Question
/* * bitAnd - x&y using only ~ and | * Example: bitAnd(6, 5) = 4 * Legal ops: ~ | * Max ops: 8
/* * bitAnd - x&y using only ~ and | * Example: bitAnd(6, 5) = 4 * Legal ops: ~ | * Max ops: 8 * Rating: 1 */ int bitAnd(int x, int y) { return ~(~x | ~y); } /* * evenBits - return word with all even-numbered bits set to 1 * Legal ops: ! ~ & ^ | + << >> * Max ops: 8 * Rating: 1 */ int evenBits(void) { int result = 0x55; result = result | result << 8; return result | result << 16; } /* * isTmin - returns 1 if x is the minimum two's complement number * and 0 otherwise * Legal ops: ! ~ & ^ | + * Max ops: 10 * Rating: 1 */ int isTmin(int x) { return !((x^(~x + 1)) + !x); } /* * allEvenBits - return 1 if all even-numbered bits in word set to 1 * Examples allEvenBits(0xFFFFFFFE) = 0, allEvenBits(0x55555555) = 1 * Legal ops: ! ~ & ^ | + << >> * Max ops: 12 * Rating: 2 */ int allEvenBits(int x) { int Bits; int Odd = (0xAA << 8) | 0xAA; Odd = Odd | (Odd << 16); /* this is for all odd bits to be set to 1 */ Bits = Odd | x; Bits = ~Bits; /* all 0's if x had each even bit at 1 */ return !Bits; }
explain what each code does, I have a general understanding but want to know more of how each works
Step by Step Solution
There are 3 Steps involved in it
Step: 1
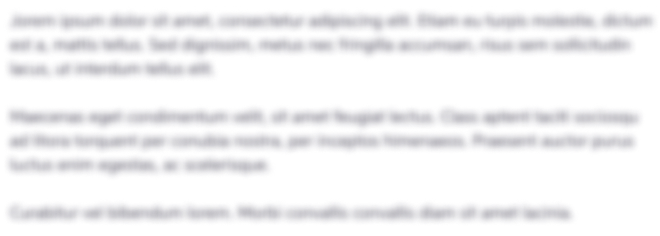
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started