Question
Assignment : This assignment focuses on process management within an operating system, including processor scheduling. You will design and implement the C/C++ program which is
Assignment :
This assignment focuses on process management within an operating system, including processor scheduling. You will design and implement the C/C++ program which is described below.
Assignment Objectives To familiarize you with the use of semaphores, thread usage, and to get a better understanding of process management Assignment Specifications The program will simulate the steps that an operating system takes to manage user processes. The operating system uses a ve-state process model: New, Ready, Running, Blocked, and Exit. When a process is created, it is placed in the New state; it moves into the Ready state when a Process Control Block (PCB) becomes available. When a process is dispatched, it moves into the Running state. While in the Running state, a process may issue two types of requests: a request to halt (which is issued when a process has completed its processing and is ready to leave the system), and a request to block (which is issued when the process must wait for some event, such as an I/O operation). When a process becomes unblocked, it moves from the Blocked state to the Ready state.
1. Your program will process a le which contains the simulation parameters and the process stream. The first four lines of the le will contain the simulation parameters (one item per line):
a) Number of CPUs in the system (the integer value 1 for this assignment)
b) Number of PCBs in the system (the integer value 1 for this assignment) c) Length of the simulation (in ticks) d) Short-term scheduling algorithm (the string "FCFS" for this assignment) 1 2. Exactly one line will follow the simulation parameters to represent the process stream. That line will contain the following elds, separated by blanks: a) Process identi cation number b) Priority (from 1 to 3)
c) Number of CPU bursts
d) Burst time (in ticks)
e) Blocked time (in ticks) f) Arrival time (in ticks since start of simulation)
The number of CPU bursts is an integer value representing the number of times that the process needs access to the CPU. The burst time is an integer value representing the length of time that the process uses the CPU before issuing a service request. The blocked time is an integer value representing the length of time that the process is blocked after issuing a service request.
3. When the process terminates, the program will display the following information about that process:
a) All process parameters (items (2a) to (2f) above)
b) Departure time (in ticks since start of simulation)
c) Cumulative time in the New state (in ticks)
d) Cumulative time in the Ready state (in ticks)
e) Cumulative time in the Running state (in ticks)
f) Cumulative time in the Blocked state (in ticks)
g) Turnaround time (in ticks)
h) Normalized turnaround time *** The following (i.e. #4) would be nice to have, but not a requirement yet.
4. The program will accept two command-line arguments: an integer representing the value of N and the name of the input le. After every N ticks of simulated time, the program will display the following information: a) Process ID of each process in the New state b) Process ID of each process in the Ready state c) Process ID of each process in the Running state d) Process ID of each process in the Blocked state The program will only display the information if N is a positive integer value.
5. The program will include appropriate error-handling. Assignment Overview (or additional specs). Were going to implement semaphores to synchronize the process management stages. Additionally, well be using threads to represent the various stages. Below is a general, and probably incomplete, overview of this project.
*** All queues can be global
NewQueue: FIFO
// FreePCBQueue: NA
ReadyQueue: FIFO
RunningState: NA
BlockedQueue: FIFO
ExitQueue: FIFO
Global Variables
Int Time = 0;
Int FreePCB = 5
Semaphores
SemTimer (1)
semRunning(0)
semBlocked(0)
semReady(0)
semExit(0)
ClockThread
While:
Sleep(2) // number susceptible to change
Time++;
semTimer.signal()
NewThread
While
semTimer.wait()
Check to add new process to NewQueue, add if new process starts at time N
If free PCB available, add process to ReadyQueue
Decrement FreePCBQueue by one
Remove appropriate process from NewQueue insert into ReadyQueue
semBlocked.signal()
Blocked
While
semBlocked.wait()
for each process in BlockedQueue
increment process.currentblocktime
if( process.currentblockedtime == process.blockedtime) process.
currentblockedtime = 0
remove process from BlockedQueue add to ReadyQueue
semRunning.signal()
Running
While
semRunning.wait()
increment process.currentRuntime
if(currentbursttime == bursttime)
vacate RunningState
increment process.burstsRun
if(process.burstsRun == process.totalBursts)
place process in ExitQueue
else
add process to BlockedQueue
semReady.signal()
Ready
While
semReady.wait()
if(RunningState is vacated)
remove appropriate
process from ReadyQueue process.currentRuntime = 0
add process to RunningState
semExit.signal()
Exit
While
semExit.wait()
print out data as specified above
increment FreePCB free any resources associated with running process as it now exits
Step by Step Solution
There are 3 Steps involved in it
Step: 1
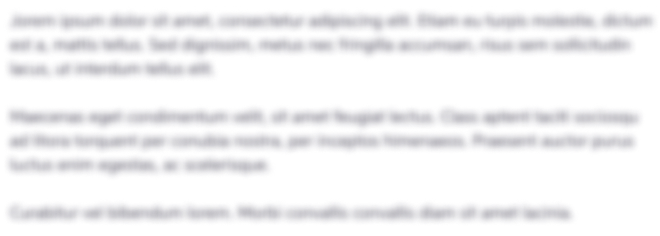
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started