Question
Assignment: Your program should randomly generate a number from 1 - 1000 to represent the number of jellybeans in the jar. Then your program should
Assignment:
- Your program should randomly generate a number from 1 - 1000 to represent the number of jellybeans in the jar. Then your program should ask the user to try and guess the number of jellybeans. If the user guesses incorrectly, the program should ask them to try again until the guess is correct; when the guess is correct, the program should print a congratulatory message! Use a do ... while loop .
Finally, keep prompting the user to ask if they want to play again.
Here is a strategy to assist in working through this problem:
- Step 1. Download the skeleton file called JellyBeanGame.java to use as a starting point. Add to this starting code so that the program plays the game as described above. Use a do..while loop. If the guess is wrong, the program says whether it is too high or too low. Get this step working before going to the next step.
- Step 2. Now add code to count how many guesses it takes the user to get the correct number of jellybeans, and print this number at the end with the congratulatory message.
- Step 3. Add code that prompts the user if they want to play another game and will continue until the user wants to stop. A while loop will work.
Question: The script works except when I type y to play the game again it ends the game instead of starting over and I'm not sure how to fix that. Thanks.
My Script:
import java.util.Scanner;
import java.util.Random;
public class JellyBeanGame
{
public static void main(String[] args)
{
int numOfJellyBeans = 0; //Number of jelly beans in jar
int guess = 0;//The user's guess
String again = "y";
int num_guesses = 0;
Random generator = new Random();
Scanner scan = new Scanner (System.in);
//randomly generate the number of jelly beans in jar
numOfJellyBeans = generator.nextInt(10)+1; //Generates an integer between 1 to 10
// Part 2.
do
{
System.out.println("There are between 1 and 1000 jellybeans in the jar,");
System.out.println("Enter your guess: "); //Prompts user to enter in a guess
guess = scan.nextInt();
num_guesses++;
if (guess > numOfJellyBeans)
{
System.out.println("Too High!"); // If the guess is the wrong display message
}
else if (guess < numOfJellyBeans)
{
System.out.println("Too Low!"); // If the guess is the wrong display message
}
else
{
System.out.println("High Five! You got it, " + num_guesses + " guesses"); // If the guess is correctly displays this message
}
}
// Part 3.
while (guess != numOfJellyBeans);
System.out.print(" Play again? y or n: "); // Asks user if they want to play again
again = scan.next();
System.out.println("See you later!"); //Message if they say n
scan.close();
}
}
Output:
There are between 1 and 1000 jellybeans in the jar,
Enter your guess:
12
Too High!
There are between 1 and 1000 jellybeans in the jar,
Enter your guess:
5
Too Low!
There are between 1 and 1000 jellybeans in the jar,
Enter your guess:
6
Too Low!
There are between 1 and 1000 jellybeans in the jar,
Enter your guess:
7
Too Low!
There are between 1 and 1000 jellybeans in the jar,
Enter your guess:
8
Too Low!
There are between 1 and 1000 jellybeans in the jar,
Enter your guess:
9
Too Low!
There are between 1 and 1000 jellybeans in the jar,
Enter your guess:
10
High Five! You got it, 7 guesses
Play again? y or n: y
See you later!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
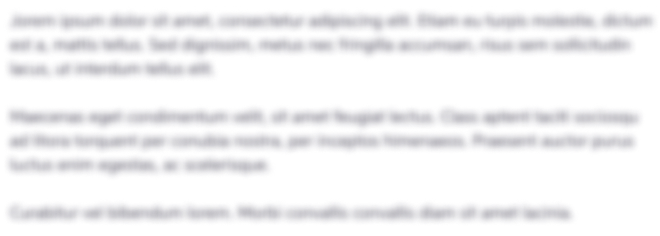
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started