Question
Below is the code that needs editing because there are a few errors. Please let me know how to fix the errors. Errors: Exception in
Below is the code that needs editing because there are a few errors. Please let me know how to fix the errors.
Errors:
Exception in thread "main" java.util.NoSuchElementException
at java.base/java.util.Scanner.throwFor(Scanner.java:937)
at java.base/java.util.Scanner.next(Scanner.java:1478)
at ShoppingCartManager.printMenu(ShoppingCartManager.java:40)
at ShoppingCartManager.main(ShoppingCartManager.java:20)
Shopping Cart improperly initialized with default constructor. getCustomerName() returns none getDate() returns February 1, 2016
error: method printMenu in class ShoppingCartManager cannot be applied to given types; ShoppingCartManager.printMenu(); ^ required: ShoppingCart,Scanner found: no arguments reason: actual and formal argument lists differ in length 1 error
error: cannot find symbol ShoppingCartManager.executeMenu(usrOption, myCart, scnr); ^ symbol: method executeMenu(char,ShoppingCart,Scanner) location: class ShoppingCartManager 1 error
Please help fix these errors and show updated code. (I need all 3 classes)
import java.util.Scanner; public class ShoppingCartManager { public static void main (String[]args) { Scanner scnr = new Scanner (System.in); String customerName; String todaysDate; System.out.print ("Enter Customer's Name: "); customerName = scnr.nextLine (); System.out.print ("Enter Today's Date: "); todaysDate = scnr.nextLine (); ShoppingCart userCart = new ShoppingCart (customerName, todaysDate); System.out.println (" Customer Name: " + userCart.getCustomerName ()); System.out.println ("Today's Date: " + userCart.getDate ()); printMenu (userCart, scnr); System.exit (0); } public static void printMenu (ShoppingCart shoppingCart, Scanner scnr) { char userChoice = ' '; boolean done = false; String clrScnr; String menu = (" MENU " + "a - Add item to cart " + "d - Remove item from cart " + "c - Change item quantity " + "i - Output items' descriptions " + "o - Output shopping cart " + "q - Quit "); System.out.print (menu); do { System.out.print (" Choose an option:"); userChoice = scnr.next ().charAt (0); switch (userChoice) { case ('q'): System.out.println (); done = true; break; case ('o'): System.out.println (" OUTPUT SHOPPING CART"); System.out.println (shoppingCart.getCustomerName () + "'s Shopping Cart - " + shoppingCart.getDate ()); if (shoppingCart.getNumItemsInCart () == 0) { System.out.println ("Number of Items: " + shoppingCart.getNumItemsInCart ()); System.out.print (" SHOPPING CART IS EMPTY"); } else { System.out.println ("Number of Items: " + shoppingCart.getNumItemsInCart ()); } shoppingCart.printTotal (); System.out.println (" Total: $" + shoppingCart.getCostOfCart ()); System.out.print (menu); break; case ('i'): System.out.println (" OUTPUT ITEMS' DESCRIPTIONS"); System.out.println (shoppingCart.getCustomerName () + "'s Shopping Cart - " + shoppingCart.getDate () + " "); shoppingCart.printDescriptions (); System.out.print (menu); break; case ('a'): clrScnr = scnr.nextLine (); ItemToPurchase newItem = new ItemToPurchase (); System.out.println (" ADD ITEM TO CART"); System.out.print ("Enter the item name:"); newItem.setName (scnr.nextLine ()); System.out.print (" Enter the item description:"); newItem.setDescription (scnr.nextLine ()); System.out.print (" Enter the item price:"); newItem.setPrice (scnr.nextInt ()); System.out.print (" Enter the item quantity:"); newItem.setQuantity (scnr.nextInt ()); shoppingCart.addItem (newItem); System.out.print (" " + menu); break; case ('d'): clrScnr = scnr.nextLine (); System.out.println (" REMOVE ITEM FROM CART"); System.out.print ("Enter name of item to remove:"); shoppingCart.removeItem (scnr.nextLine ()); System.out.print (" " + menu); break; case ('c'): clrScnr = scnr.nextLine (); System.out.println (" CHANGE ITEM QUANTITY"); ItemToPurchase changeItem = new ItemToPurchase (); System.out.print ("Enter the item name:"); changeItem.setName (scnr.nextLine ()); System.out.print (" Enter the new quantity:"); changeItem.setQuantity (scnr.nextInt ()); shoppingCart.modifyItem (changeItem); System.out.print (menu); break; default: break; } } while (!done); } }
import java.util.ArrayList; import java.util.Scanner; public class ShoppingCart { private String customerName; private String currentDate; private ArrayList < ItemToPurchase > cartItems = new ArrayList < ItemToPurchase > (); public ShoppingCart () { this.customerName = "none"; this.currentDate = "February 1, 2016"; } public ShoppingCart (String customerName, String currentDate) { this.customerName = customerName; this.currentDate = currentDate; } public void setCustomerName (String customerName) { this.customerName = customerName; } public void setDate (String currentDate) { this.currentDate = currentDate; } public String getCustomerName () { return customerName; } public String getDate () { return currentDate; } public void addItem (ItemToPurchase ItemToPurchase) { this.cartItems.add (cartItems.size (), ItemToPurchase); } public void removeItem (String ItemToRemove) { int count = 0; for (int i = 0; i < cartItems.size (); ++i) { if (cartItems.get (i).getName ().equalsIgnoreCase (ItemToRemove)) { cartItems.remove (i); ++count; } } if (count == 0) { System.out.print (" Item not found in cart. Nothing removed."); } } public void modifyItem (ItemToPurchase itemToPurchase) { int count = 0; for (int i = 0; i < cartItems.size (); ++i) { if (cartItems.get (i).getName ().equals (itemToPurchase.getName ())) { cartItems.get (i).setQuantity (itemToPurchase.getQuantity ()); ++count; } } if (count == 0) { System.out.println (" Item not found in cart. Nothing modified."); } } public int getNumItemsInCart () { int numItems = 0; for (int i = 0; i < cartItems.size (); ++i) { numItems = numItems + cartItems.get (i).getQuantity (); } return numItems; } public int getCostOfCart () { int totalCost = 0; for (int i = 0; i < cartItems.size (); ++i) { totalCost = totalCost + (cartItems.get (i).getPrice () * cartItems.get (i).getQuantity ());; } return totalCost; } public void printTotal () { for (int i = 0; i < cartItems.size (); ++i) { System.out.print (cartItems.get (i).printItemCost ()); } System.out.println (); } public void printDescriptions () { System.out.println ("Item Descriptions"); for (int i = 0; i < cartItems.size (); ++i) { System.out.println (cartItems.get (i).printItemDescription ()); } } }
public class ItemToPurchase { private String itemName; private int itemPrice; private int itemQuantity; private String itemDescription; public ItemToPurchase () { this.itemName = "none"; this.itemPrice = 0; this.itemQuantity = 0; this.itemDescription = "none"; } public ItemToPurchase (String itemName, String itemDescription, int itemPrice, int itemQuantity) { this.itemName = itemName; this.itemPrice = itemPrice; this.itemQuantity = itemQuantity; this.itemDescription = itemDescription; } public void setName (String itemName) { this.itemName = itemName; } public void setPrice (int itemPrice) { this.itemPrice = itemPrice; } public void setQuantity (int itemQuantity) { this.itemQuantity = itemQuantity; } public void setDescription (String itemDescription) { this.itemDescription = itemDescription; } public String getName () { return itemName; } public int getPrice () { return itemPrice; } public int getQuantity () { return itemQuantity; } public String getDescription () { return itemDescription; } public String printItemCost () { String cost = (" " + itemName + " " + itemQuantity + " @ $" + itemPrice + " = $" + (itemQuantity * itemPrice)); return cost; } public String printItemDescription () { String description = (itemName + ": " + itemDescription); return description; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
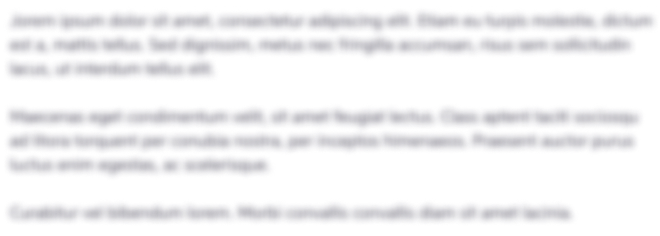
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started