Question
Background The Game of Life was invented by John Conway, who is currently a professor of mathematics at Princeton. Its not a game in the
Background The Game of Life was invented by John Conway, who is currently a professor of mathematics at Princeton. Its not a game in the traditional sense, but rather a grid of cells that changes over time according to a few simple rules.
At a given point in time, each cell in the grid is either alive (represented by a value of 1) or dead (represented by a value of 0). The neighbors of a cell are the cells that immediately surround it in the grid.
Over time, the grid is repeatedly updated according to the following five rules:
All cells on the outer boundary of the grid remain fixed at 0.
An inner cell that has fewer than 2 alive neighbors dies (because of loneliness).
An inner cell that has more than 3 alive neighbors dies (because of overcrowding).
An inner cell that is dead and has exactly 3 alive neighbors comes to life.
All other cells maintain their state.
Although these rules seem simple, they give rise to complex and interesting patterns! You can find more information and a number of interesting patterns here.
Getting Started In the ps7twoD folder that you used for Problem 3, you should find a file named ps7pr4.py. (Note that this is not the same file that you used for Problem 3.) Open ps7pr4.py in IDLE, and put your work for this problem in that file. Once again, you should not move any of the files out of the ps7twoD folder.
IMPORTANT: We have included an import statement at the top of ps7pr4.py that imports all of the functions from your ps7pr3.py file. Therefore, you will be able to call any of the functions that you wrote for Problem 3.
Your tasks
Write a function count_neighbors(cellr, cellc, grid) that returns the number of alive neighbors of the cell at position [cellr][cellc] in the specified grid. You may assume that the indices cellr and cellc will always represent one of the inner cells of grid, and thus the cell will always have eight neighbors.
Here are two possible approaches that you could take to this problem:
first approach: You could use nested loops to compute the necessary cumulative sum. Set the ranges of the two loops so that you end up examining only the specified cell and its eight neighbors. And although these loops will end up including the specified cell, you should make sure that you dont actually count the cell itself as one of its own neighbors!
OR
second approach: You could write eight separate if statements to check each of the eight neighbors. If you take this approach, you will not need to use any loops.
To test your function, run the file in IDLE and enter the following from the Shell:
>>> grid1 = [[0,0,0,0,0], [0,0,1,0,0], [0,0,1,0,0], [0,0,1,0,0], [0,0,0,0,0]] >>> print_grid(grid1) 00000 00100 00100 00100 00000 >>> count_neighbors(2, 1, grid1) # grid1[2][1] has 3 alive neighbors 3 >>> count_neighbors(2, 2, grid1) # we don't count the cell itself! 2 >>> count_neighbors(1, 2, grid1) 1 >>> grid2 = [[0,0,0,0,0,0], [0,0,1,1,0,0], [0,1,1,1,0,0], [0,0,1,0,1,0], [0,0,1,0,1,0], [0,0,0,0,0,0]] >>> count_neighbors(2, 2, grid2) # grid2[2][2] has 5 alive neighbors 5 >>> count_neighbors(2, 3, grid2) # so does grid2[2][3] 5 >>> count_neighbors(3, 3, grid2) # grid2[3][3] has 6 alive neighbors 6
Now lets implement the rules of the Game of Life!
A given configuration of the grid in the Game of Life is known as a generation of cells. Write a function next_gen(grid) that takes a 2-D list called grid that represents the current generation of cells, and that uses the rules of the Game of Life (see above) to create and return a new 2-D list representing the next generation of cells.
Notes/hints:
Begin by creating a copy of grid (call it new_grid). Use one of the functions that you wrote for the previous problem!
Limit your loops so that they never consider the outer boundary of cells. You did this already in one of the functions that you wrote for the previous problem.
You can use the count_neighbors() function that you just wrote. Make sure that you count the neighbors in the current generation (grid) and not the new one (new_grid).
When updating a cell, make sure to change the appropriate element of new_grid and not the element of grid.
Testing next_gen Here are two patterns that you might want to take advantage of when testing your function:
If a 3x1 line of alive cells is isolated in the center of a 5x5 grid, the line will oscillate from vertical to horizontal and back again, as shown below:
>>> grid1 = [[0,0,0,0,0], [0,0,1,0,0], [0,0,1,0,0], [0,0,1,0,0], [0,0,0,0,0]] >>> print_grid(grid1) 00000 00100 00100 00100 00000 >>> grid2 = next_gen(grid1) >>> print_grid(grid2) 00000 00000 01110 00000 00000 >>> grid3 = next_gen(grid2) >>> print_grid(grid3) 00000 00100 00100 00100 00000
and so on!
In a 4x4 grid, if the inner cells are all alive, they should remain alive over time:
>>> grid1 = [[0,0,0,0], [0,1,1,0], [0,1,1,0], [0,0,0,0]] >>> print_grid(grid1) 0000 0110 0110 0000 >>> grid2 = next_gen(grid1) >>> print_grid(grid2) 0000 0110 0110 0000
Optional (but encouraged): graphical life! We have given you functions that you can use to run a graphical version of the Game of Life. Heres an example of how to use it with a random starting configuration:
>>> grid = random_grid(15, 15) >>> show_graphics(grid) # run the Game of Life in a graphics window
The following key presses can be used to control the simulation:
Enter (or Return): begin/resume the simulation
P: pause the simulation
Spacebar: clear the grid
Q: quit the simulation
When the simulation is paused, you should be able to change the state of a cell by clicking on it.
Trying other patterns The Life Lexicon is a website that includes many examples of interesting starting patterns. They use a different representation for the cells in a grid: a dot . character for a dead cell and an O character for an alive cell. For example, here is a well-known pattern known as the Gosper glider gun:
........................O........... ......................O.O........... ............OO......OO............OO ...........O...O....OO............OO OO........O.....O...OO.............. OO........O...O.OO....O.O........... ..........O.....O.......O........... ...........O...O.................... ............OO......................
You can easily try a pattern that is specified in Life Lexicon form by using the read_patternfunction. It takes 2 inputs specifying the amount of padding (i.e., the number of empty rows and empty columns) that should be added around a pattern that is entered by the user. When you call this function, it waits for you to enter a pattern in the form found in the Life Lexicon, and it converts it into a 2-D list of the form that our functions use.
For example:
>>> grid = read_pattern(20, 20) # use a padding of 20 on all sides enter the pattern: ........................O........... ......................O.O........... ............OO......OO............OO ...........O...O....OO............OO OO........O.....O...OO.............. OO........O...O.OO....O.O........... ..........O.....O.......O........... ...........O...O.................... ............OO......................
When you are prompted to enter the pattern, copy and paste it into the Shell window and hit Enter. (Its not a problem if you have extra spaces at the start of some of the lines when you paste the pattern.) You can then run the pattern graphically as indicated above.
Colors You can also change the colors used for the alive and dead cells. For example, to invert the default color schemeproducing something that is more obviously Terrier-themed!you can do the following from the Shell before calling show_graphics:
>>> set_color(0, 'red') >>> set_color(1, 'white')
A full list of color names is available here.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
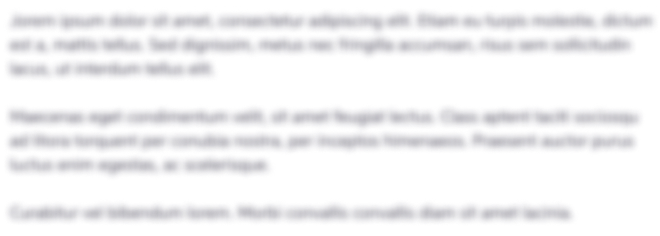
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started