Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Assignment Title: Student Records Management Description: Create a program to manage student records. The program should allow the user to read student information from a
Assignment Title: Student Records Management
Description:
Create a program to manage student records. The program should allow the user to read student
information from a file, perform operations on the records, and write the modified records back to a
file. You will also implement inheritance to handle different types of students.
Instructions:
Create a class named "Student" with the following attributes:
id int: The student's identification number.
name String: The student's name.
grade double: The student's overall grade.
Create a constructor in the Student class to initialize the attributes.
Create a method named "displayInfo" in the Student class that prints the student's information.
Create two subclasses named "UndergraduateStudent" and "GraduateStudent" that inherit from
the Student class.
The UndergraduateStudent class should have an additional attribute:
year int: The student's current year of study.
The GraduateStudent class should have an additional attribute:
researchTopic String: The topic of the student's research.
Implement the necessary constructors and displayInfo methods in the subclasses.
Implement equals method in all classes.
Create a main class named "StudentRecordsManager" that will serve as the entry point for your
program.
In the StudentRecordsManager class, implement the following methods:
readFromFileString filename: Reads student records from a file specified by the filename
parameter. Each line in the file should contain a student's information in the following format:
idname,grade,year,researchTopic" for GraduateStudent or idname,grade,year" for
UndergraduateStudent Store the records in an appropriate data structure eg ArrayList
writeToFileString filename, ArrayList students: Writes the student records stored in
the ArrayList back to a file specified by the filename parameter. Each line in the file should follow
the same format as mentioned above.
displayStudentInfoArrayList students: Displays the information of all students in the
ArrayList.
calculateAverageGradeArrayList students: Calculates and returns the average grade
of all students.
In the main method of the StudentRecordsManager class, create an instance of the class and
perform the following actions:
Call the readFromFile method to read student records from a file.
Call the displayStudentInfo method to display the student records.
Call the calculateAverageGrade method and display the average grade.
Modify the records eg change the grade of a student as per the rules below:
Increase scores between by points so will be will be etc.
Increase score by points
Decrease score by points
Call the calculateAverageGrade method and display the average grade.
Call the writeToFile method to write the modified records back to a file.
Call readFromFile and then displayStudentInfo method again to verify the changes.
Note: Make sure to handle file IO exceptions and provide appropriate error messages to the user.
Submission:
Submit the Java source code files screenshot of the output and updated student record file. Also
include a writeup that describes your implementation. Submission without the writeup will get a score
of
Remember to comment your code appropriately and provide meaningful variable and method names.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
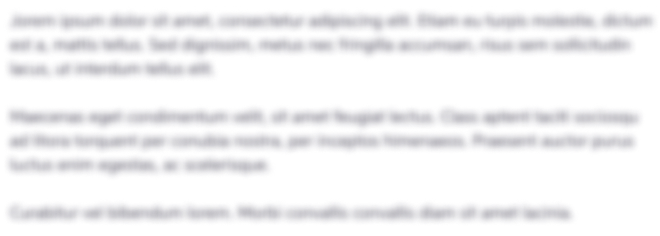
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started