Question
At this point you understand how structures work, what theyre used for, and how to manipulate arrays of them. This time, were going to make
At this point you understand how structures work, what theyre used for, and how to manipulate arrays of them. This time, were going to make things a bit more interesting by using a structure with many fields in it and manipulating them in a binary file instead of an array
Binary files are a great way to store structs, because the programmer doesnt have to deal with the hassle of coming up with tricky format strings for scanf()/printf(). All you need is a single fwrite()/fread() at the correct offset and you have the whole struct filled for you! The trade-off, of course, is that these files are not human-readable.
For this homework, were going to create a structure that will be used to represent the employees working in an office. There are lots of pieces of information for an employee that should be kept together in one group. The information to be maintained is as follows:
ID Number: this is an integer
First Name: this is an array of 20 characters
Last Name: this is an array of 20 characters
Title: this is an enum type corresponding to the employees title, which will be one of technician, manager, or salesperson.
Salary: this is a float (though in practice, representing monetary values with floating point numbers is a very bad idea)
Schedule: this is a two dimensional array of characters. The first (left) dimension represents the day of the workweek (M - F). The second dimension represents the hour of the day (8AM - 4PM). If the employee is busy during that hour, the corresponding array entry contains the character B, and if the employee is available during the hour, the array entry contains the character A
The structure should look like this (we combine it with a typedef so that we can refer to it with one name):
typedef struct {
int id_number;
char first_name[MAX_NAME_LEN];
char last_name[MAX_NAME_LEN];
enum title_t title;
float salary;
char schedule[N_DAYS][N_HOURS];
} employee_t;
employee_t read_employee(FILE *, int) {
This function will read the Nth employee record (where N is the second argument) from the file (the first argument), which has already been opened. The function should always return an employee_t structure.
If there is any kind of error, it should instead return BAD_EMPLOYEE, which is a structure that has its fields set to known invalid values. Errors include trying to read beyond the length of the file or any situation where fread() fails.
This function should assert that the file pointer is non-NULL and that N is greater than or equal to 0
}
I am confused and need help on how to do this Function, this is a C program.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
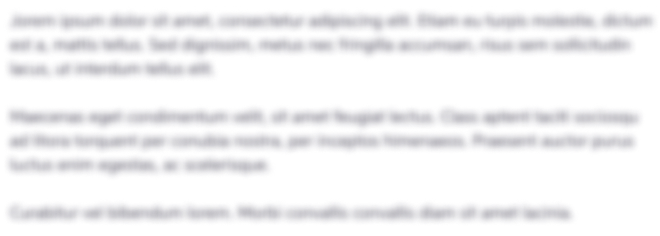
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started