Answered step by step
Verified Expert Solution
Question
1 Approved Answer
AUTOMOBILE CLASS: /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates







AUTOMOBILE CLASS:
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
* An Automobile
* @author kerlin
*/
public class Auto
{
private String plate;
private Color color;
private boolean hasPermit;
/**
* Default Auto
*/
public Auto()
{
plate = "N/A";
color = Color.Unknown;
hasPermit = false;
}
/**
* Full parameter Constructor
* @param inPlate String with Plate Data
* @param inColor String with Color Data
* @param inHasPermit boolean: True for has permit, False for not having permit
*/
public Auto(String inPlate, String inColor, boolean inHasPermit)
{
setPlate(inPlate);
setColor(inColor);
hasPermit = inHasPermit;
}
/**
* Describes the Auto
* @return a String with the Auto's data
*/
@Override
public String toString()
{
return "Auto{" + "plate=" + plate + ", color=" + color + ", hasPermit=" + hasPermit + '}';
}
/**
* Get the Auto's license plate data
* @return a String with License Plate data
*/
public String getPlate()
{
return plate;
}
/**
* Set the Auto's license plate data
* @param inPlate a String to use for the Auto's License Plate data
*/
public void setPlate(String inPlate)
{
if (inPlate.length() > 0 && inPlate.length()
plate = inPlate;
else
System.out.println("Attempted to set plate to a String too long or too short!");
}
/**
* Get the color of the Auto
* @return a String containing the color information for the Auto
*/
public Color getColor()
{
return color;
}
/**
* Set the color of the Auto
* @param inColor a String denoting the color of the Auto
*/
public void setColor(String inColor)
{
Color[] validColors = Color.values();
boolean foundMatch = false;
//Check the elements/values in the array
for (int index = 0; index
{
if (inColor.equals( validColors[index].toString() ))
{
foundMatch = true; //We found a match!!!
}
}
inColor = inColor.replaceAll(" ", "");//Removing Spaces!
//foundMatch is true ONLY IF we found a matching enum value
if (foundMatch)
{
color = Color.valueOf(inColor);
}
else
{
color = Color.Unknown;
}
}
/**
* Check if the Auto has a permit
* @return a true if Auto has a permit and a false if Auto does NOT have a permit
*/
public boolean isHasPermit()
{
return hasPermit;
}
/**
* Sets the Auto's Permit status
* @param inPermit a boolean denoting if the Auto should (true) or should not (false) have a permit
*/
public void setHasPermit(boolean inPermit)
{
hasPermit = inPermit;
}
public boolean equals(Object otherObject)
{
//Check if the other object is even an Auto
if (!(otherObject instanceof Auto))
{
return false; //Since it's not even a Auto!
}
else
{
// Your logic for figuring out if the objects should match goes here!
if (((Auto) otherObject).getPlate().equals(getPlate()) && ((Auto) otherObject).getColor().equals(getColor()) && ((Auto) otherObject).isHasPermit() == (isHasPermit()))
{
return true;
}
else
{
return false; // At least 1 attribute is different
}
}
}
}
BICYCLE CLASS:
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
* A Bicycle Object
* @author kerlin
*/
public class Bicycle
{
private String serialNumber;
private Brand brand;
private boolean locked;
/**
* Default Bicycle
*/
public Bicycle()
{
serialNumber = "N/A";
brand = Brand.Unknown;
locked = false;
}
/**
* Full parameter Bicycle constructor
* @param inSerialNumber String with Serial Number Data
* @param inBrand String with Brand Data
* @param inLocked boolean: True if bike is locked, False if bike is not locked
*/
public Bicycle(String inSerialNumber, String inBrand, boolean inLocked)
{
setSerialNumber(inSerialNumber);
setBrand(inBrand);
locked = inLocked;
}
/**
* Describes the Bicycle
* @return a String with the Bicycle's data
*/
@Override
public String toString()
{
return "Bicycle{" + "serialNumber=" + serialNumber + ", brand=" + brand + ", locked=" + locked + '}';
}
/**
* The Bicycle's serial number
* @return a String containing the Bicycle's serial number
*/
public String getSerialNumber()
{
return serialNumber;
}
/**
* Change the Bicycle's serial number
* @param inSerialNumber a String to set as the Bicycle's serial number
*/
public void setSerialNumber(String inSerialNumber)
{
if ( inSerialNumber.length() > 2 && inSerialNumber.length() his week we are focusing on arrays We will be adding a ParkingLot to our simulation ParkingLot: This object should have AT LEAST 2 attributes: o An array of ParkingSpace objects o A value to denote how many of the available ParkingSpaces in the ParkingLot are full (MUST BE a percentage!) Required methods o A constructor method which: Takes in an integer to denote how many Auto ParkingSpaces to create in the ParkingLot - Takes in an integer to denote how many Bicycle ParkingSpaces to create in the ParkingLot Updates the ParkingLots array attribute to reflect this input o toString) method: Should create and return a String with information about Number of total
serialNumber = inSerialNumber;
else
System.out.println("Attempted to set serial number to something too small or too large");
}
/**
* Get the type of Bicycle
* @return a String with the brand of the Bicycle
*/
public Brand getBrand()
{
return brand;
}
/**
* Set the type of Bicycle
* @param inBrand a String with the brand info for the Bicycle
*/
public void setBrand(String inBrand)
{
Brand[] validBrands = Brand.values();
boolean foundMatch = false;
//Check the elements/values in the array
for (int index = 0; index
{
if (inBrand.equals( validBrands[index].toString() ))
{
foundMatch = true; //We found a match!!!
}
}
inBrand = inBrand.replaceAll(" ", "");//Removing Spaces!
//foundMatch is true ONLY IF we found a matching enum value
if (foundMatch)
{
brand = Brand.valueOf(inBrand);
}
else
{
brand = Brand.Unknown;
}
}
/**
* Check if the Bicycle is locked or not
* @return a boolean with data on if the Bicycle is locked (true) or not (false)
*/
public boolean isLocked()
{
return locked;
}
/**
* Set the locked status of the Bicycle
* @param lock a boolean which locks (true) or unlocks (false) a Bicycle
*/
public void setLocked(boolean lock)
{
locked = lock;
}
public boolean equals(Object otherObject)
{
if (!(otherObject instanceof Bicycle))
{
return false; //Since it's not even a Bicycle!
}
else
{
if (((Bicycle) otherObject).getSerialNumber().equals(getSerialNumber()) && ((Bicycle) otherObject).getBrand().equals(getBrand()) && ((Bicycle) otherObject).isLocked() == (isLocked()))
{
return true;
}
else
{
return false; // At least 1 attribute is different
}
}
}
}
BRAND CLASS:
import java.util.Random;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
* Bike Brands
* @author kerlin
*/
public enum Brand
{
Unknown, Schwinn, Huffy, Aero;
public static Brand randomBrand()
{
Brand[] myBrands = Brand.values();
Random gen = new Random();
int index = gen.nextInt((myBrands.length -1)) + 1;
return myBrands[index];
}
public String toString()
{
String base = super.toString();
String output = "";
boolean firstChar = true;
for (int index = 0; index
{
//Check for uppercase
if (Character.isUpperCase( base.charAt(index) ) && !firstChar)
{
output = output + " ";
}
output = output + base.charAt(index);
firstChar = false;
}
return output;
}
}
COLOR CLASS:
import java.util.Random;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
* Colors
* @author kerlin
*/
public enum Color
{
Unknown, Red, Orange, Pink, Black;
public static Color randomColor()
{
Color[] myColors = Color.values();
Random gen = new Random();
int index = gen.nextInt((myColors.length -1)) + 1;
return myColors[index];
}
public String toString()
{
String base = super.toString();
String output = "";
boolean firstChar = true;
for (int index = 0; index
{
//Check for uppercase
if (Character.isUpperCase( base.charAt(index) ) && !firstChar)
{
output = output + " ";
}
output = output + base.charAt(index);
firstChar = false;
}
return output;
}
}
DRIVER MAINCLASS:
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
* This code tests Auto (and eventually you should test Bicycle and ParkingSpace)
* @author kerlin
*/
public class Driver
{
/**
* @param args the command line arguments
*/
public static void main(String[] args)
{
//Create variables for Auto attributes
String color = "Red";
String plate = "123 ABC";
boolean permitStatus = true;
//Create an Auto Object
Auto myAuto = new Auto(plate, color, permitStatus);
//Test Gets
System.out.println("Auto color was set to: " + color);
System.out.println("The color stored in/by the Auto is: " + myAuto.getColor());
System.out.println("Auto plate was set to: " + plate);
System.out.println("The plate info stored in/by the Auto is: " + myAuto.getPlate());
System.out.println("Auto permit status was set to: " + permitStatus);
System.out.println("The permit status stored in/by the Auto is: " + myAuto.isHasPermit());
//Create variables for Bicycle attributes
String serial = "BMX123";
String brand = "Schwinn";
boolean lockStatus = true;
//Create an Bicycle Object
Bicycle myBicycle = new Bicycle(serial, brand, lockStatus);
//Test Gets
System.out.println("Bicycle serial number was set to: " + serial);
System.out.println("The serial stored in/by the Bicycle is: " + myBicycle.getSerialNumber());
System.out.println("Bicycle brand was set to: " + brand);
System.out.println("The brand info stored in/by the Bicycle is: " + myBicycle.getBrand());
System.out.println("Bicycle lock status was set to: " + lockStatus);
System.out.println("The lock status stored in/by the Bicycle is: " + myBicycle.isLocked());
//Create variables for ParkingSpace attributes
String vehicleType = "Car";
boolean occupied = false;
int number = 24;
//Create an ParkingSpace Object
ParkingSpace myParkingSpace = new ParkingSpace(occupied, number, vehicleType);
//Test Gets
System.out.println("This Parking Space should hold: " + vehicleType + " objects.");
System.out.println("The Parking Space holds: " + myParkingSpace.getType());
System.out.println("This Parking Space should be occupied: " + occupied);
System.out.println("The Parking Space is: " + myParkingSpace.isOccupied());
System.out.println("This Parking Space should be number: " + number);
System.out.println("The Parking Space number is: " + myParkingSpace.getNumber());
// TODO code application logic here
}
}
PARKINGSPACE CLASS:
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
* A Parking Space object
* @author kerlin
*/
public class ParkingSpace
{
private boolean occupied;
private int number;
private ValidParkingObject type;
/**
* Default Parking Space
*/
public ParkingSpace()
{
occupied = false;
number = -13;
type = ValidParkingObject.Unknown;
}
/**
* Full parameter ParkingSpace constructor
* @param inOccupied boolean: True if occupied, False if not occupied
* @param inNumber int with the Parking Space's number
* @param inType String noting the type of object which can park in this Parking Space
*/
public ParkingSpace(boolean inOccupied, int inNumber, String inType)
{
occupied = inOccupied;
number = inNumber;
setType(inType);
}
/**
* Describes the Parking Space
* @return a String with the Parking Space's data
*/
@Override
public String toString()
{
return "ParkingSpace{" + "occupied=" + occupied + ", number=" + number + ", type=" + type + '}';
}
/**
* Try to park an Auto in this Parking Space
* @param inAuto the Auto try to park here
* @return a boolean noting if the Auto was able to park (true) or not (false)
*/
public boolean parkAuto(Auto inAuto)
{
if (isOccupied())
{
return false;
}
else if (getType().equals(ValidParkingObject.Auto))
{
occupied = true;
return true;
}
else
return false;
}
/**
* Try to park a Bicycle in this Parking Space
* @param inBike the Bicycle to try parking here
* @return a boolean noting if the Bicycle was able to park (true) or not (false)
*/
public boolean parkBicycle (Bicycle inBike)
{
if (isOccupied())
{
return false;
}
else if (getType().equals(ValidParkingObject.Bicycle))
{
occupied = true;
return true;
}
else
return false;
}
/**
* Check if the Parking Space is occupied or not
* @return a boolean which is true if the Parking Space is in use and false if the Parking Space is not in use
*/
public boolean isOccupied()
{
return occupied;
}
/**
* Get the ParkingSpace number
* @return an int with the ParkingSpace's number
*/
public int getNumber()
{
return number;
}
/**
* Get the type of ParkingSpace
* @return a String denoting what type of Object is allowed to park in this ParkingSpace
*/
public ValidParkingObject getType()
{
return type;
}
/**
* Set the type of Parking Space
* @param inType a String to note what type of Object is allowed to park in this Parking Space
*/
public void setType(String inType)
{
ValidParkingObject[] validTypes = ValidParkingObject.values();
boolean foundMatch = false;
//Check the elements/values in the array
for (int index = 0; index
{
if (inType.equals( validTypes[index].toString() ))
{
foundMatch = true; //We found a match!!!
}
}
inType = inType.replaceAll(" ", "");//Removing Spaces!
//foundMatch is true ONLY IF we found a matching enum value
if (foundMatch)
{
type = ValidParkingObject.valueOf(inType);
}
else
{
type = ValidParkingObject.Unknown;
}
}
public boolean equals(Object otherObject)
{
if (!(otherObject instanceof ParkingSpace))
{
return false; //Since it's not even a ParkingSpace!
}
else
{
if (((ParkingSpace) otherObject).getNumber() == (getNumber()) && ((ParkingSpace) otherObject).getType().equals(getType()) && ((ParkingSpace) otherObject).isOccupied() == (isOccupied()))
{
return true;
}
else
{
return false; // At least 1 attribute is different
}
}
}
}
VALIDPARKINGOBJECT CLASS:
import java.util.Random;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
*
* @author kerlin
*/
public enum ValidParkingObject
{
Unknown, Auto, Bicycle;
public static ValidParkingObject randomValidParkingObject()
{
ValidParkingObject[] myValidParkingObjects = ValidParkingObject.values();
Random gen = new Random();
int index = gen.nextInt((myValidParkingObjects.length -1)) + 1;
return myValidParkingObjects[index];
}
public String toString()
{
String base = super.toString();
String output = "";
boolean firstChar = true;
for (int index = 0; index
{
//Check for uppercase
if (Character.isUpperCase( base.charAt(index) ) && !firstChar)
{
output = output + " ";
}
output = output + base.charAt(index);
firstChar = false;
}
return output;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
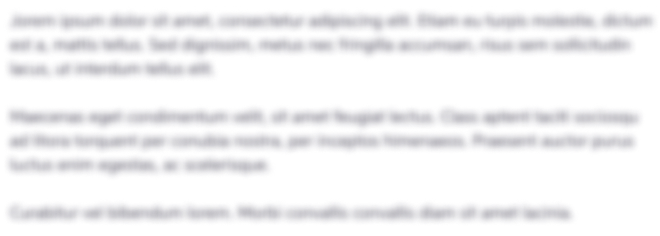
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started