Question
Below is the .h, .cpp, and main .cpp files - Code looks lengthy but its a few simple questions. #include #include #include #include #include #include
Below is the .h, .cpp, and main .cpp files - Code looks lengthy but its a few simple questions.
#include
#include
#include
#include
#include
#include
using namespace std;
//The following two lines come from HALglobals.h
const int MAX_COMMAND_LINE_ARGUMENTS = 8;
const int SLEEP_DELAY = 100000;
int GetCommand (string tokens []);
int TokenizeCommandLine (string tokens [], string commandLine);
bool CheckForCommand ();
int ProcessCommand (string tokens [], int tokenCount);
static volatile sig_atomic_t cullProcess = 0;
#include "HALmod.h"
int main()
{
string tokens [MAX_COMMAND_LINE_ARGUMENTS];
int tokenCount;
do
{
tokenCount = GetCommand (tokens);
} while (ProcessCommand (tokens, tokenCount));
return 0;
}
#include "HALmod.h"
int GetCommand (string tokens [])
{
string commandLine;
bool commandEntered;
int tokenCount;
do
{
cout << "HALshell> ";
while (1)
{
getline (cin, commandLine);
commandEntered = CheckForCommand ();
if (commandEntered)
{
break;
}
}
} while (commandLine.length () == 0);
tokenCount = TokenizeCommandLine (tokens, commandLine);
return tokenCount;
}
int TokenizeCommandLine (string tokens [], string commandLine)
{
char *token [MAX_COMMAND_LINE_ARGUMENTS];
char *workCommandLine = new char [commandLine.length () + 1];
int i;
int tokenCount;
for (i = 0; i < MAX_COMMAND_LINE_ARGUMENTS; i ++)
{
tokens [i] = "";
}
strcpy (workCommandLine, commandLine.c_str ());
i = 0;
if ((token [i] = strtok (workCommandLine, " ")) != NULL)
{
i ++;
while ((token [i] = strtok (NULL, " ")) != NULL)
{
i ++;
}
}
tokenCount = i;
for (i = 0; i < tokenCount; i ++)
{
tokens [i] = token [i];
}
delete [] workCommandLine;
return tokenCount;
}
//Do not touch the below function
bool CheckForCommand ()
{
if (cullProcess)
{
cullProcess = 0;
cin.clear ();
cout << "\b\b \b\b";
return false;
}
return true;
}
//This is a very paired down version of Dr. Hilderman's function
int ProcessCommand (string tokens [], int tokenCount)
{
if (tokens [0] == "shutdown" || tokens [0] == "restart")
{
if (tokenCount > 1)
{
cout << "HALshell: " << tokens [0] << " does not require any arguments" << endl;
return 1;
}
cout << endl;
cout << "HALshell: terminating ..." << endl;
return 0;
}
else
return 1;
}
Question- We want to be able to turn the tokens array into an array of c strings (In other words, in the format of char **). Build the following functions and call them from main:
- char ** ConvertToC (string tokens [], int tokenCount);
- This converts the tokens into a c version of an array of words and returns the pointer to that array
- void CleanUpCArray (char ** cTokens, int tokenCount);
- This cycles through the c string version of the array and frees up any memory that has been allocated
- void PrintReverse (char ** cTokens, int tokenCount);
- This cycles in reverse through the c string version of the words and prints each word
A sample run might look something like the following
a049840[8]%./demo HALshell> This is a test The words in reverse are: test a is This HALshell> to see what happens The words in reverse are: happens what see to HALshell> It is a success The words in reverse are: success a is It HALshell> lo The words in reverse are: lo HALshell: terminating ...
When you run your code through valgrind using: valgrind --leak-check=yes ./demo A message like the following should be displayed:
==23683== ==23683== HEAP SUMMARY: ==23683== in use at exit: 0 bytes in 0 blocks ==23683== total heap usage: 46 allocs, 46 frees, 992 bytes allocated ==23683== ==23683== All heap blocks were freed -- no leaks are possible ==23683== ==23683== For counts of detected and suppressed errors, rerun with: -v ==23683== ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
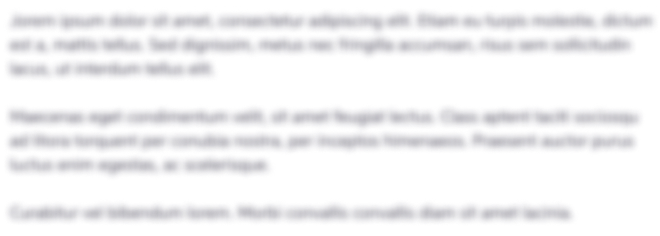
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started