Question
/** * * @author JP */ // Fig. 16.17: WordTypeCount.java // Program counts the number of occurrences of each word in a String. import java.io.IOException;
/**
*
* @author JP
*/
// Fig. 16.17: WordTypeCount.java
// Program counts the number of occurrences of each word in a String.
import java.io.IOException;
import java.nio.file.Paths;
import java.util.Map;
import java.util.HashMap;
import java.util.Set;
import java.util.TreeSet;
import java.util.Scanner;
public class TextCompare {
public static void main(String[] args) throws IOException {
// create HashMap to store String keys and Integer values
Map
Map
createMap(myMap, myMap2); // create map based on user input
displayMap(myMap, myMap2);
compareMap(myMap, myMap2);
}
// create map from user input
private static void createMap(Map
Scanner scan = new Scanner(System.in);
String txtfile = scan.next();
String txtfile2 = scan.next();
Scanner scanner = new Scanner(Paths.get(txtfile)); // create scanner
while (scanner.hasNext()){
String input = scanner.nextLine();
// tokenize the input
input = input.replaceAll("\\s+|(\\.|,|-|;|:|!|\\?|\"|\\[|\\]|\\(|\\)|'$|\ |\ )*(\\s)*(\\.|,|-|;|:|!|\\?|\"|\\[|\\]|\\(|\\)|'$|\ |\ )+(\\s)*"," ");
String[] tokens = input.split(" ");
// processing input text
for (String token : tokens) {
String word = token.toLowerCase(); // get lowercase word
// if the map contains the word
if (map.containsKey(word)) { // is word in map?
int count = map.get(word); // get current count
map.put(word, count + 1); // increment count
}
else {
map.put(word, 1); // add new word with a count of 1 to map
}
}
}
Scanner scanner2 = new Scanner(Paths.get(txtfile2)); // create scanner
while (scanner2.hasNext()){
String input = scanner2.nextLine();
// tokenize the input
input = input.replaceAll("\\s+|(\\.|,|-|;|:|!|\\?|\"|\\[|\\]|\\(|\\)|'$|\ |\ )*(\\s)*(\\.|,|-|;|:|!|\\?|\"|\\[|\\]|\\(|\\)|'$|\ |\ )+(\\s)*"," ");
String[] tokens2 = input.split(" ");
// processing input text
for (String token2 : tokens2) {
String word = token2.toLowerCase(); // get lowercase word
// if the map contains the word
if (map2.containsKey(word)) { // is word in map?
int count2 = map2.get(word); // get current count
map2.put(word, count2 + 1); // increment count
}
else {
map2.put(word, 1); // add new word with a count of 1 to map
}
}
}
}
private static void compareMap(Map
double first, second;
double jaccard;
Set
Set
// sort keys
TreeSet
TreeSet
first = sortedKeys.size()+sortedKeys2.size();
sortedKeys.retainAll(sortedKeys2);
second = first - sortedKeys.size();
jaccard = (sortedKeys.size())/(second);
if (jaccard < 0.5){
System.out.printf("%nIt is not a bad case. Similarity score = %.6f < threshold score 0.50000%n",jaccard);
}
else{
System.out.print("It is a bad case");
}
}
// display map content
private static void displayMap(Map
Set
Set
// sort keys
TreeSet
TreeSet
sortedKeys.retainAll(sortedKeys2);
System.out.printf("Number of unique words in text 1: %d%n", map.size());
System.out.printf("Number of unique words in text 2: %d", map2.size());
System.out.printf("%nThey have %d words in common.%n",sortedKeys.size());
System.out.printf("%nWord\t\tText 1 \tText 2 %n");
// generate output for each key in map
for (String key : sortedKeys) {
System.out.printf("%-14s%8s%8s%n", key, map.get(key), map2.get(key));
}
}
}
This is my code and I want to run this java with the cmd command line. But I do not know how to
The command line should be : 1.txt 2.txt which are the given documents
Step by Step Solution
There are 3 Steps involved in it
Step: 1
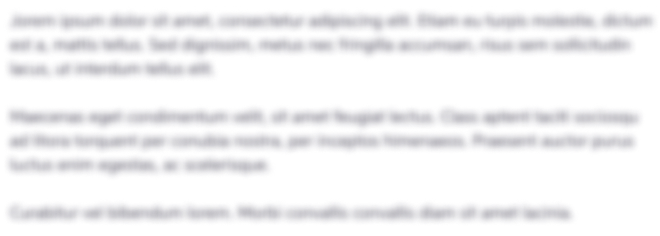
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started