Question
Background You have been asked to develop the logic for a version of a card game that has aspects similar to the popular card game
Background
You have been asked to develop the logic for a version of a card game that has aspects similar to the popular card game Uno. The working title for the game is Quattro (as it has four players).
Game Materials:
There are 40 cards in the pack in total
Cards can be of: four different colours Red, Yellow, Green, Blue; and have a number value between 0 9
The game is played with four players.
Rules for Gameplay
1. Each player receives a set number of cards to play (for this game players will receive three cards each). These are referred to as the player hand.
2. The remaining cards are placed in a draw pile.
3. The first card in the draw pile is placed in the discard pile and its colour and number revealed. It is now the turn of the first player.
4. The player checks the cards in their hand to see if any match against the top card of the discard pile in terms of: a. The numbers of the two cards b. The colours of the two cards
5. If they have a match on colour or number, the player removes the matching card from their hand, and it becomes the first (top) card in the discard pile.
6. If they do not have a match, they need to instead take the top card from the draw pile and add it to their hand.
7. Play then moves onto the next player, who works their way through steps 4-6
8. Play continues until one player has no cards remaining. This player is declared the winner of the game. There are numerous online videos of people playing Uno which is a more complicated version of the game we are developing. Search People Playing Uno and check out a couple to get a better idea of gameplay in action if you are unsure of the game mechanics. Note that unlike Uno we are not including any Wild, Draw Two, Reverse, or Skip cards into our game. Also review Sample Gameplay below for a description of how a game may play out
Items to note in relation to the solution:
This is a text based game only i.e. you do not have to implement any imagery of cards.
You can complete the solution however you like, within the following boundaries
o You must use the class structure as outlined in the Class Diagram below
o You need to include the functionality as outlined Task 2 below
o You must complete all Tasks below
Class Diagram
You have done some preliminary work and come up with the following class diagram. Note the following in relation to this diagram
The classes with a blue background are active classes in this project. They will contain code for the solution that you are creating
The classes with an orange background are part of a proposed future expansion of the game. They need to be implemented but only as a shell (i.e. they will contain no code towards your current solution)
Tasks
Task 1:
Program Design Create a new NetBeans 8.2 Java 8 project called QuattroTest. Add the eight classes from the UML class diagram in a package called quattrotest as shown below.
Create a class stub for each class such as below:
public class ClassName {
}
Add the necessary additional keywords for classes that inherit from interfaces or abstract classes
This should also be done for classes that do not have current functionality (as highlighted in the diagram above)
Task 2: Functionality
It is expected that your solution will implement the following functionality (check Rules for Gameplay under Background for more context on the functionality.
Check as well the Suggested Tips for Implementing for Ideas
Functionality to Implement:
The card game has four players
Only one of the players is a human player (the remaining three players are bots)
The program will ask for the human player to give their name.
Bot players should have a name as well. This should be given to them by the program.
The deck of cards will consist of forty cards in total made up of: o 10 Blue cards numbered 0-9 o 10 Yellow cards numbered 0-9 o 10 Red cards numbered 0-9 o 10 Green cards numbered 0-9
The order of any collection of cards must be randomized (i.e. the cards need to be shuffled)
Each player will be dealt three cards initially from the deck (or draw pile)
The human (and bot) players will be able to view what cards they have in their hand
The human (and bot) players will not be able to view of what cards opponents have.
Once cards have been distributed to players from the draw pile, the first card from this pile is moved to become the first/top card in the discard pile.
A player needs to match the top card of the discard pile with a card they have in their hand. o If they have a matching card, it is selected and is then moved from their hand to become the top card of the discard pile. Play then moves onto the next player o If they do not have a matching card, the first card from the draw pile needs to be selected and added to their hand. Play then moves onto the next player
Each player should have their turn in order
Once any player has played their last card a message should be displayed indicating that this player is the winner of the game. The game should end at this point.
The human player should be asked at the start of the game what their name is
The human player should be provided with the following information during the game where appropriate o Their name o What cards they hold in their hand o What the top card of the discard pile is o What cards other players played o If other players had to select a card from the draw pile o How many cards the other players have in their hands
The human player should have some means of indicating which card they would like to play via the console
If the human does not enter details of a valid card to play, they should be advised to enter details again
If the human does not have a valid card to play, they should be able to indicate this via the console
If the draw pile is exhausted (i.e. there are no more cards in this pile) o The top card of the discard pile should be retained in the discard pile o All other cards in the discard pile should be moved to the draw pile and randomized (shuffled).
Suggested tips for implementing:
Give each of the cards a code to identify it i.e. Red 5 could be given the code R5 or even just a number like 16. What matters is that the code is unique. This code could then be used for the human player to indicate which card they would like to play (along with other functionality)
Consider making use of Arraylists for the following reasons:
o Arrays lists can be used to manage cards in the following locations Hold cards in the deck or draw pile Hold cards in the discard pile Hold the cards for individual players (i.e. each player has their own arraylist of cards)
o Arraylists can be used to move cards i.e. A player that has a valid card to play can have that card moved from their personal arraylist of cards to the top of the discard arraylist i.e. a player than has no valid card to play can
o Arraylists can have their contents randomized through the function Collections.shuffle(arraylistname) available through importing java.util.*
Use the QuattroTest class to run and test your program frequently. It may be that much of the logic that you write for the program ends up in this class.
Start by creating the deck of cards making use of the StandardColourCard class. As suggested above consider adding the created cards to an arraylist, and develop logic to move cards between a deck and draw pile as required by the game
From there, add in a player (Using the Player class), and develop the logic to move cards between the player hand, and the deck and draw piles
From there add in the logic to manage the three bot players and player turns.
Note the above are suggestions only so long as all functionality is included and all directions in Tasks 1-4 are followed you can create the program how you wish.
Task 03 Exceptions
You will need to run some checks on the validity of data been entered. It is expected that the program will throw an exception in the following circumstances:
If no name is entered for the human player of the game
If the human player makes an invalid selection when entering details of a card to play You must have a catch block that catches these exceptions and displays an appropriate error message. (You can use the getMessage method of the Throwable class to achieve this).
You do need to manage the exceptions appropriately i.e. the program should alert the user that an exception has occurred, and advise the user how to proceed.
Task 04 - Comments
Ensure you include appropriate comments in your code including:
Purpose of classes
Purpose of methods
Explanatory notes for any potentially confusing items
Task 05: Testing
Testing Tasks
As part of your program implementation you should test to determine how the program performs when the user makes an invalid selection when choosing a card to play (refer Task 03)
1. What is white box testing and how would you use it to test this feature
2. What is black box testing and how would you use it to test this feature.
3. Describe one of the error scenarios that could unfold if a user does enter incorrect data.
4. Design a custom Java Class to represent a custom exception for Q3. Your solution must follow best practices from Javas Exception class hierarchy including:
A default constructor
A one-time argument with a message
The getMessage() accessor method.
Note there is no need to implement this custom exception class
Key: Fully Implemented Card (Abstract) Partially Implemented Colour Card Wild Card Player Standard Colour Card Special Colour Card Special Ability (Interface) Quattro Test Projects X Files Services QuattroTest Source Packages quattrotest Card.java ColourCard.java Player.java QuattroTest.java SpecialAbility.java Special ColourCard.java Standard ColourCard.java WildCard.java LibrariesStep by Step Solution
There are 3 Steps involved in it
Step: 1
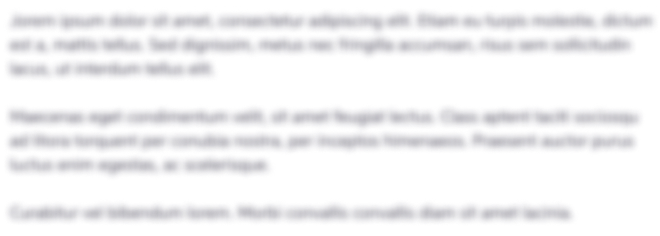
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started