Question
Assignment: Write a MIPS assembly language program to calculate some geometric information for each hexagon1 in a series of hexagons. Specifically, the program will find
Assignment: Write a MIPS assembly language program to calculate some geometric information for each hexagon1 in a series of hexagons. Specifically, the program will find the area for each of the hexagon in a set of hexagons. The formula for hexagon area is as follows: hexAreas[i ] = sideLens[ i] * apothemLens[i] / 2 After the areas have been computed, the program should find the minimum, maximum, middle value, sum, and average for the areas. Since the data is not sorted, we will obtain the middle value as follows. For an even length list, the middle value is computed by summing the two middle values and dividing by 2. The program must display the results to the console window. The output should look something like the following (with the correct answers displayed):
################################################################################
# data segment
.data
sideLens:
.word 15, 25, 33, 44, 58, 69, 72, 86, 99, 101
.word 369, 374, 377, 379, 382, 384, 386, 388, 392, 393
.word 501, 513, 524, 536, 540, 556, 575, 587, 590, 596
.word 634, 652, 674, 686, 697, 704, 716, 720, 736, 753
.word 107, 121, 137, 141, 157, 167, 177, 181, 191, 199
.word 102, 113, 122, 139, 144, 151, 161, 178, 186, 197
.word 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
.word 202, 209, 215, 219, 223, 225, 231, 242, 244, 249
.word 203, 215, 221, 239, 248, 259, 262, 274, 280, 291
.word 251, 253, 266, 269, 271, 272, 280, 288, 291, 299
.word 1469, 2474, 3477, 4479, 5482, 5484, 6486, 7788, 8492, 1493
apothemLens:
.word 32, 51, 76, 87, 90, 100, 111, 123, 132, 145
.word 634, 652, 674, 686, 697, 704, 716, 720, 736, 753
.word 782, 795, 807, 812, 827, 847, 867, 879, 888, 894
.word 102, 113, 122, 139, 144, 151, 161, 178, 186, 197
.word 1782, 2795, 3807, 3812, 4827, 5847, 6867, 7879, 7888, 1894
.word 206, 212, 222, 231, 246, 250, 254, 278, 288, 292
.word 332, 351, 376, 387, 390, 400, 411, 423, 432, 445
.word 10, 12, 14, 15, 16, 22, 25, 26, 28, 29
.word 400, 404, 406, 407, 424, 425, 426, 429, 448, 492
.word 457, 487, 499, 501, 523, 524, 525, 526, 575, 594
.word 1912, 2925, 3927, 4932, 5447, 5957, 6967, 7979, 7988, 1994
hexAreas:
.space 440
len: .word 110
haMin: .word 0
haMid: .word 0
haMax: .word 0
haSum: .word 0
haAve: .word 0
LN_CNTR = 7
# -----
hdr: .ascii "MIPS Assignment #1 "
.ascii "Program to calculate area of each hexagon in a series "
.ascii "of hexagons. "
.ascii "Also finds min, mid, max, sum, and average for the "
.asciiz "hexagon areas. "
new_ln: .asciiz " "
blnks: .asciiz " "
a1_st: .asciiz " Hexagon min = "
a2_st: .asciiz " Hexagon mid = "
a3_st: .asciiz " Hexagon max = "
a4_st: .asciiz " Hexagon sum = "
a5_st: .asciiz " Hexagon ave = "
###########################################################
# text/code segment
.text
.globl main
.ent main
main:
# -----
# Display header.
la $a0, hdr
li $v0, 4
syscall # print header
# --------------------------------------------------
# YOUR CODE GOES HERE
##########################################################
# Display results.
la $a0, new_ln # print a newline
li $v0, 4
syscall
la $a0, new_ln # print a newline
li $v0, 4
syscall
# Print min message followed by result.
la $a0, a1_st
li $v0, 4
syscall # print "min = "
lw $a0, haMin
li $v0, 1
syscall # print min
# -----
# Print middle message followed by result.
la $a0, a2_st
li $v0, 4
syscall # print "med = "
lw $a0, haMid
li $v0, 1
syscall # print mid
# -----
# Print max message followed by result.
la $a0, a3_st
li $v0, 4
syscall # print "max = "
lw $a0, haMax
li $v0, 1
syscall # print max
# -----
# Print sum message followed by result.
la $a0, a4_st
li $v0, 4
syscall # print "sum = "
lw $a0, haSum
li $v0, 1
syscall # print sum
# -----
# Print average message followed by result.
la $a0, a5_st
li $v0, 4
syscall # print "ave = "
lw $a0, haAve
li $v0, 1
syscall # print average
# -----
# Done, terminate program.
endit:
la $a0, new_ln # print a newline
li $v0, 4
syscall
li $v0, 10
syscall # all done!
.end main
MIPS Assignment #1 Program to calculate area of each hexagon in a series of hexagons. Also finds min, mid, max, sum, and average for the hexagon areas 240 637 1254 1914 2610 3450 3996 5289 6534 7322 116973 121924 127049 129997 133127 135168 138188 139680 144256 147964 195891 203917 211434 217616 223290 235466 249262 257986 261960 266412 32334 36838 41114 47677 50184 [... display all numbers ...1 Hexagon min -'? Hexagon mid - ? Hexagon max ? Hexagon sum = ? Hexagon ave = ? MIPS Assignment #1 Program to calculate area of each hexagon in a series of hexagons. Also finds min, mid, max, sum, and average for the hexagon areas 240 637 1254 1914 2610 3450 3996 5289 6534 7322 116973 121924 127049 129997 133127 135168 138188 139680 144256 147964 195891 203917 211434 217616 223290 235466 249262 257986 261960 266412 32334 36838 41114 47677 50184 [... display all numbers ...1 Hexagon min -'? Hexagon mid - ? Hexagon max ? Hexagon sum = ? Hexagon ave =Step by Step Solution
There are 3 Steps involved in it
Step: 1
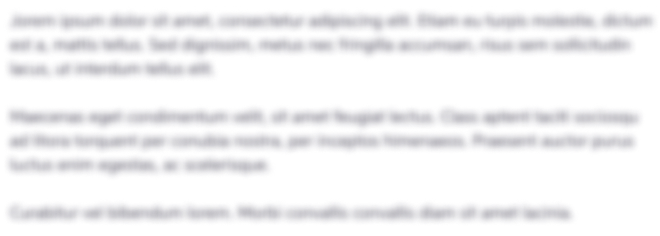
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started