Question
BinaryTree1 public class BinaryTree1 implements Serializable { /* */ /** Class to encapsulate a tree node. */ protected static class Node implements Serializable { //
BinaryTree1
public class BinaryTree1
/*
/** The information stored in this node. */ public E data; /** Reference to the left child. */ public Node
// Constructors /** * Construct a node with given data and no children. * @param data The data to store in this node */ public Node(E data) { this.data = data; left = null; right = null; }
// Methods /** * Returns a string representation of the node. * @return A string representation of the data fields */ @Override public String toString() { return data.toString(); } } /*
/** Construct an empty BinaryTree1 */ public BinaryTree1() { root = null; }
/** * Construct a BinaryTree1 with a specified root. * Should only be used by subclasses. * @param root The node that is the root of the tree. */ protected BinaryTree1(Node
/** * Constructs a new binary tree with data in its root,leftTree * as its left subtree and rightTree as its right subtree. */ public BinaryTree1(E data, BinaryTree1
/** * Return the left subtree. * @return The left subtree or null if either the root or * the left subtree is null */ public BinaryTree1
/** * Return the right sub-tree * @return the right sub-tree or * null if either the root or the * right subtree is null. */ public BinaryTree1
/** * Return the data field of the root * @return the data field of the root * or null if the root is null */ public E getData() { if (root != null) { return root.data; } else { return null; } }
/** * Determine whether this tree is a leaf. * @return true if the root has no children */ public boolean isLeaf() { return (root == null || (root.left == null && root.right == null)); }
@Override public String toString() { StringBuilder sb = new StringBuilder(); preOrderTraverse(root, 1, sb); return sb.toString(); }
/** * Perform a preorder traversal. * @param node The local root * @param depth The depth * @param sb The string buffer to save the output */ private void preOrderTraverse(Node /*
BinarySearchTree
public class BinarySearchTree
/** Return value from the public add method. */ protected boolean addReturn; /** Return value from the public delete method. */ protected E deleteReturn;
//Methods /*
/** * Recursive find method. * @param localRoot The local subtrees root * @param target The object being sought * @return The object, if found, otherwise null */ private E find(Node
// Compare the target with the data field at the root. int compResult = target.compareTo(localRoot.data); if (compResult == 0) { return localRoot.data; } else if (compResult */
/*
/** * Recursive add method. * @post The data field addReturn is set true if the item is added to * the tree, false if the item is already in the tree. * @param localRoot The local root of the subtree * @param item The object to be inserted * @return The new local root that now contains the * inserted item */ private Node
/*
/** * Recursive delete method. * @post The item is not in the tree; * deleteReturn is equal to the deleted item * as it was stored in the tree or null * if the item was not found. * @param localRoot The root of the current subtree * @param item The item to be deleted * @return The modified local root that does not contain * the item */ private Node
// Search for item to delete. int compResult = item.compareTo(localRoot.data); if (compResult 0) { // item is larger than localRoot.data. localRoot.right = delete(localRoot.right, item); return localRoot; } else { // item is at local root. deleteReturn = localRoot.data; if (localRoot.left == null) { // If there is no left child, return right child // which can also be null. return localRoot.right; } else if (localRoot.right == null) { // If there is no right child, return left child. return localRoot.left; } else { // Node being deleted has 2 children, replace the data // with inorder predecessor. if (localRoot.left.right == null) { // The left child has no right child. // Replace the data with the data in the // left child. localRoot.data = localRoot.left.data; // Replace the left child with its left child. localRoot.left = localRoot.left.left; return localRoot; } else { // Search for the inorder predecessor (ip) and // replace deleted node's data with ip. localRoot.data = findLargestChild(localRoot.left); return localRoot; } } } } /*
/*
BinarySearchTreeTest
public class BinarySearchTreeTest { /** * @param args the command line arguments */ public static void main(String[] args) { //create a binary search tree BinarySearchTree
1. Objectives This assignment will help you to: Learn how to program using data structure Binary Search Tree Understand how Binary Search Tree works Enhance your skills in programming using linked lists 2. Overview You are given the classes BinaryTree1.java, BinarySearchTree.java, and BinarySearchTreeTest.java. Add the following member methods to the class BinarySearchTree: depthOfMinValueRecursive): returns the depth of the node in the calling BST which contains the minimum value. Use recursion. depthOfMinValuelterative(): returns the depth of the node in the calling BST which contains the minimum value. Do not use recursion. equalStruct(BinarySearchTree bst2): Check if the calling BST and the bst2 have the same structure. Test your methods in another class BinarySearchTreeTest that uses the class BinarySearchTree and completes a number of operations on a single BinarySearchTree objects and multiple BinarySearchTree objects. 3. Implementation Requirements You must use the classes provided to you. This requirement means that you cannot use Java API class for Binary Search Tree. . You must implement the required methods as stated in the BinarySearchTree class. Major Steps Understand the related classes I gave you in previous lectures (Lec#17, Lec#18). i. BinaryTree1.java, BinarySearchTree.java, SearchTree.java, ReadBinaryTree1.java, BinarySearchTreeTest.java Revise the class BinarySearchTree. Add necessary methods. At one method at a time. Test it in BinarySearchTreeTest
Step by Step Solution
There are 3 Steps involved in it
Step: 1
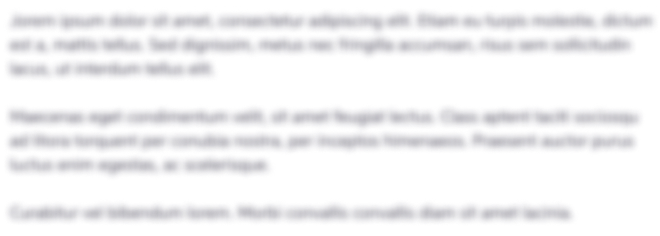
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started