Question
below is a program with a Card class, Deck class, Hand class. i have to make a hand of 13 cards and put them in
below is a program with a Card class, Deck class, Hand class.
i have to make a hand of 13 cards and put them in rank order within their suit order.
i continue to print the same card 13 times and cannot figure out how to get 13 different cards from the new deck
public class Card {
private int rank; private int suit;
/** * Constructor for Card class * * @param rank : value from 2-14 * @param suit : all four suits */ public Card(int rank, int suit) { this.rank = rank; this.suit = suit; }
/** * This returns the rank of the Card object * * @return */ public int getRank() { return rank; }
/** * @return the suit of the Card object */ public int getSuit() { return suit; }
/** * @return applies the correct rank and suit as a String instead of integers */ @Override public String toString() { String properRank = ""; String properSuit = "";
if (2 <= this.rank || this.rank <= 10) { properRank = String.valueOf(rank); } if (this.rank == 11) { properRank = "J"; } if (this.rank == 12) { properRank = "Q"; } if (this.rank == 13) { properRank = "K"; } if (this.rank == 14) { properRank = "A"; }
if (this.suit == 1) { properSuit = "\u2660"; //Spade } if (this.suit == 2) { properSuit = "\u2665"; //Heart } if (this.suit == 3) { properSuit = "\u2663"; //Club } if (this.suit == 4) { properSuit = "\u2666"; //Diamond }
return properRank + properSuit; } }
.----------------------------------------------------------------
import java.util.ArrayList; import java.util.Random;
public class Deck {
private ArrayList ArrayListOfCard;
public Deck() { ArrayListOfCard = new ArrayList<>();
for (int a = 1; a <= 4; a++) { for (int b = 2; b <= 14; b++) { ArrayListOfCard.add(new Card(b, a)); } } }
public Card topCard() { Card topCard = ArrayListOfCard.get(0); return topCard; }
public void Shuffle() { Random generator = new Random(); int shuffNum = generator.nextInt(100);
for (int x = 0; x < shuffNum; x++) {
for (int y = 0; y < ArrayListOfCard.size(); y++) { int randIndex = generator.nextInt(ArrayListOfCard.size()); Card remCard = ArrayListOfCard.get(randIndex); Card yCard = ArrayListOfCard.get(y); ArrayListOfCard.remove(y); ArrayListOfCard.remove(randIndex); ArrayListOfCard.add(randIndex, yCard); ArrayListOfCard.add(y, remCard); } } } }
-----------------------------------------------------------------------------
import java.util.ArrayList;
public class Hand {
private ArrayList ArrayListOfCard; ArrayList ArrayListOfSpade = new ArrayList<>(); ArrayList ArrayListOfHeart = new ArrayList<>(); ArrayList ArrayListOfClub = new ArrayList<>(); ArrayList ArrayListOfDiamond = new ArrayList<>();
public Hand() { ArrayListOfCard = new ArrayList<>(); }
public void fillHand(int handSize) { Deck deck = new Deck(); deck.Shuffle();
while (ArrayListOfCard.size() < handSize) {
for (int a = 0; a < handSize; a++) { Card newCard = deck.topCard();
if (newCard.getSuit() == 1) {
if (ArrayListOfSpade.isEmpty()) { ArrayListOfSpade.add(newCard); } else {
for (int i = 0; i < ArrayListOfSpade.size(); i++) { Card tempCard1 = ArrayListOfSpade.get(i);
if (newCard.getRank() < tempCard1.getRank()) { ArrayListOfSpade.add(i, newCard); }
} int max = ArrayListOfSpade.size(); Card maxRank = ArrayListOfSpade.get(max - 1);
if (newCard.getRank() > maxRank.getRank()) { ArrayListOfSpade.add(newCard); } } }
if (newCard.getSuit() == 2) {
if (ArrayListOfHeart.isEmpty()) { ArrayListOfHeart.add(newCard); } else {
for (int i = 0; i < ArrayListOfHeart.size(); i++) { Card tempCard = ArrayListOfHeart.get(i);
if (newCard.getRank() < tempCard.getRank()) { ArrayListOfHeart.add(i, newCard); }
} int max = ArrayListOfHeart.size(); Card maxRank = ArrayListOfHeart.get(max - 1);
if (newCard.getRank() > maxRank.getRank()) { ArrayListOfHeart.add(newCard); } } }
if (newCard.getSuit() == 3) {
if (ArrayListOfClub.isEmpty()) { ArrayListOfClub.add(newCard); } else {
for (int i = 0; i < ArrayListOfClub.size(); i++) { Card tempCard = ArrayListOfClub.get(i);
if (newCard.getRank() < tempCard.getRank()) { ArrayListOfClub.add(i, newCard); }
} int max = ArrayListOfClub.size(); Card maxRank = ArrayListOfClub.get(max - 1);
if (newCard.getRank() > maxRank.getRank()) { ArrayListOfClub.add(newCard); } } }
if (newCard.getSuit() == 4) {
if (ArrayListOfDiamond.isEmpty()) { ArrayListOfDiamond.add(newCard); } else {
for (int i = 0; i < ArrayListOfDiamond.size(); i++) { Card tempCard = ArrayListOfDiamond.get(i);
if (newCard.getRank() < tempCard.getRank()) { ArrayListOfDiamond.add(i, newCard); }
} int max = ArrayListOfDiamond.size(); Card maxRank = ArrayListOfDiamond.get(max - 1);
if (newCard.getRank() > maxRank.getRank()) { ArrayListOfDiamond.add(newCard); } } } }
ArrayListOfCard.addAll(ArrayListOfSpade); ArrayListOfCard.addAll(ArrayListOfHeart); ArrayListOfCard.addAll(ArrayListOfClub); ArrayListOfCard.addAll(ArrayListOfDiamond); } }
public String handToString() { String handToString = "";
for (int i = 0; i < ArrayListOfCard.size(); i++) { handToString = ArrayListOfCard.get(i).toString() + " "; }
return handToString; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
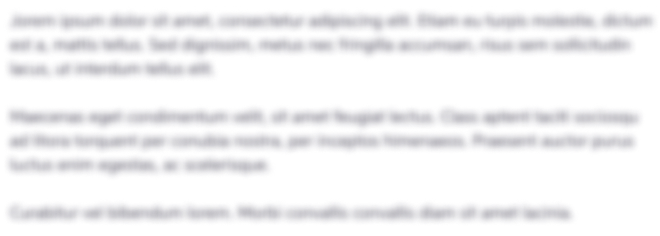
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started