Question
Background (C++) Create a loop which prompts the user for an item purchased. Continue to process input lines until the user inputs DONE. Each line
Background (C++)
Create a loop which prompts the user for an item purchased. Continue to process input lines until the user inputs DONE. Each line has a description of an item followed by a comma, then the number of items purchased, the price per item, and an optional note. You may assume that there are no errors in the input and that the user uses the format shown. Following is an example of program input:
Tacos al Pastor, 3 2.25 Spicy but small Nachos with Carnitas, 5 1.50 Chiles Rellenos, 4 4.99 Very tasty! DONE
The output for the above input sequence would be:
Item: Tacos al Pastor, 3 2.25 Spicy but small Item: Nachos with Carnitas, 5 1.50 Item: Chiles Rellenos, 4 4.99 Very tasty! Item: Item Quantity Price Total Cost Tacos al Pastor 3 $2.25 $6.75 Nachos with Carnitas 5 $1.50 $7.50 Chiles Rellenos 4 $4.99 $19.96 Total Invoice Cost: $34.21
Observe that the optional notes are thrown away and you calculate some pricing.
Implementation
The purpose of this lab is to learn about string streams. The ostringstream is very common and useful and you will use it in future labs. The istringstream is used less but can still be great in certain situations. For this lab the input starts with an arbitrary length "item" and ends with an arbitrary length "note," with an int and a double in the middle. If you used cin you would have to read everything in one word at a time. getline() is convenient to bring in the entire line at once. The problem with getline() though is that now everything is part of a string including the int and double which are a hassle to get into their proper type. If we had used cin >> price at just the right time it would have automatically put the value into a double. By using istringstream you can get the best of both worlds.
Use a getline() to enter each line and put it in a string variable.
Hint: Use substring to extract the "item" part of the string and then put the remaining part of the string into an istringstream. Then you can use the extraction operator (>>) to extract the following values directly to their proper variable types.
Create the final output string "on the fly" using an ostringstream at the same time as you are parsing each line of input. Make sure that you use iomanip with the ostringstream in order to do proper formatting of widths, precisions, etc. This means you cannot store the output data in an array, vector or any data structure. It just gets inserted (<<) into the ostringstream and made into a string at the very end, like you did in challenge activity 7.1.3.
Left justify and use a width of 30 for the Item, 10 for quantity, 10 for price, and 12 for total cost.
After the loop terminates, you will add the last two lines to the ostringstream, create a string variable with the content of the ostringstream, and then output the string. You may only use a single cout and a single string variable to output the entire final output table. That single string should already be formatted correctly and include endlines, etc. The only other cout in your program will be cout << "Item: " and "endl" in your loop.
This prep lab will help you see the power of stringstreams. Note that rather than output the string variable, you could have passed it as a parameter to a function, or anything else you can do with a string variable.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
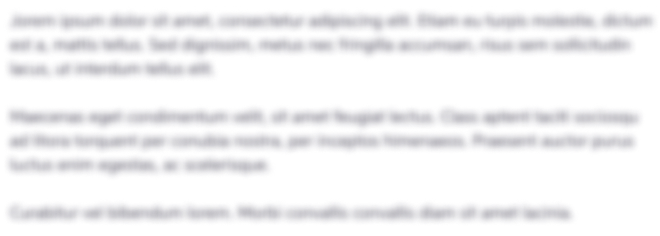
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started