebreakerAttempt(ArrayList codebreakerAttempt) { this.codebreakerAttempt = codebreakerAttempt; } /** * * @param attempt * @return */ public boolean CheckCode(ArrayList attempt) { boolean errorFlag=false; for (Color color : attempt) { if(color!=Color.RED){ //only if ALL the colors in the list are red, attempt is correct else wrong / partially correct errorFlag=true; } } if(errorFlag){ System.out.println(); System.out.println("Found correct color in right position!"); for (int i=0;i { System.out.print(" "+col); }); System.out.println(); } else{ consoleAttempt(); } } private Color stringToColor(String colorString){ Color color; try{ Field field=Class.forName("java.awt.Color").getField(colorString); color=(Color)field.get(null); }catch (Exception e) { color=null; } return color; } }
Codemaker.java
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package core; import constants.constants; import java.awt.Color; import java.util.ArrayList; import java.util.HashSet; import java.util.Random; import java.util.Set; public final class Codemaker implements ICodemaker { // member variables private SetsecretCode; private ArrayList codemakerResponse; public Codemaker() { // instantiate the member variable objects secretCode = new HashSet(); codemakerResponse = new ArrayList(); // call the method to generate the secret code generateSecretCode(); } public void generateSecretCode() { Random random = new Random(); //randomly select four of the eight colors to be the secret code, only // use each color once while(secretCode.size() { System.out.println(color.toString()); }); } /** * @return the secretCode */ public Set getSecretCode() { return secretCode; } /** * @param secretCode the secretCode to set */ public void setSecretCode(Set secretCode) { this.secretCode = secretCode; } /** * @return the codemakerResponse */ public ArrayList getCodemakerResponse() { return codemakerResponse; } /** * @param codemakerResponse the codemakerResponse to set */ public void setCodemakerResponse(ArrayList codemakerResponse) { this.codemakerResponse = codemakerResponse; } public void checkAttemptedCode(ArrayList attempt) { codemakerResponse.clear(); //resetting the response System.out.println("Codemaker is checking codebreaker attempt"); ArrayList evaluatedPos=new ArrayList (); //List for storing indices of right guesses ArrayList whitePegs=new ArrayList (); //A temporary list for storing indices of matching colors, with wrong positions int red_pegs=0,white_pegs=0; ArrayList secret=new ArrayList (); secret.addAll(getSecretCode()); if(secret.equals(attempt)){ red_pegs=4; white_pegs=0; for(int i=0;i Game.java
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package core; import java.awt.Color; import java.util.ArrayList; import constants.constants; public final class Game implements IGame { private int attempt; private Codebreaker codebreaker; private Codemaker codemaker; public Game() { // instantiate the instances of the member variables codemaker = new Codemaker(); codebreaker = new Codebreaker() { @Override public void checkCode(ArrayListattempt) { throw new UnsupportedOperationException("Not supported yet."); // To // change // body // of // generated // methods, // choose // Tools // | // Templates. } }; attempt = 0; // play(); } public void play() { while (true) { // will loop again and again until codebreaker wins or // runs out of attempts if (attempt codeBreakerAttempt = codebreaker.getCodebreakerAttempt(); codemaker.checkAttemptedCode(codeBreakerAttempt); boolean win = codebreaker.CheckCode(codemaker .getCodemakerResponse()); /* * checkCode function of * ICodebreaker has been * modified,will return true * if codebreaker wins */ if (win) { System.out.println("Codebreaker wins"); break; // breaking the loop } } else { System.out.println("Codemaker wins"); // after max attempts and // codebreaker couldn't // guess correctly break; } attempt++; } } /** * @return the attempt */ public int getAttempt() { return attempt; } /** * @param attempt * the attempt to set */ public void setAttempt(int attempt) { this.attempt = attempt; } /** * @return the codebreaker */ public Codebreaker getCodebreaker() { return codebreaker; } /** * @param codebreaker * the codebreaker to set */ public void setCodebreaker(Codebreaker codebreaker) { this.codebreaker = codebreaker; } /** * @return the codemaker */ public Codemaker getCodemaker() { return codemaker; } /** * @param codemaker * the codemaker to set */ public void setCodemaker(Codemaker codemaker) { this.codemaker = codemaker; } public void checkIfWon() { } } Mastermind.java package mastermind; import core.Game; import userinterface.MasterMindUi; import javax.swing.JOptionPane; public class MasterMind { public static void main(String[] args) { // System.out.println("Welcome to MasterMind!"); JOptionPane.showMessageDialog(null, "Let's Play MasterMind!"); Game game = new Game(); MasterMindUi ui = new MasterMindUi(game); } }CodebreakerUi
package userinterface; import javax.swing.JPanel; import javax.swing.BorderFactory; import java.awt.Dimension; import core.Codebreaker; public class CodebreakerUi { // Instance variables private JPanel codebreakerAttempt; private JPanel codebreakerColors; private Codebreaker codebreaker; /** * Constructor * * @param codebreaker * - Codebreaker object */ public CodebreakerUi(Codebreaker codebreaker) { initComponents(codebreaker); } /** * Initialize all the components for the UI. * * @param codebreaker * - Codebreaker object */ private void initComponents(Codebreaker codebreaker) { this.codebreaker = codebreaker; this.codebreakerAttempt = new JPanel(); this.codebreakerColors = new JPanel(); // Set jpanel sizes codebreakerAttempt.setMinimumSize(new Dimension(430, 400)); codebreakerAttempt.setPreferredSize(new Dimension(430, 400)); codebreakerColors.setMinimumSize(new Dimension(300, 50)); codebreakerColors.setPreferredSize(new Dimension(300, 50)); // Set border codebreakerAttempt.setBorder(BorderFactory.createTitledBorder("Codebreaker Attempt")); codebreakerColors.setBorder(BorderFactory.createTitledBorder("Codebreaker Colors")); } /** * Returns the codebreakerAttempt */ public JPanel getCodebreakerAttempt() { return codebreakerAttempt; } /** * Returns the codebreakerColors */ public JPanel getCodebreakerColors() { return codebreakerColors; } }CodemakerUi
package userinterface; import javax.swing.JPanel; import java.awt.Dimension; import javax.swing.BorderFactory; import core.Codemaker; public class CodemakerUi { // Instance variables private JPanel codemakerResponse; private JPanel secretCode; private Codemaker codemaker; /** * Constructor * * @param codemaker * - Codemaker object */ public CodemakerUi(Codemaker codemaker) { initComponents(codemaker); } /** * Initialize all the components for the UI. * * @param codemaker * - Codemaker object */ private void initComponents(Codemaker codemaker) { this.codemaker = codemaker; this.codemakerResponse = new JPanel(); this.secretCode = new JPanel(); // Set jpanel sizes codemakerResponse.setMinimumSize(new Dimension(150, 400)); codemakerResponse.setPreferredSize(new Dimension(150, 400)); secretCode.setMinimumSize(new Dimension(300, 50)); secretCode.setPreferredSize(new Dimension(300, 50)); // Set border codemakerResponse.setBorder(BorderFactory.createTitledBorder("Codemaker Response")); secretCode.setBorder(BorderFactory.createTitledBorder("Secret Code")); } /** * Returns the codemakerResponse */ public JPanel getCodemakerResponse() { return codemakerResponse; } /** * Returns the secretCode */ public JPanel getSecretCode() { return secretCode; } }MasterMindUi.java /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ //bryan tavarez package userinterface; import core.Game; import java.awt.BorderLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.*; public class MasterMindUi { // Instance variables private Game game; private CodebreakerUi codebreakerUi; private CodemakerUi codemakerUi; private JFrame frame; private JMenuBar menuBar; private JMenu gameMenu; private JMenu helpMenu; private JMenuItem newGameMenuItem; private JMenuItem exitMenuItem; private JMenuItem aboutMenuItem; private JMenuItem rulesMenuItem; /** * Constructor * * @param game * - Game object */ public MasterMindUi(Game game) { this.game = game; // Instantiate CodemakerUI this.codemakerUi = new CodemakerUi(game.getCodemaker()); // Instantiate CodebreakerUI this.codebreakerUi = new CodebreakerUi(game.getCodebreaker()); initComponents(); } /** * Initializes the UI components */ private void initComponents() { frame = new JFrame("Mastermind"); // Set the default size of the JFrame frame.setSize(600, 500); // Set the default close operation of the JFrame frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Use default layout manager BorderLayout frame.setLayout(new BorderLayout(10, 0)); // Center frame to the screen frame.setLocationRelativeTo(null); // Set up the JMenuBar menuBar = new JMenuBar(); // Add JMenu Game to the JMenuBar gameMenu = new JMenu("Game"); menuBar.add(gameMenu); // Add JMenuItems New Game and Exit the JMenu Game newGameMenuItem = new JMenuItem("New Game"); gameMenu.add(newGameMenuItem); exitMenuItem = new JMenuItem("Exit"); gameMenu.add(exitMenuItem); // Add JMenu Help to the JMenuBar helpMenu = new JMenu("Help"); menuBar.add(helpMenu); // Add JMenuItems About and Game Rules to the JMenu Help aboutMenuItem = new JMenuItem("About"); helpMenu.add(aboutMenuItem); rulesMenuItem = new JMenuItem("Game Rules"); helpMenu.add(rulesMenuItem); // Add JMenuBar to the JFrame frame.setJMenuBar(menuBar); // Add the CodemakerUi JPanels to the JFrame using the getters defined // in the class frame.add(this.codemakerUi.getSecretCode(), BorderLayout.NORTH); frame.add(this.codemakerUi.getCodemakerResponse(), BorderLayout.EAST); // Add the CodebreakerUi JPanels to the JFrame using the getters defined // in the class frame.add(this.codebreakerUi.getCodebreakerAttempt(), BorderLayout.WEST); frame.add(this.codebreakerUi.getCodebreakerColors(), BorderLayout.SOUTH); // Add action listener to exit menu item exitMenuItem.addActionListener(new ExitActionListener()); // Add action listener to about menu item aboutMenuItem.addActionListener(new AboutActionListener()); // Add action listener to game rules menu item rulesMenuItem.addActionListener(new RulesActionListener()); // Set the visibility of the JFrame frame.setVisible(true); } // Inner class to create an ActionListener for Exit menu item class ExitActionListener implements ActionListener { @Override public void actionPerformed(ActionEvent ae) { // Display a JOptionPane message confirming the user wants to exit // using method showConfirmDialog() int ans = JOptionPane.showConfirmDialog(null, "Confirm to exit Mastermind?", "Exit?", JOptionPane.YES_NO_OPTION); // If yes, exit the application by calling method System.exit() // passing the value of 0 as an argument // If no, do not exit the application if (ans == 0) System.exit(0); } } // Inner class to create an ActionListener for About menu item class AboutActionListener implements ActionListener { @Override public void actionPerformed(ActionEvent ae) { // Display a JOptionPane message informing the user: // Application name and version // Author // Date of development JOptionPane.showMessageDialog(null, "Mastermind version 1.0 Karin Whiting Fall 2018"); } } // Inner class to create an ActionListener for Game rules menu item class RulesActionListener implements ActionListener { @Override public void actionPerformed(ActionEvent ae) { String rules = "Step 1: The codemaker selects a four color secret code, in any order, no duplicate colors. " + "Step 2: The codemaker places a guess in the bottom row, no duplicate colors. " + "Step 3: The codemaker gives feedback next to each guess row with four pegs " + "~Each red peg means that one of the guessed colors is correct, and is in the right location. " + "~Each white peg means that one of the guessed colors is correct, but is in the wrong location. " + "Step 4: Repeat with the next row, unless the secret code was guessed on the first turn " + "Step 5: Continue until the secret code is guessed or there are no more guesses left, there are 10 attempts"; // Display a JOptionPane message informing the user about the rules // of the game JOptionPane.showMessageDialog(null, rules); } } }Check
Step by Step Solution
There are 3 Steps involved in it
Step: 1
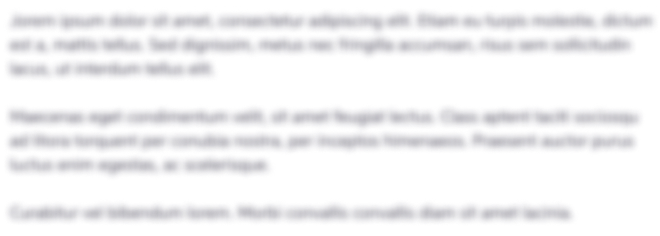
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started