Question
BinaryTree.java: public class BinaryTree > { private Node root; public BinaryTree (){ root = null; } private BinaryTree (Node r){ root = r; // null
BinaryTree.java:
public class BinaryTree
public BinaryTree (){ root = null; }
private BinaryTree (Node
public BinaryTree (E data, BinaryTree
public String toString(){ String s = ""; return rec_toString(root, 1, s); }
private String rec_toString(Node
private static class Node
private Node(E item){ data = item; left = null; right = null; }
private Node(E item, Node
} //end of private class }
BinaryTreeTest.java:
import javax.swing.JOptionPane;
import java.util.*;
import java.io.*;
public class BinaryTreeTest {
public static void main(String args[]){
BinaryTree
String filename =
JOptionPane.showInputDialog("Enter the file name: ");
File inputFile = new File (filename);
try {
Scanner input = new Scanner(inputFile);
while(input.hasNext()){
Integer val = input.nextInt();
a.add(val);
}
input.close();
}
catch (FileNotFoundException e)
{
System.out.println("file reading fails.");
}
System.out.println(a);
Scanner kb = new Scanner(System.in);
System.out.println("1: select the deletion");
System.out.println("2: select the search");
System.out.println("3: find_min");
System.out.println("4: find_max");
System.out.println("5: insert another record");
System.out.println("6: display the tree in infix travel");
System.out.println("7: display the tree in postfix travel");
System.out.println("8: display the tree in prefix travel");
System.out.println("9: display the tree with toString");
System.out.println("0/others: QUiT");
int ans = kb.nextInt();
while(1
String str;
switch(ans){
case 1:
str =
JOptionPane.showInputDialog("Key in the item (i.e., content of tree node) to delete:");
a.delete(Integer.parseInt(str));
break;
case 2:
str =
JOptionPane.showInputDialog("Key in the item (i.e., content of tree node) to search:");
System.out.println("Search result: "+a.search(Integer.parseInt(str)));
break;
case 3:
System.out.println("Minimum: "+a.find_min());
break;
case 4:
System.out.println("Maximum: "+a.find_max());
break;
case 5:
str =
JOptionPane.showInputDialog("Key in the item (i.e., content of tree node) to insert");
a.add(Integer.parseInt(str));
break;
case 6:
System.out.println("Display the tree in the infix travel");
System.out.println(a.infixString());
break;
case 7:
System.out.println("Display the tree in the postfix travel");
System.out.println(a.postfixString());
break;
case 8:
System.out.println("Display the tree in the prefix travel");
System.out.println(a.prefixString());
break;
case 9:
System.out.println("The tree display with reserved spaces");
System.out.println(a);
break;
}
System.out.println("1: select the deletion");
System.out.println("2: select the search");
System.out.println("3: find_min");
System.out.println("4: find_max");
System.out.println("5: insert another record");
System.out.println("6: display the tree in infix travel");
System.out.println("7: display the tree in postfix travel");
System.out.println("8: display the tree in prefix travel");
System.out.println("9: display the tree with toString");
System.out.println("0/others: QUiT");
ans = kb.nextInt();
}
}
}
DictionaryTreeTest.java:
import javax.swing.JOptionPane;
import java.util.*;
import java.io.*;
public class DictionaryTreeTest {
public static void main(String args[]){
BinaryTree
String filename =
JOptionPane.showInputDialog("Enter the file name: ");
File inputFile = new File (filename);
try {
Scanner input = new Scanner(inputFile);
while(input.hasNext()){
String val = input.nextLine();
a.add(val);
}
input.close();
}
catch (FileNotFoundException e)
{
System.out.println("file reading fails.");
}
System.out.println(a);
Scanner kb = new Scanner(System.in);
System.out.println("1: select the deletion");
System.out.println("2: select the search");
System.out.println("3: find_min");
System.out.println("4: find_max");
System.out.println("5: insert another record");
System.out.println("6: display the tree in infix travel");
System.out.println("7: display the tree in postfix travel");
System.out.println("8: display the tree in prefix travel");
System.out.println("9: display the tree with toString");
System.out.println("0/others: QUiT");
int ans = kb.nextInt();
while(1
String str;
switch(ans){
case 1:
str =
JOptionPane.showInputDialog("Key in the item (i.e., content of tree node) to delete:");
a.delete(str);
break;
case 2:
str =
JOptionPane.showInputDialog("Key in the item (i.e., content of tree node) to search:");
System.out.println("Search result: "+a.search(str));
break;
case 3:
System.out.println("Minimum: "+a.find_min());
break;
case 4:
System.out.println("Maximum: "+a.find_max());
break;
case 5:
str =
JOptionPane.showInputDialog("Key in the item (i.e., content of tree node) to insert");
a.add(str);
break;
case 6:
System.out.println("Display the tree in the infix travel");
System.out.println(a.infixString());
break;
case 7:
System.out.println("Display the tree in the postfix travel");
System.out.println(a.postfixString());
break;
case 8:
System.out.println("Display the tree in the prefix travel");
System.out.println(a.prefixString());
break;
case 9:
System.out.println("The tree display with reserved spaces");
System.out.println(a);
break;
}
System.out.println("1: select the deletion");
System.out.println("2: select the search");
System.out.println("3: find_min");
System.out.println("4: find_max");
System.out.println("5: insert another record");
System.out.println("6: display the tree in infix travel");
System.out.println("7: display the tree in postfix travel");
System.out.println("8: display the tree in prefix travel");
System.out.println("9: display the tree with toString");
System.out.println("0/others: QUiT");
ans = kb.nextInt();
}
}
}
2. Implement the class BinarvTree to support the program BinaryTreeTest.java. You can test the program with the input files BT1 and BT2 from the above zip file jGRASP exec: java Bin st null null null null null null null 1: select the deletion 2: select the search 3: find min 4: find max 5: insert another record 6: display the tree in infix travel 7: display the tree in postfix travel 8: display the tree in prefix travel 9:display the tree with toString 0/Others : QUT Display the tree in the infix travel 1: select the deletion 2: select the search 3: find min 4: find max 5: insert another record 6: display the tree in infix travel 7: display the tree in postfix travel 8: display the tree in prefix travel 9: display the tree with tostring 0/Others: QUT The same binary tree class should also support the DictionaryTree.java with the input file BTdictionaryStep by Step Solution
There are 3 Steps involved in it
Step: 1
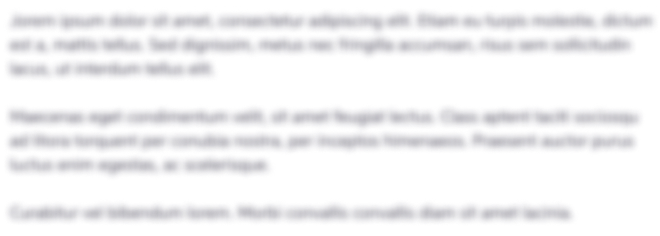
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started