Question
Below is the class that needs to be changed slightly. #pragma once #include // Declaration template class FixedVector { private: size_t size_; // number of
Below is the class that needs to be changed slightly.
#pragma once | |
#include | |
// Declaration | |
template | |
class FixedVector { | |
private: | |
size_t size_; // number of elements in the data structure | |
const size_t capacity_; // length of the array | |
T* array_; // pointer to dynamically allocated array | |
public: | |
// Constructors | |
FixedVector(size_t arraysize = 0); // Also serves as default constructor | |
FixedVector(const FixedVector& input ); // Copy constructor | |
~FixedVector(); | |
// Getters / Setters | |
T& at(size_t index); | |
T& operator[](size_t index); | |
void push_back(const T& value); | |
void set(size_t index, const T& value); | |
void erase(size_t index); | |
void insert(size_t beforeIndex, const T& value); | |
size_t size(); | |
bool empty(); | |
void clear(); | |
// Overloaded Operators | |
FixedVector& operator=(const FixedVector& rhs); //Copy assignment | |
}; | |
// Implementation | |
// Constructor with initial capacity argument | |
template | |
FixedVector | |
array_ = new T[capacity_]; | |
} | |
template | |
size_t FixedVector | |
return size_; | |
} | |
template | |
bool FixedVector | |
return (size_ == 0); | |
} | |
template | |
void FixedVector | |
size_ = 0; | |
} | |
// Getter | |
template | |
T& FixedVector | |
if (index >= size_) { | |
throw std::range_error( "index out of bounds" ); | |
} | |
return array_[index]; | |
} | |
// Getter | |
template | |
void FixedVector | |
insert( size_, value ); // delegate to insert() leveraging error checking | |
} | |
// Overloaded Array-Access Operator | |
template | |
T& FixedVector | |
return array_[index]; // Note: intentionally not checking array bounds | |
} | |
// Setter | |
template | |
void FixedVector | |
at( index ) = value; // delegate to at() leveraging error checking | |
} | |
// Removes element from position. Elements from higher positions are shifted back to fill gap. | |
// Vector size decrements | |
template | |
void FixedVector | |
if (index >= size_) { | |
throw std::range_error( "index out of bounds" ); | |
} | |
// move elements to close the gap from the left and working right | |
for (size_t j = index + 1; j < size_; j++) { // shift elements to the left | |
array_[j-1] = array_[j]; | |
} | |
size_--; | |
} | |
// Copies x to element at position. Items at that position and higher are shifted over to make room. Vector size increments. | |
template | |
void FixedVector | |
if( size_ >= capacity_) { | |
throw std::range_error("insufficient capacity to add another element"); | |
} | |
if( beforeIndex > size_ ) { | |
beforeIndex = size_; // insert at the back | |
} | |
// move elements to create space starting from the right and working left | |
for( size_t j = size_; j > beforeIndex; j-- ) { | |
array_[ j ] = array_[ j-1 ]; // shift elements to the right | |
} | |
array_[ beforeIndex ] = value; // put in empty slot | |
size_++; | |
} | |
// Copy Constructor | |
template | |
FixedVector | |
array_ = new T[capacity_]; | |
// Copy each element from the input vector to this vector | |
for (size_t i = 0; i < size_; i++) { | |
array_[i] = input.array_[i]; | |
} | |
} | |
// Overloaded Assignment Operator | |
template | |
FixedVector | |
if (this != &rhs) { | |
// Being fixed size, the already allocated array can be reused | |
// Capacity is not adjusted. If capacity_ < rhs.capacity, then some | |
// rhs elements may not be copied. | |
for (size_ = 0; size_ < rhs.size_ && size_ < capacity_ ; ++size_ ) { | |
array_[size_] = rhs.array_[size_]; | |
} | |
} | |
return *this; | |
} | |
// Destructor | |
template | |
FixedVector | |
delete[] array_; | |
} |
Working with char vector using Array
You want to use additional operations within the class, so the function member insert needs to be changed, as well as two new functions members, find and operator == need to be added to the class definition and implemented. Consider the new class definition below, and the new/modified functions being in bold.
Please Update the class by adding the 2 new functions, and implementing it.
size_t find(const T& value);
bool operator== (const FixedVector& rhs);
Please change the insert class
size_t insert(size_t beforeIndex, const T& value);
//Heres the main function to do the testing on.
#include #include "FixedVector.h"
using namespace std;
int main() { // testing the new implementation of a FixedVector
// declare & initialize a FixedVector of int with 10 elements FixedVector Array1(5);
// place 1,5,10 in the array cout << "FixedArray gets the elements 1, 5, 10" << endl; Array1.push_back(1); Array1.push_back(5); Array1.push_back(10);
// Try the find operation cout << "Value 5 is at index " << Array1.find(5) << endl;
// Try the insert operation cout << "Value 2 is inserted at index" << Array1.insert(1, 2) << endl;
// Try the == operator FixedVector Array2(5); Array2.push_back(1); Array2.push_back(5); Array2.push_back(10); if (Array1 == Array2) cout << "The two arrays are the same." << endl; else cout << "The two arrays are different." << endl;
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
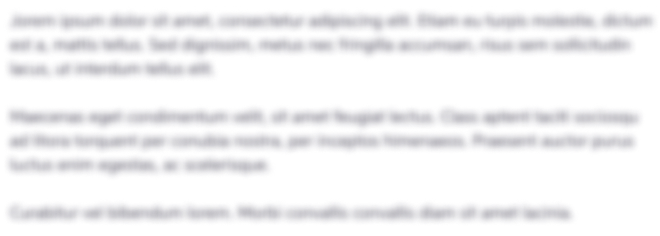
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started