Question
Below attached you will find a file from Programming Challenge 5 chapter 6 with required you to write a Payroll class that calculates an employees
Below attached you will find a file from Programming Challenge 5 chapter 6 with required you to write a Payroll class that calculates an employees payroll. Write exception classes for the following error conditions:
An empty string is given for the employees name.
An invalid value is given to the employees ID number. If you implemented this field as a string, then an empty string could be invalid. If you implemented this field as a numeric variable, then a negative number or zero would be invalid.
An invalid number is given for the number of hours worked. This would be a negative number or a number greater than 84.
An invalid number is given for the hourly pay rate. This would be a negative number or a number greater than 25.
Modify the Payroll class so that it throws the appropriate exception when any of these errors occur. Demonstrate the exception classes in a program.
1 /** 2 Payroll class 3 Chapter 6, Programming Challenge 5 4 */ 5 6 public class Payroll 7 { 8 private String name; // Employee name 9 private int idNumber; // ID number 10 private double payRate; // Hourly pay rate 11 private double hoursWorked; // Number of hours worked 12 13 /** 14 The constructor initializes an object with the 15 employee's name and ID number. 16 @param n The employee's name. 17 @param i The employee's ID number. 18 */ 19 20 public Payroll(String n, int i) 21 { 22 name = n; 23 idNumber = i; 24 } 25 26 /** 27 The setName sets the employee's name. 28 @param n The employee's name. 29 */ 30 31 public void setName(String n) 32 { 33 name = n; 34 } 35 36 /** 37 The setIdNumber sets the employee's ID number. 38 @param i The employee's ID number. 39 */ 40 41 public void setIdNumber(int i) 42 { 43 idNumber = i; 44 } 45 46 /** 47 The setPayRate sets the employee's pay rate. 48 @param p The employee's pay rate. 49 */ 50 51 public void setPayRate(double p) 52 { 53 payRate = p; 54 } 55 56 /** 57 The setHoursWorked sets the number of hours worked. 58 @param h The number of hours worked. 59 */ 60 61 public void setHoursWorked(double h) 62 { 63 hoursWorked = h; 64 } 65 66 /** 67 The getName returns the employee's name. 68 @return The employee's name. 69 */ 70 71 public String getName() 72 { 73 return name; 74 } 75 76 /** 77 The getIdNumber returns the employee's ID number. 78 @return The employee's ID number. 79 */ 80 81 public int getIdNumber() 82 { 83 return idNumber; 84 } 85 86 /** 87 The getPayRate returns the employee's pay rate. 88 @return The employee's pay rate. 89 */ 90 91 public double getPayRate() 92 { 93 return payRate; 94 } 95 96 /** 97 The getHoursWorked returns the hours worked by the 98 employee. 99 @return The hours worked. 100 */ 101 102 103 public double getHoursWorked() 104 { 105 return hoursWorked; 106 } 107 108 /** 109 The getGrossPay returns the employee's gross pay. 110 @return The employee's gross pay. 111 */ 112 113 public double getGrossPay() 114 { 115 return hoursWorked * payRate; 116 } 117 } 118
Step by Step Solution
There are 3 Steps involved in it
Step: 1
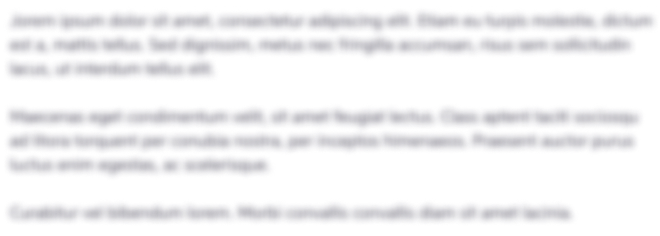
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started